How to Add Text to Shapes in SwiftUI
SwiftUI makes it incredibly easy to combine shapes and text, enabling you to create custom buttons, labels, and other interactive elements. This blog post will take you through the steps of adding text to shapes in SwiftUI, providing you with the knowledge to enhance the user interface of your app.
Combine Text and Shapes
Let’s start by putting text over a simple shape. SwiftUI’s ZStack
is perfect for layering views on top of each other.
import SwiftUI
struct ContentView: View {
var body: some View {
ZStack {
Circle()
.fill(Color.blue)
.frame(width: 150, height: 150)
Text("Hello, World!")
.foregroundColor(.white)
}
}
}
Here, a blue circle serves as the backdrop for the white “Hello, World!” text. The ZStack
ensures the text is positioned directly over the center of the circle.
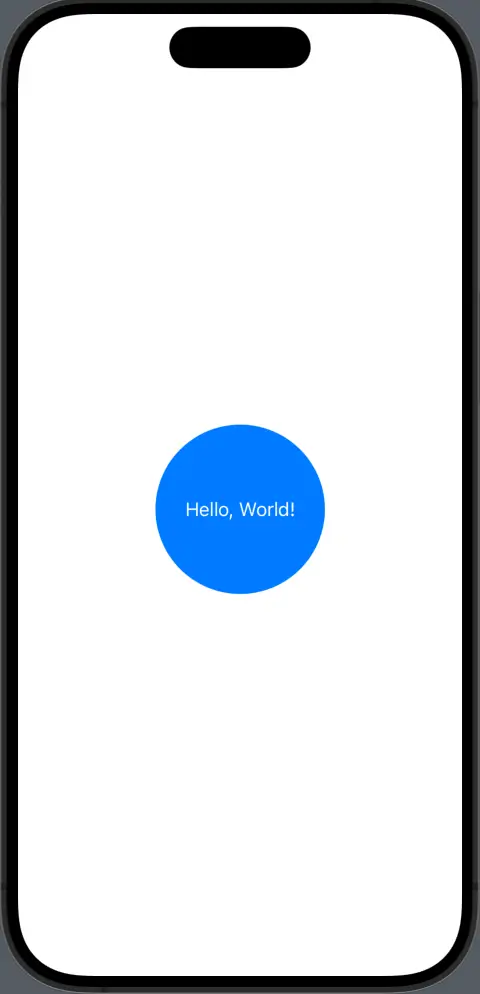
Customize Text Style
To make text more readable and visually appealing, you can apply various styles to it.
ZStack {
RoundedRectangle(cornerRadius: 10)
.fill(Color.green)
.frame(width: 200, height: 100)
Text("Hello")
.font(.title)
.bold()
.foregroundColor(.white)
}
In the above code, the text “Hello” is styled with a title font and bold weight, which stands out clearly against the green rounded rectangle background.
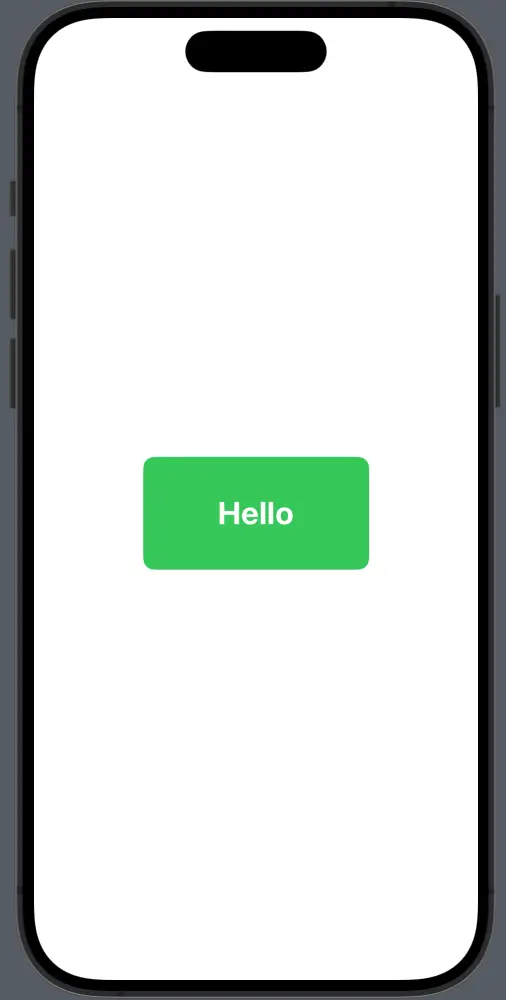
Align Text within Shapes
You might want to align your text differently depending on the design. SwiftUI gives you the control to do just that.
ZStack(alignment: .bottomTrailing) {
Capsule()
.fill(Color.orange)
.frame(width: 200, height: 100)
Text("Bottom Right")
.padding()
.foregroundColor(.white)
}
With ZStack
alignment set to .bottomTrailing
, the text “Bottom Right” is positioned at the bottom-right corner of the orange capsule shape.
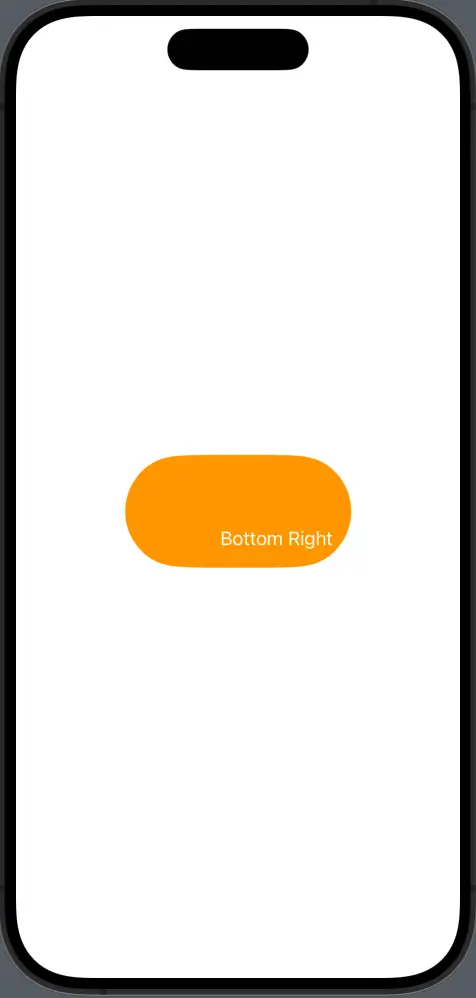
Embed Text Inside Shapes
For more complex designs, you can embed the text within the border of a shape.
Text("Embedded")
.padding()
.background(Capsule().stroke(Color.blue, lineWidth: 4))
Here, “Embedded” is surrounded by a capsule-shaped border. The padding
around the text ensures there is some space between the text and the border.
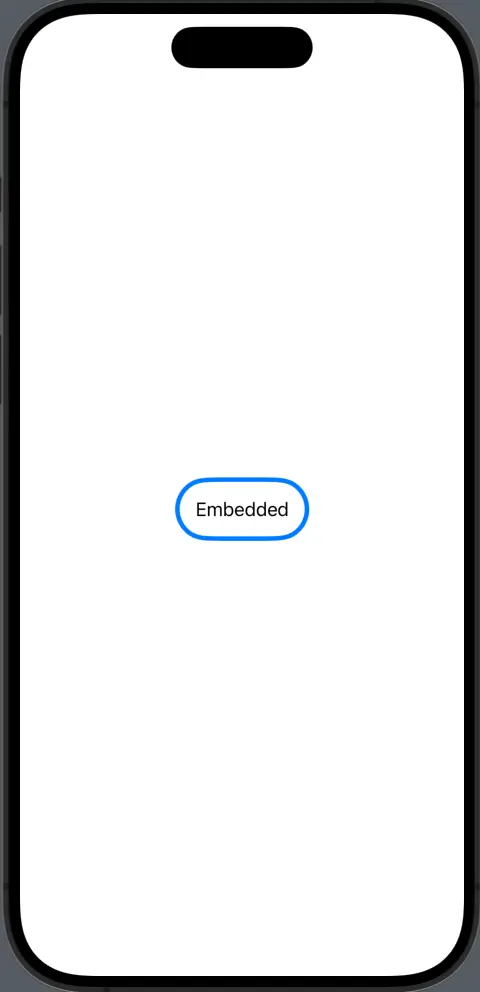
Adding text to shapes in SwiftUI can be both functional and aesthetically pleasing. Whether it’s for creating custom buttons, badges, or icons, the combination of text and shapes is fundamental in many design patterns. By adjusting the styles, alignment, and making your components reusable, you can create a consistent and interactive UI.
Experiment with these techniques to discover the possibilities that SwiftUI offers for integrating text and shapes. Your app’s design can benefit greatly from the careful use of these elements.