How to Create Password TextField in iOS SwiftUI
Security is a fundamental aspect of modern applications, and protecting user credentials is paramount. SwiftUI provides a way to create password text fields that ensure the privacy of user input.
This blog post will guide you through creating a secure password text field in SwiftUI.
Password TextField in SwiftUI
In SwiftUI, the SecureField
is specifically designed to handle password inputs. When a user types into a SecureField
, the characters are obscured, typically with dots or asterisks, to protect the information from prying eyes.
Here’s an example of how you can create a SecureField
:
struct ContentView: View {
@State private var password = ""
var body: some View {
SecureField("Enter password", text: $password)
.padding()
}
}
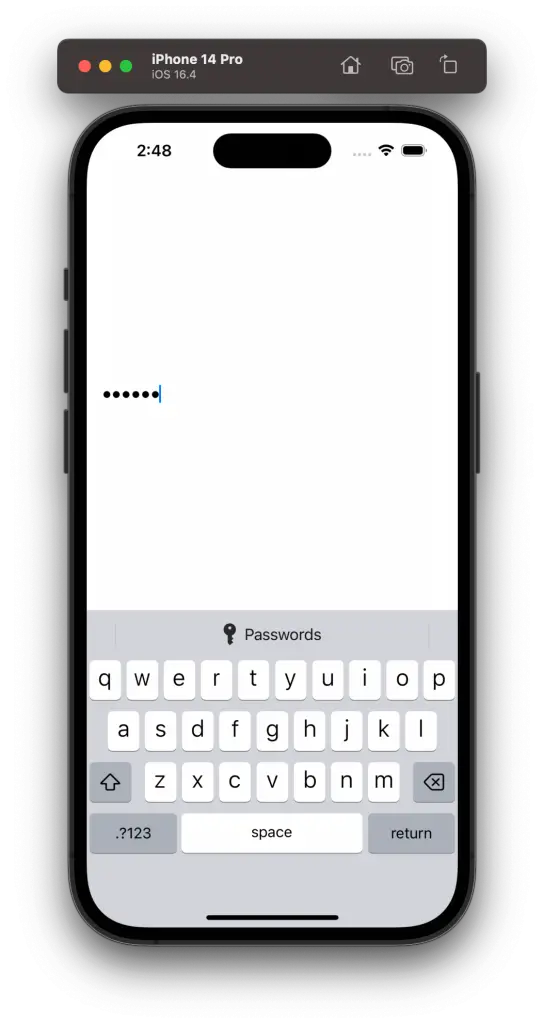
Code Explanation
@State
Property Wrapper
The @State
property wrapper is used to declare a private variable called password
. It stores the user’s password and triggers the view to update when the password changes.
SecureField
The SecureField
is created with a placeholder "Enter password"
and is bound to the password
state variable. The user’s input is automatically obscured.
padding
Modifier
Padding is added to provide space around the SecureField, enhancing its appearance.
Handling passwords securely is a critical part of app development, and SwiftUI’s SecureField
makes it simple to create an input field designed explicitly for this purpose.
By obscuring the user’s input and allowing for further customization, you can provide a secure and user-friendly way for users to enter their passwords in your app.