How to Present Sheet from List in iOS SwiftUI
In SwiftUI, sheets are a popular way to present secondary views or modals. Often, you’ll want to present a sheet based on a user’s interaction with a list. In this blog post, we’ll explore how to present a sheet from a list in SwiftUI, complete with passing data between the list and the sheet.
The Scenario: Presenting Details
Imagine you have a list of items, and you want to show more details about an item when it’s tapped. You can use a sheet to present this detail view.
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
@State private var selectedItem: Int? = nil
var body: some View {
List(1...100, id: \.self) { index in
Text("Item \(index)")
.onTapGesture {
selectedItem = index
showModal = true
}
}
.sheet(isPresented: $showModal) {
DetailView(selectedItem: $selectedItem, showModal: $showModal)
}
}
}
struct DetailView: View {
@Binding var selectedItem: Int?
@Binding var showModal: Bool
var body: some View {
VStack {
if let item = selectedItem {
Text("You selected item \(item)")
}
Button("Dismiss") {
showModal = false
}
}
}
}
Code Explanation
@State private var showModal = false
: This state variable controls whether the sheet is presented or not.@State private var selectedItem: Int? = nil
: This state variable holds the index of the selected item..onTapGesture
: We use this gesture recognizer to updateselectedItem
and setshowModal
totrue
, which triggers the sheet to appear.DetailView(selectedItem: $selectedItem, showModal: $showModal)
: We pass theselectedItem
andshowModal
as bindings to theDetailView
, allowing us to read and modify them inside the sheet.
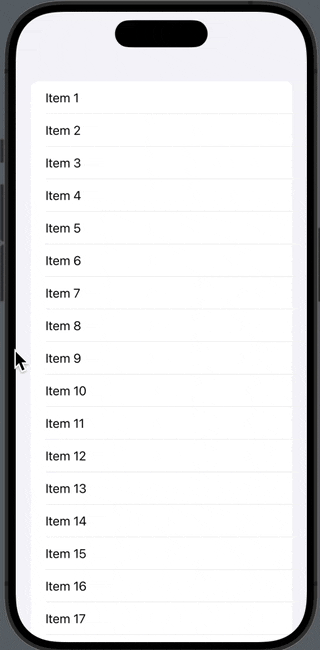
Why Use This Approach?
Here are some reasons why you might want to use this approach:
- User Experience: It provides a seamless way to show more details about an item without navigating away from the list.
- Data Passing: It allows you to pass data between the list and the sheet easily.
- Code Reusability: The
DetailView
can be reused in other parts of your app, as it’s a separate SwiftUI view.
Presenting a sheet from a list in SwiftUI is a common but crucial task, especially when you need to pass data between the list and the sheet. By using state variables and bindings, you can easily control the sheet’s presentation and pass the selected item’s details.