How to Center Text in iOS SwiftUI TextField
Alignment plays a crucial role in the aesthetics and usability of an application’s user interface. Centering text within a text field is a common design pattern that can add a touch of professionalism and clarity to your app.
In SwiftUI, centering text inside a TextField can be accomplished with just a few simple modifications. This blog post will walk you through how to center text within a TextField using SwiftUI.
Centering Text in SwiftUI TextField
Here’s a simple example of how to center the text within a TextField:
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter text", text: $text)
.textFieldStyle(.plain)
.multilineTextAlignment(.center)
.padding()
}
}
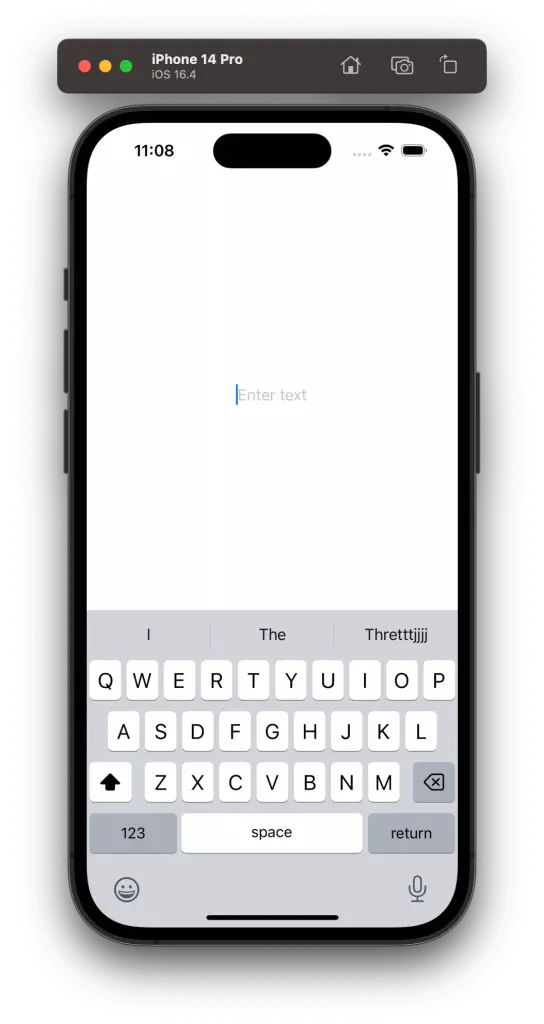
Code Explanation
@State
Property Wrapper
The @State
property wrapper is used to store the text input from the user. It will keep the text value and update the view whenever the text changes.
TextField
The TextField
is initialized with a placeholder “Enter text” and is bound to the text
state variable.
textFieldStyle
Modifier
The textFieldStyle
modifier is used with the plain
to remove any default styling that might interfere with our text alignment.
multilineTextAlignment
Modifier
The multilineTextAlignment
modifier is where the text alignment is set to .center
. This ensures that the text within the TextField is centered.
padding
Modifier
Padding is added around the TextField to give it some breathing room within the view.
Handling Different States
Depending on the state of the TextField (active, inactive, filled, empty), you might want to apply different stylings or logic. This can be accomplished using additional conditional statements and SwiftUI modifiers.
Centering text in a SwiftUI TextField can provide a polished and clear user experience. With the combination of textFieldStyle
, multilineTextAlignment
, and other SwiftUI modifiers, you can easily achieve centered text and further customize the TextField to fit your app’s design.