How to Use Fill and Stroke in SwiftUI Shapes
SwiftUI has brought a paradigm shift in designing UI elements for iOS, and its approach to shapes is no exception. This blog will delve deep into the mechanics of applying fill and stroke to shapes, which are foundational to crafting the look and feel of any app’s UI.
Fill Shapes with Color
When it comes to adding life to shapes, the fill
modifier is your first port of call.
Circle()
.fill(Color.blue)
.frame(width: 100, height: 100)
In this snippet, we create a Circle
and fill it with a solid blue color. The fill
modifier takes a Color
and applies it to the entire area of the shape within the frame we set.
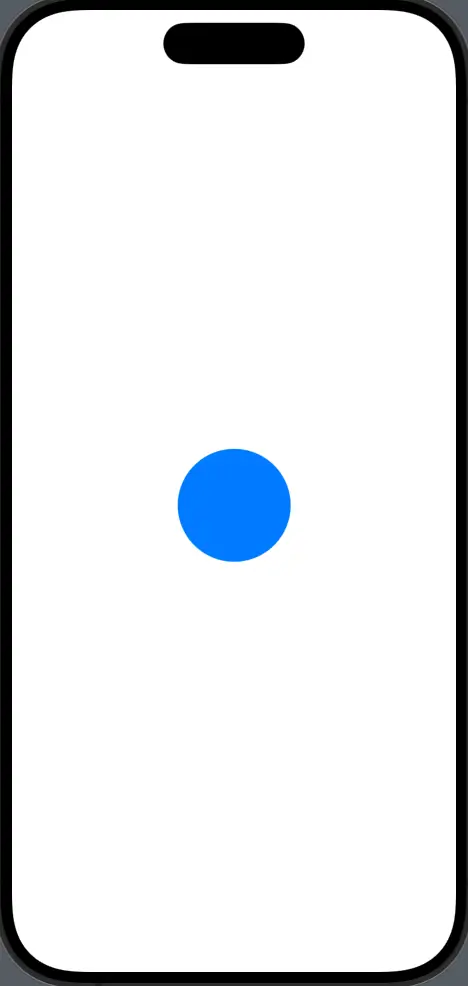
Gradient Fills
For more visually dynamic shapes, SwiftUI supports gradient fills directly within the shape’s fill
modifier.
Rectangle()
.fill(LinearGradient(gradient: Gradient(colors: [Color.red, Color.orange]), startPoint: .leading, endPoint: .trailing))
.frame(width: 200, height: 100)
This code shows a Rectangle
being filled with a LinearGradient
that smoothly transitions from red to orange. The gradient starts from the leading edge and ends at the trailing edge of the rectangle.
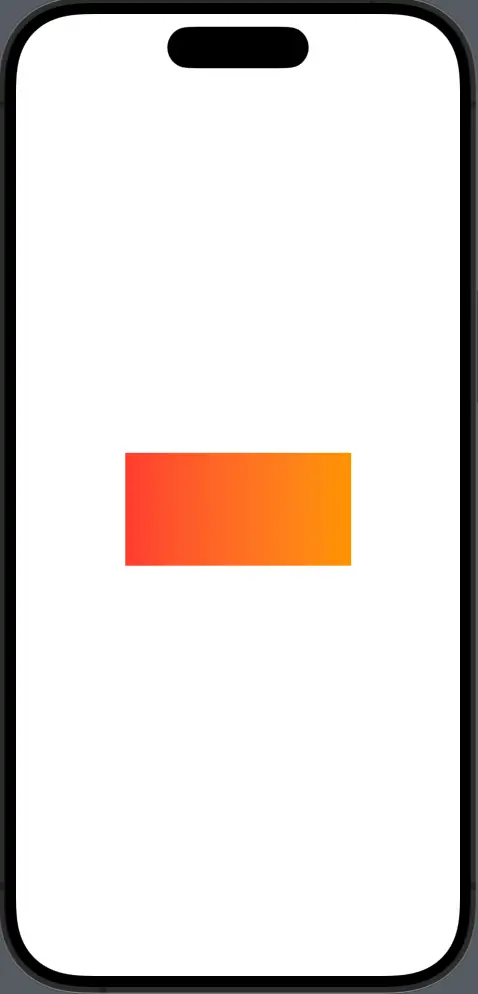
Apply Stroke to Shapes
Strokes outline your shapes. The stroke
modifier lets you define the line weight and color.
Ellipse()
.stroke(Color.green, lineWidth: 4)
.frame(width: 150, height: 100)
An Ellipse
is outlined with a green stroke that’s 4 points thick. The stroke
modifier applies the style to the perimeter of the shape only.
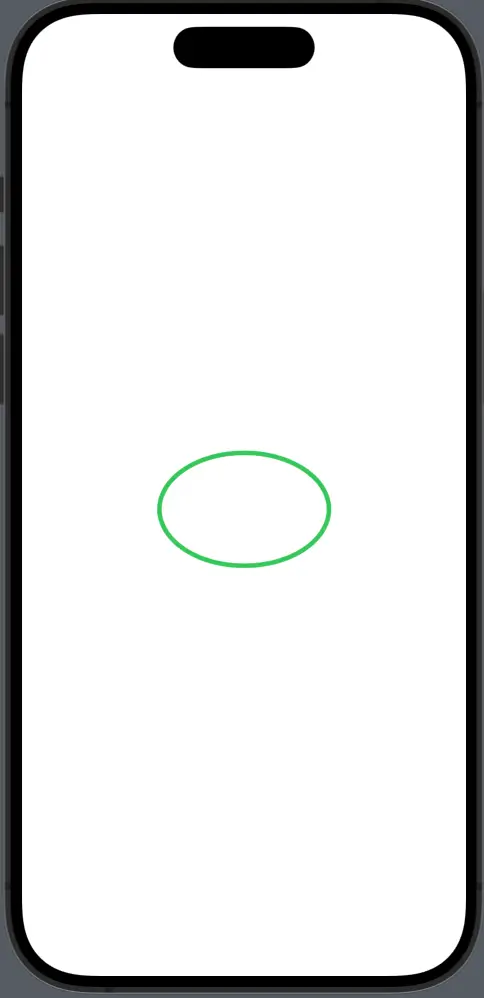
Combine Fill and Stroke
You can also combine both fill
and stroke
. However, it requires a specific approach since applying both modifiers directly to a shape isn’t possible.
Circle()
.strokeBorder(Color.blue, lineWidth: 4)
.background(Circle().fill(Color.red))
.frame(width: 100, height: 100)
The Circle
has a blue stroke and is filled with red. The strokeBorder
modifier is used to apply the stroke, and a background
modifier with another Circle
filled with red is layered beneath it.
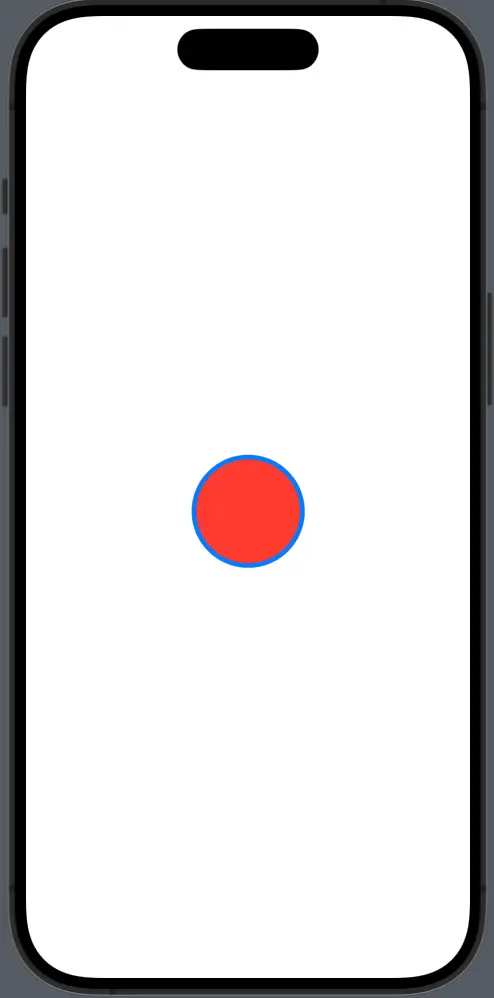
The fill
and stroke
modifiers in SwiftUI provide a simple yet powerful way to style shapes. Whether you’re filling shapes with solid colors, gradients, or combining fill and stroke for a more complex look, these tools are the building blocks for designing intricate and appealing UI elements.
By understanding and mastering these modifiers, you can ensure your shapes not only fit the functional needs of your app but also carry its visual language with grace and clarity.