How to Add ProgressView in iOS SwiftUI
Progress indicators are essential UI elements that inform users about the status of ongoing tasks. SwiftUI offers a simple yet powerful way to create these indicators through the ProgressView
element.
In this blog post, we’ll explore the ins and outs of using ProgressView
in SwiftUI, complete with examples and code explanations.
What is SwiftUI ProgressView?
ProgressView
in SwiftUI is a UI element that displays the progress of a task. It can be used in two primary ways: as an indeterminate loader that spins continuously or as a determinate loader that fills up as a task progresses.
Basic Usage of ProgressView
Indeterminate ProgressView
An indeterminate ProgressView
doesn’t show the percentage of completion; instead, it uses a continuous animation to indicate that a task is ongoing.
Example: Basic Indeterminate ProgressView
import SwiftUI
struct ContentView: View {
var body: some View {
ProgressView()
}
}
This is the simplest way to create an indeterminate ProgressView
. It will display a spinning loader by default.
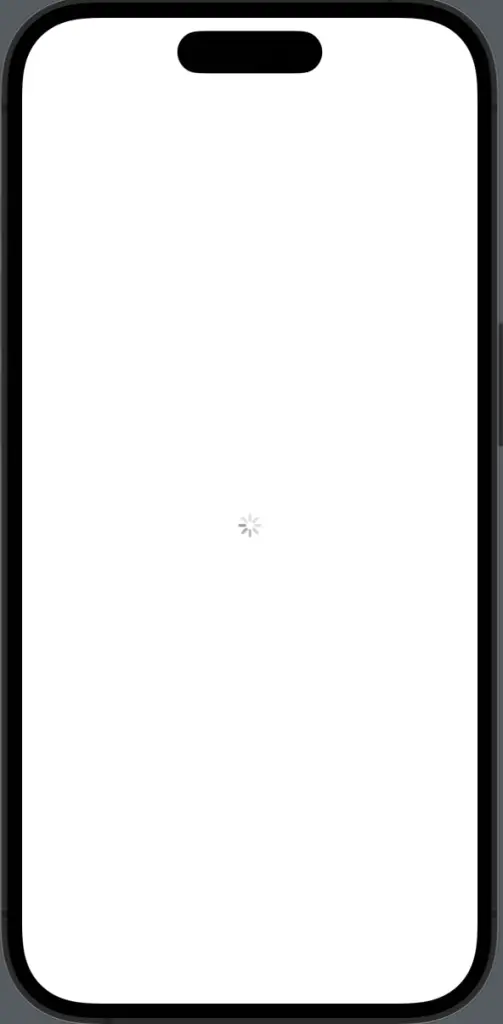
Determinate ProgressView
A determinate ProgressView
shows the percentage of completion, usually as a horizontal bar that fills up.
Example: Basic Determinate ProgressView
import SwiftUI
struct ContentView: View {
@State private var progress: Double = 0.5
var body: some View {
ProgressView(value: progress, total: 1.0)
}
}
Here, we have a @State
variable progress
that holds the current progress value. The ProgressView
takes this value and a total (1.0 in this case) to display the progress.
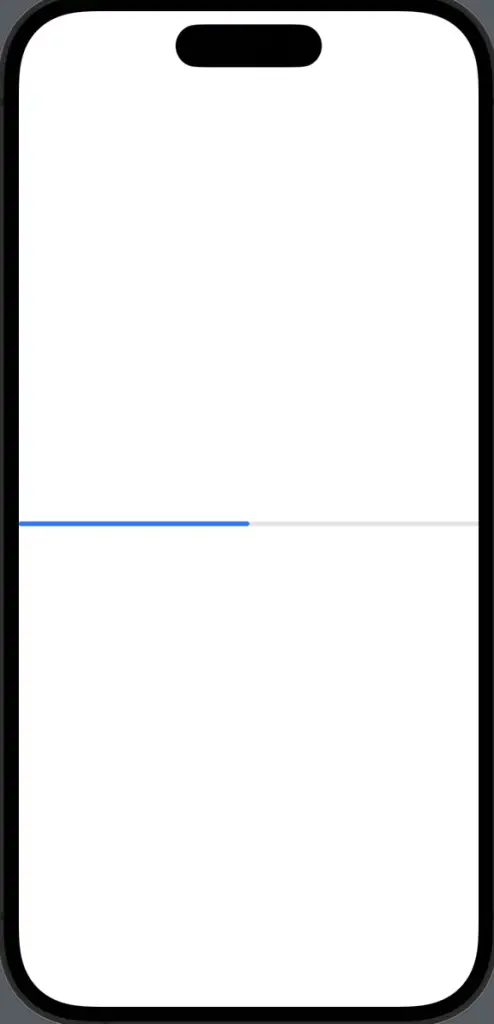
Customize ProgressView
Change the Appearance
You can change the appearance of ProgressView
using various modifiers.
Example: Colored ProgressView
import SwiftUI
struct ContentView: View {
@State private var progress: Double = 0.5
var body: some View {
ProgressView(value: progress, total: 1.0).progressViewStyle(.linear).tint(.red)
}
}
The progressViewStyle
modifier is used to change the appearance. Here, we set the tint color to red.
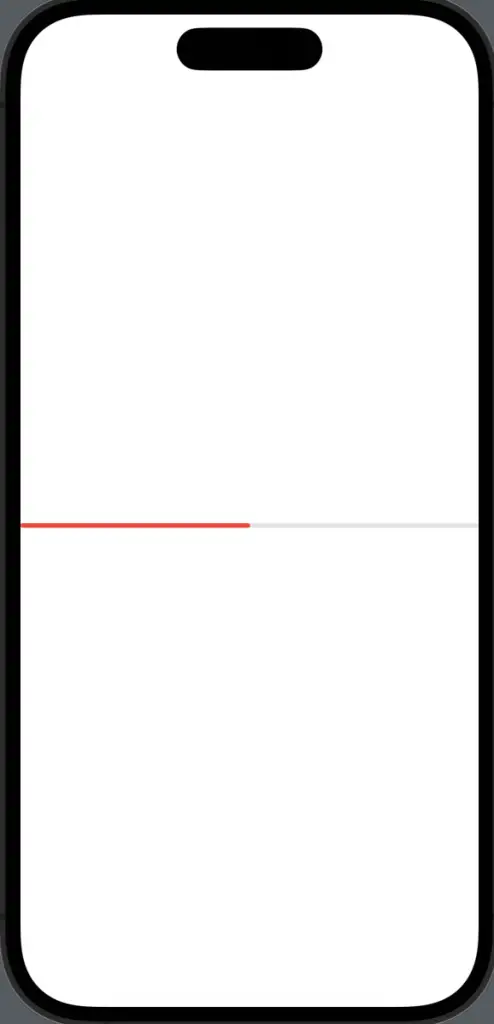
Add Labels
You can add labels to provide additional information.
Example: ProgressView with Label
ProgressView("Loading...", value: progress, total: 1.0)
The first parameter in ProgressView
is the label. In this example, it displays “Loading…” next to the progress bar.
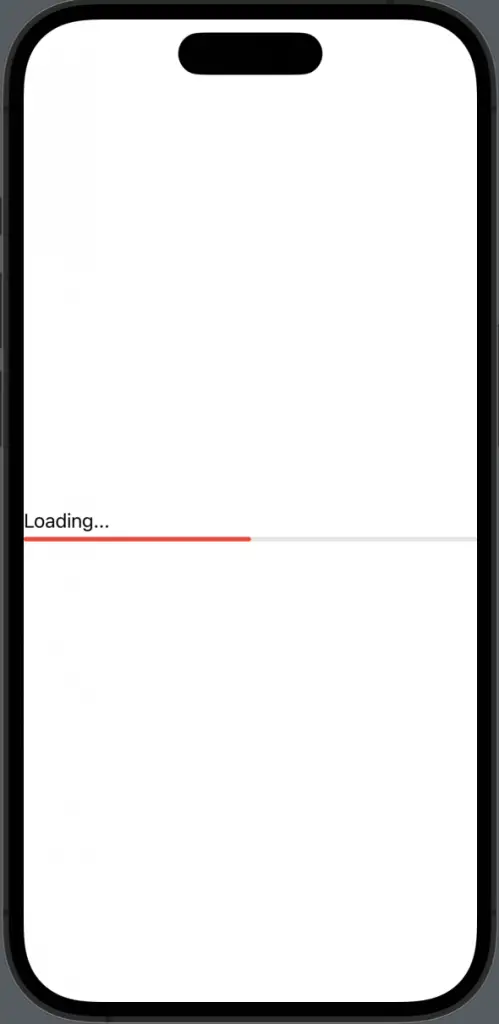
Advanced Usage
Dynamic Progress Updates
You can dynamically update the progress by binding it to a state variable.
Example: Dynamic ProgressView
@State private var progress: Double = 0
Button("Update Progress") {
progress += 0.1
}
ProgressView(value: progress, total: 1.0)
Explanation: Here, we have a button that updates the progress
state variable. The ProgressView
reflects these changes automatically.
ProgressView
in SwiftUI offers a simple yet effective way to display task progress. Whether you need a basic loader or a customized, dynamic progress indicator, ProgressView
has got you covered.
One Comment