How to Add Label to ProgressView in iOS SwiftUI
Progress indicators are crucial in modern apps to inform users about ongoing tasks. While the visual indicator is important, adding a label can provide additional context or information. In this blog post, we’ll explore how to add labels to ProgressView
in iOS SwiftUI, complete with examples and best practices.
What is a ProgressView?
A ProgressView
in SwiftUI is a UI component that indicates the progress of a task. It can be either determinate, showing the exact percentage of completion, or indeterminate, displaying a general waiting indicator.
Add a Basic Label
Example: Simple Label
Here’s how you can add a simple label to a ProgressView
:
import SwiftUI
struct ContentView: View {
var body: some View {
ProgressView("Downloading...")
}
}
The label “Downloading…” provides context to the user, indicating that a download is in progress.
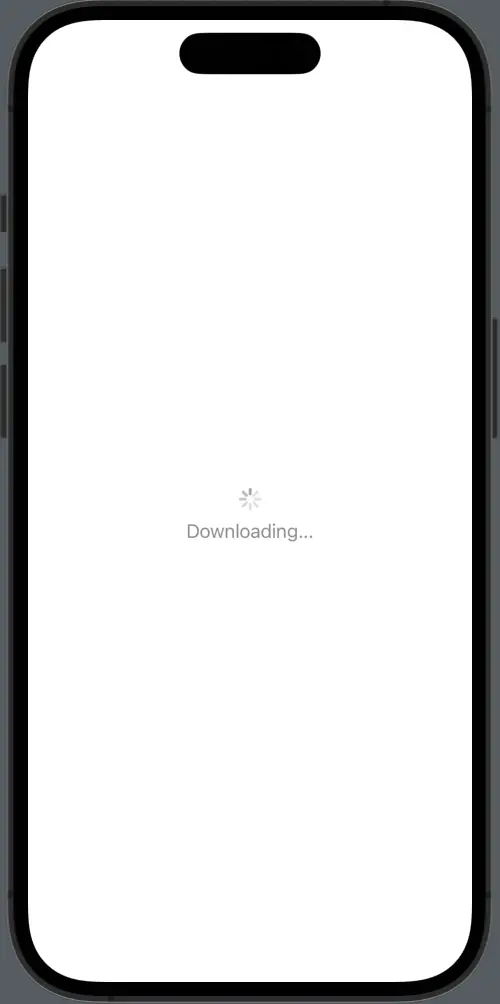
Add a Label to Determinate ProgressView
Example: Label with Percentage
You can also add a label to a determinate ProgressView
to show the percentage of completion.
import SwiftUI
struct ContentView: View {
var body: some View {
ProgressView("Downloading", value: 0.5, total: 1)
}
}
The label “Downloading” is accompanied by a progress bar that shows 50% completion. The label provides context, while the progress bar shows the exact progress.
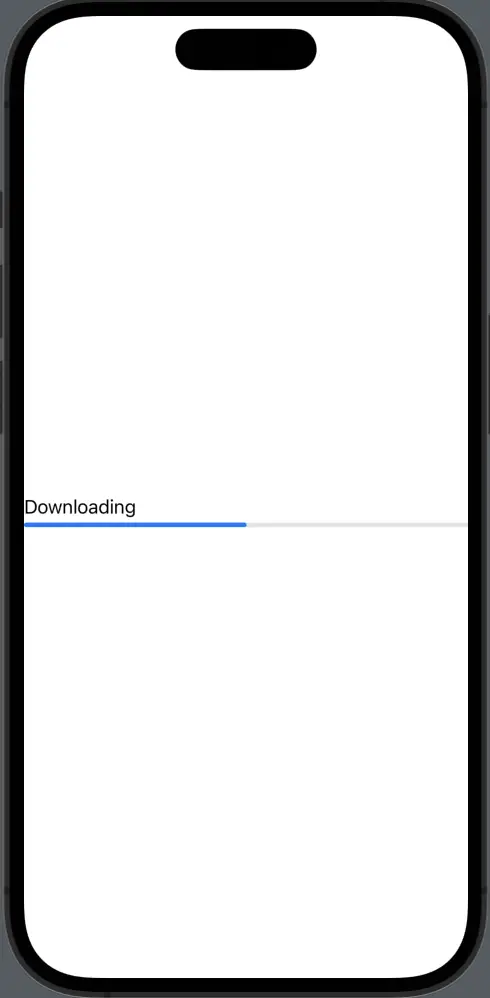
Customize the Label
Example: Styled Label
You can style the label using standard SwiftUI modifiers.
import SwiftUI
struct ContentView: View {
var body: some View {
ProgressView("Downloading", value: 0.5, total: 1).font(.largeTitle)
.foregroundColor(.red)
}
}
Here, the label is styled with a large title font and red text color, making it more prominent.
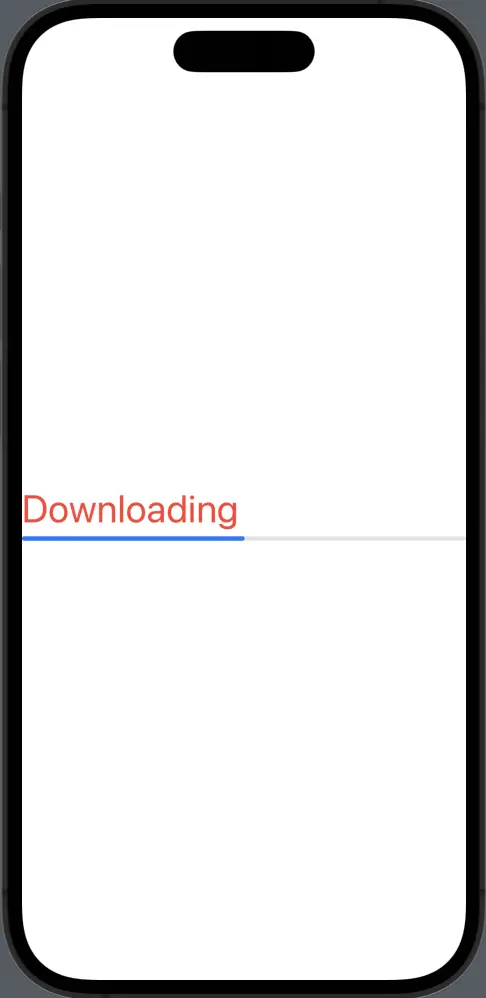
Dynamic Labels
Example: Update Label Text
You can use state variables to dynamically update the label text.
@State private var progress: Double = 0.5
@State private var labelText: String = "Downloading"
var body: some View {
ProgressView(labelText, value: progress, total: 1)
.onAppear {
if progress >= 1 {
labelText = "Download Complete"
}
}
}
The label text is bound to a state variable labelText
. When the progress reaches 100%, the label updates to “Download Complete.”
Adding a label to ProgressView
in SwiftUI is a straightforward yet powerful way to provide additional context to your progress indicators. Whether you’re showing a simple message, indicating the percentage of completion, or dynamically updating the label, SwiftUI offers the flexibility to do it all.