How to Add Form Section in iOS SwiftUI
In SwiftUI, Forms play a vital role in collecting and organizing user input. Often, these forms require different sections to separate related elements or provide specific functionalities. In this blog post, we’ll explore SwiftUI’s Form Sections, how to use them, and the various ways they can be customized.
Create Basic Form Sections: Example
Here’s a simple example to get started with:
import SwiftUI
struct ContentView: View {
@State private var username = ""
@State private var email = ""
var body: some View {
Form {
Section(header: Text("User Information")) {
TextField("Username", text: $username)
TextField("Email", text: $email)
}
Section(header: Text("Preferences")) {
Toggle("Enable Notifications", isOn: .constant(true))
Picker("Theme", selection: .constant(1)) {
Text("Light").tag(1)
Text("Dark").tag(2)
}
}
}
}
}
Key Components:
Section Header:
You can provide a header to each section using header
parameter, helping the user to understand the content of that particular section.
Content Inside Sections:
You can place a variety of SwiftUI views inside a section, including TextField
, Toggle
, Picker
, and others.
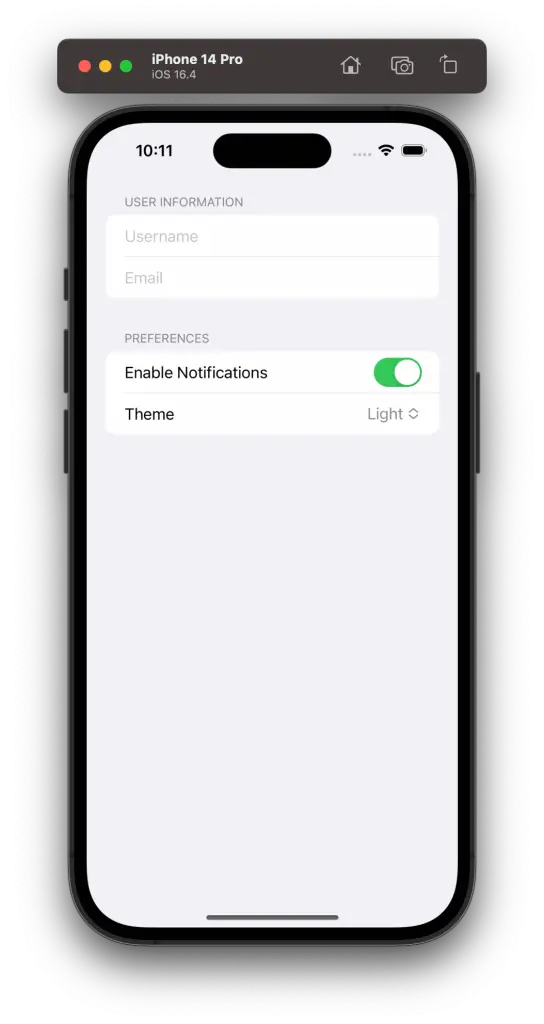
Customize Form Sections
Add Footers:
You can add footers to provide additional information or guidance related to the section:
import SwiftUI
struct ContentView: View {
@State private var username = ""
@State private var email = ""
var body: some View {
Form {
Section(header: Text("User Information"), footer: Text("Please provide accurate details")) {
TextField("Username", text: $username)
TextField("Email", text: $email)
}
}
}
}
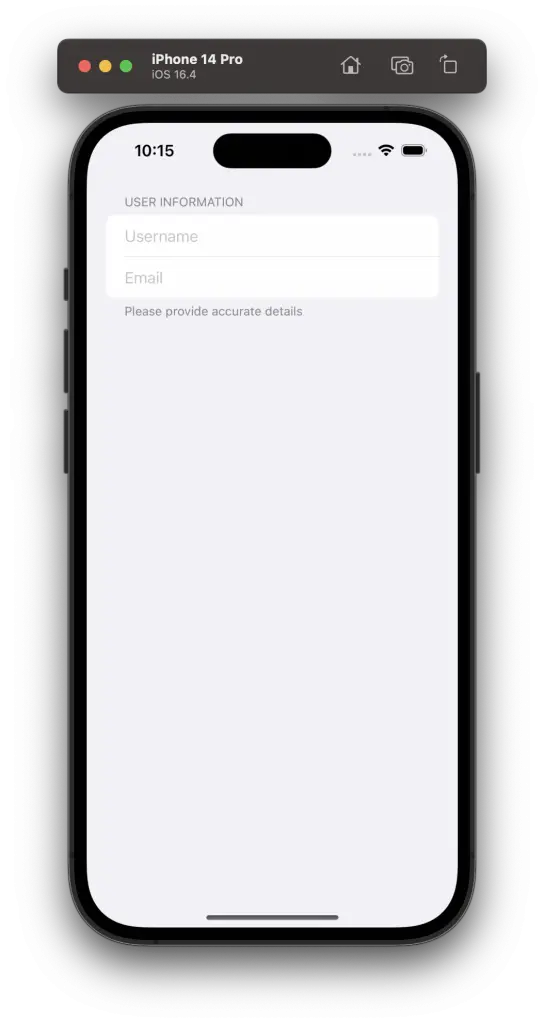
Style Headers and Footers:
You can style headers and footers by applying various modifiers like .font()
, .foregroundColor()
, etc.
Accessibility and Compatibility
- Accessibility: Always consider providing descriptive headers and footers to improve accessibility.
- Compatibility: Form Sections are supported in iOS 13 and later versions.
Form Sections in SwiftUI are a powerful tool to create organized, easy-to-follow user interfaces. Through sections, you can categorize related controls and inputs, enhancing the user experience and facilitating smoother interactions.
With ample customization options, you can create sections that align perfectly with your app’s design and functionality.