How to Create Button Press Effect in iOS SwiftUI
Interactivity is key in mobile applications. One area where this comes into play is button design. In SwiftUI, we can employ custom button styles to create interactive, responsive buttons. This article will walk you through creating a SwiftUI button press effect using custom button styles, complete with scale and opacity animations.
SwiftUI Custom Button Styles: A Brief Overview
In SwiftUI, custom button styles provide an opportunity to design unique, reusable button appearances. This feature greatly enhances the interactive elements of your app and allows for an exceptional level of customization.
Button Press Effects with Custom Button Styles
Laying the Foundation
Before we jump into the press effect, let’s understand how to create a basic button with a custom style. We define a struct that conforms to the ButtonStyle
protocol, which uses the makeBody
method to style the button:
struct BasicButtonStyle: ButtonStyle {
func makeBody(configuration: Configuration) -> some View {
configuration.label
.padding()
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(10)
}
}
Button(action: {
// What to perform
}) {
Text("Press Me!")
}.buttonStyle(BasicButtonStyle())
Inject a Press Effect
With the basic setup in place, it’s time to add the press effect. We’ll create a button that changes its scale and opacity when pressed, providing an interactive feedback:
struct ContentView: View {
@State private var isPressed = false
var body: some View {
Button(action: {
// What to perform
}) {
Text("Press Me!")
}.buttonStyle(PressEffectButtonStyle())
}
}
struct PressEffectButtonStyle: ButtonStyle {
func makeBody(configuration: Configuration) -> some View {
configuration.label
.padding()
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(10)
.scaleEffect(configuration.isPressed ? 0.9 : 1.0)
.opacity(configuration.isPressed ? 0.6 : 1.0)
.animation(.easeInOut, value: configuration.isPressed)
}
}
In this setup, configuration.isPressed
informs us if the button is currently being pressed. If it is, the button’s scale and opacity change, giving a subtle yet noticeable feedback to the user. The changes animate smoothly, creating a visually appealing interaction.
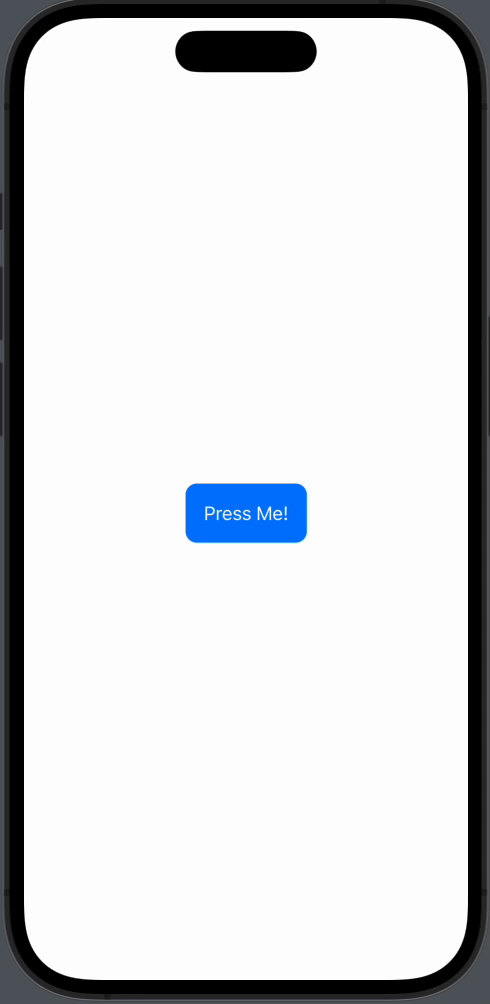
Creating a button press effect using custom button styles in SwiftUI is an effective way to enhance your app’s user experience. It offers a high degree of customization and can make your app stand out. It’s time to let your creativity loose and experiment with different styles and animations!