How to Create Oval Shape in SwiftUI
SwiftUI’s shape library is quite extensive and provides a plethora of options to create and manipulate different shapes, including ovals. In this blog post, I’ll walk you through creating an oval shape in SwiftUI, customizing it, and applying it to your UI design.
Unlike other UI frameworks that may require you to draw an oval shape manually, SwiftUI simplifies this process. An oval can be created by modifying the Ellipse
shape which is inherently available in SwiftUI.
Basic Oval
To start, let’s create a basic oval. In SwiftUI, this can be achieved by using the Ellipse
shape and adjusting its frame.
Ellipse()
.fill(Color.blue)
.frame(width: 200, height: 100)
The code snippet above produces an oval with a blue fill. The frame
modifier’s width and height parameters determine the oval’s aspect ratio — a wider width relative to height stretches the oval horizontally, and vice versa.
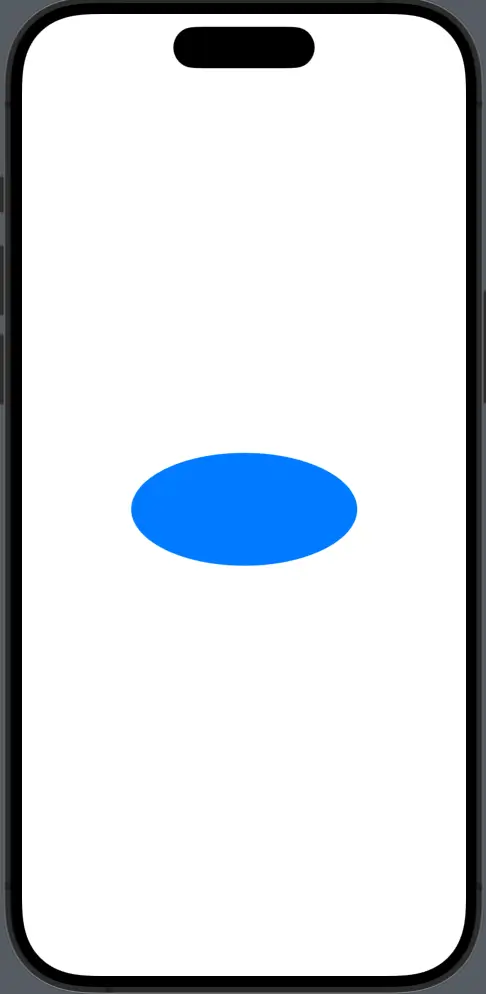
Style the Oval
You can style an oval in much the same way as any other shape in SwiftUI. Let’s give our oval a stroke, a shadow, and make it semi-transparent.
Ellipse()
.stroke(Color.green, lineWidth: 5)
.shadow(radius: 10)
.opacity(0.5)
.frame(width: 300, height: 150)
This oval has a green border that’s 5 points thick, a shadow that gives it depth, and it’s semi-transparent, allowing the background to seep through slightly.
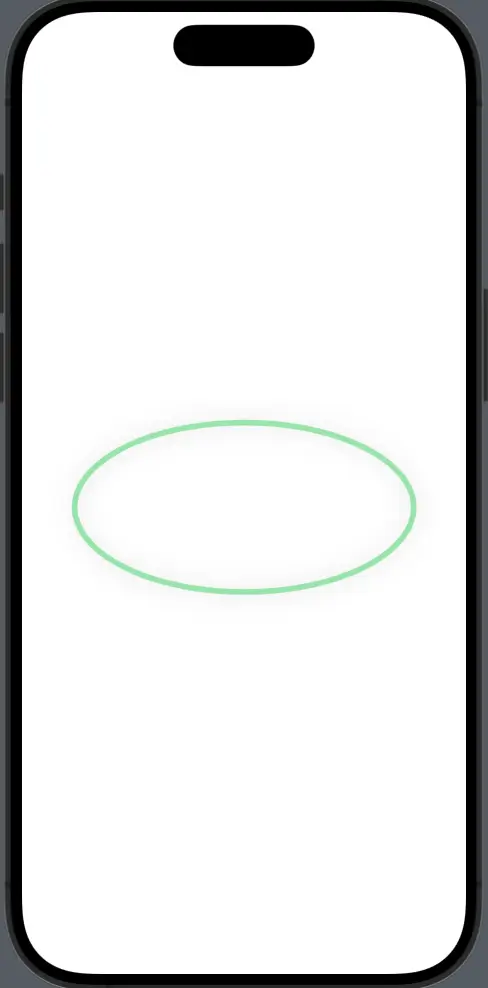
Use Ovals as Clipping Masks
Ovals can also be used as clipping masks to give other views an oval-shaped boundary.
import SwiftUI
struct ContentView: View {
var body: some View {
Image("dog")
.frame(width: 350, height: 300)
.clipShape(Ellipse())
}
}
In this example, an image named “dog” is clipped to fit within the bounds of an Ellipse
, thus taking on an oval shape.
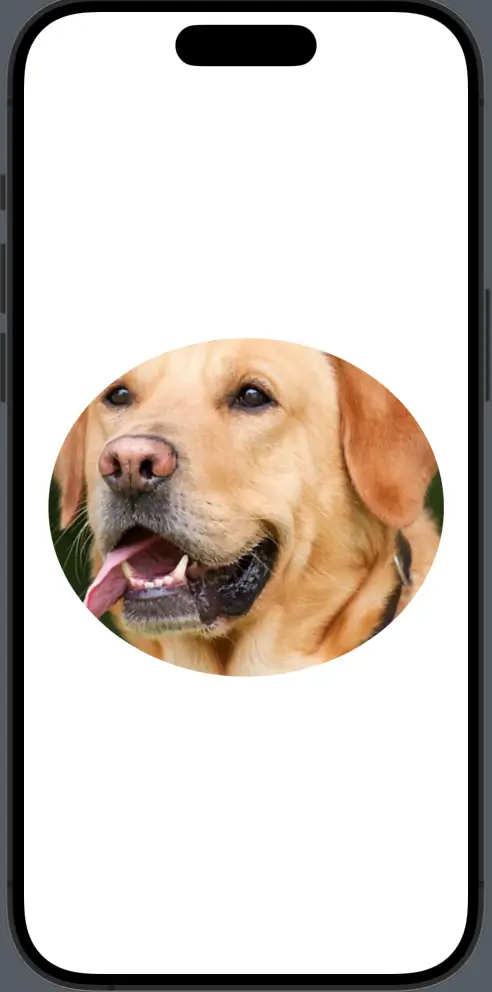
The oval shape is a versatile tool in the SwiftUI designer’s toolkit. Whether you’re using it as a standalone element, styling it with strokes and shadows, or clipping other views to its form. By mastering how to work with ovals, you can add a variety of elegant and eye-catching elements to your SwiftUI applications.
Experiment with these techniques to explore the full range of design possibilities that ovals can offer to your user interface.