How to Add Picker with Segmented Style in iOS SwiftUI
SwiftUI offers a variety of ways to implement pickers in your apps. One of the most visually appealing and user-friendly styles is the segmented picker. In this blog post, we’ll explore how to implement a segmented picker using SwiftUI and discuss its advantages.
What is a Segmented Picker?
A segmented picker is a horizontal control made of multiple segments. Each segment is a discrete option. Users can tap on a segment to make a selection. This style is particularly useful when you have a limited set of options that can fit horizontally on the screen.
Advantages of Segmented Picker
- Quick Selection: Allows for fast and easy option selection.
- Clear Visibility: All options are visible at once, making it easier for users to make a choice.
How to Implement Segmented Picker in SwiftUI
Create a State Variable
First, you’ll need a state variable to keep track of the selected segment.
@State private var selectedSegment = 0
Add the Picker
Next, add the Picker element to your SwiftUI view. Make sure the pickerStyle is segmented.
Picker("Options", selection: $selectedSegment) {
// Picker segments go here
}
.pickerStyle(.segmented)
Add Segments
Now, add the segments for the Picker.
Text("Segment 1").tag(0)
Text("Segment 2").tag(1)
Text("Segment 3").tag(2)
Complete Example
Here’s how everything comes together:
import SwiftUI
struct ContentView: View {
@State private var selectedSegment = 0
var body: some View {
Picker("Options", selection: $selectedSegment) {
Text("Segment 1").tag(0)
Text("Segment 2").tag(1)
Text("Segment 3").tag(2)
}
.pickerStyle(.segmented)
}
}
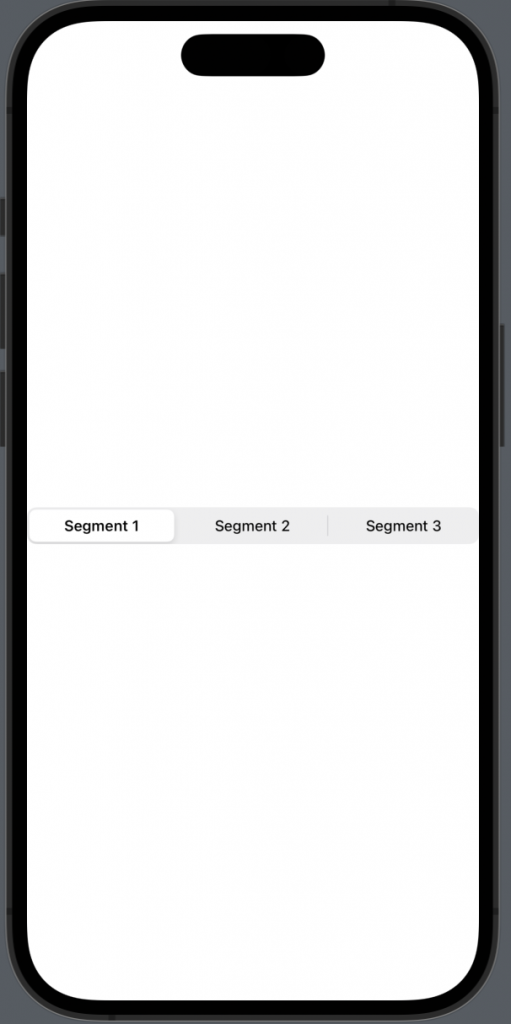
The segmented picker in SwiftUI is a powerful UI element that allows for a streamlined, user-friendly selection process. It’s especially useful when you have a limited set of options and want to make efficient use of screen space.