How to Capitalize First Letter in iOS SwiftUI TextField
Capitalizing the first letter in a text field is a common requirement in many applications, such as when entering names or starting a new sentence. This standard text formatting helps in providing a consistent and professional user experience.
In SwiftUI, you can control the capitalization behavior of a TextField using the autocapitalization
modifier. This blog post will explain how to capitalize the first letter in a SwiftUI TextField.
Capitalize the First Letter
Example Code
Here’s a basic example to demonstrate how to capitalize the first letter in a TextField:
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter text", text: $text)
.autocapitalization(.sentences)
.padding()
}
}
Key Components Explained
1. @State
Property Wrapper
The @State
property wrapper declares a private variable called text
to store the user’s input and allows the view to update when the text changes.
2. TextField
The TextField is created with a placeholder "Enter text"
and is bound to the text
state variable.
3. autocapitalization
Modifier
The autocapitalization
modifier controls the capitalization behavior of the text field. By setting it to .sentences
, the first letter of each sentence will be capitalized.
4. padding
Modifier
Padding is added to provide space around the TextField, making it visually appealing.
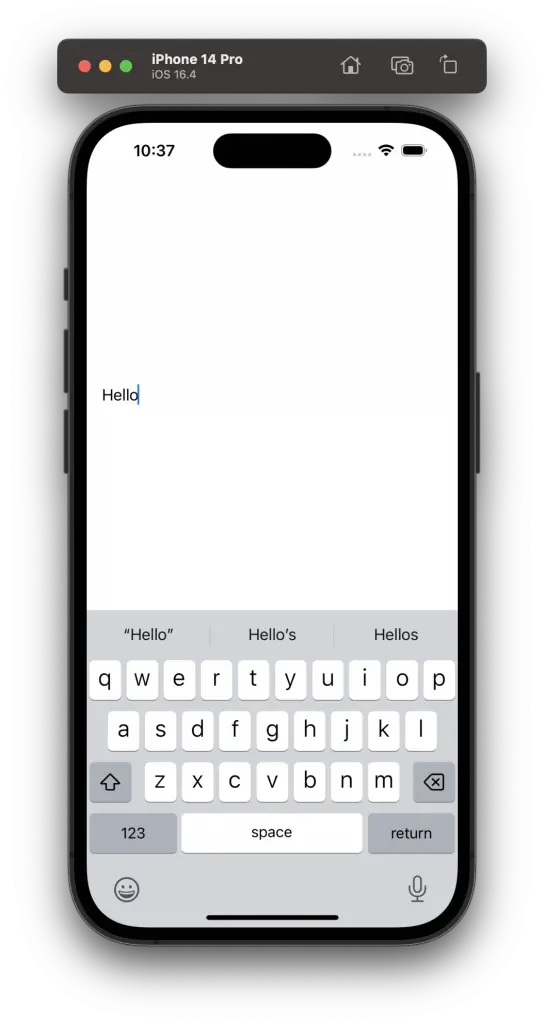
Other Capitalization Options
Besides .sentences
, SwiftUI provides other options for the autocapitalization
modifier:
.none
: No automatic capitalization..words
: Capitalizes the first letter of each word..allCharacters
: Capitalizes all characters.
You can experiment with these options to find the one that suits your needs.
Capitalizing the first letter in a TextField is a subtle but crucial detail that can enhance your app’s usability and professionalism.
SwiftUI’s autocapitalization
modifier provides a straightforward and efficient way to control the capitalization behavior of your text fields. Whether you need to capitalize the first letter of each sentence, word, or the entire text, SwiftUI has you covered.