How to Customize Font Color in iOS SwiftUI TextField
Designing an engaging user interface often requires customization of various elements, including fonts and colors. In SwiftUI, the TextField component is a common place for this customization, as it’s a key point of user interaction.
This post will explore how to change the font color of text within a SwiftUI TextField.
Change Font and Color in SwiftUI TextField
SwiftUI provides straightforward ways to modify the font and color of a TextField. Below, you’ll find an example that shows how to apply these changes:
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter text here", text: $text)
.font(.system(size: 20, weight: .bold, design: .rounded))
.foregroundColor(.blue)
.padding()
}
}
Font Customization
In the example, the font
modifier is used to customize the font of the TextField:
- Size: The font size is set to 20.
- Weight: The font weight is set to bold.
- Design: The font design is set to rounded.
Color Customization
The foregroundColor
modifier is applied to change the text color to blue.
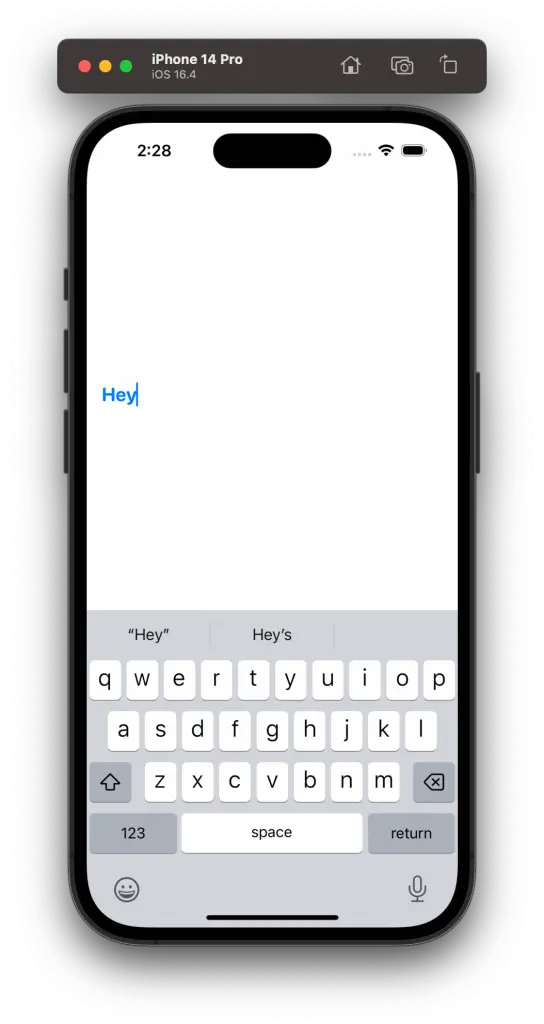
Step-by-Step Explanation
- State Property: We’re using the
@State
property to bind the text in the TextField, allowing the text to be stored and updated as the user types. - TextField: The placeholder text
"Enter text here"
is shown when the TextField is empty. It’s bound to thetext
state variable, so it updates as the user types. - Font Modifier: This modifier is used to set the font size, weight, and design.
- ForegroundColor Modifier: This sets the color of the text. You can choose from predefined colors like
.blue
, or define custom colors usingColor(red:green:blue:)
. - Padding Modifier: Padding is added around the TextField to give it some space within the layout.
Additional Customizations
You can further enhance the appearance by adding more modifiers. For instance, to set a background color, you can use the background
modifier:
.background(Color.yellow)
SwiftUI makes it effortless to customize the appearance of TextField, including changes to the font and color. With just a few lines of code, you can create a TextField that matches the design and theme of your app.
Remember that an effective UI is not just about looks; it should also enhance the usability and accessibility of your application. Choose your font sizes, weights, and colors wisely to make sure they contribute positively to the overall user experience.