SwiftUI Alert vs ConfirmationDialog: A Comparative Guide with Examples
In SwiftUI, both Alert
and ConfirmationDialog
are used to capture user decisions or provide important information. While they may seem similar, there are key differences that make each suitable for specific use-cases.
In this blog post, we’ll compare Alert
and ConfirmationDialog
in SwiftUI, complete with examples to help you decide which to use in different scenarios.
What is an Alert?
An Alert
is a pop-up message that appears on the screen to notify the user or capture a decision. It usually contains a title, a message, and one or more buttons.
Example: Basic Alert
Here’s a simple example of an alert:
import SwiftUI
struct ContentView: View {
@State private var showAlert = false
var body: some View {
Button("Show Alert") {
showAlert = true
}
.alert(isPresented: $showAlert) {
Alert(title: Text("Hello, SwiftUI!"))
}
}
}
The showAlert
state variable controls the visibility of the alert. When the button is clicked, the alert appears with the title “Hello, SwiftUI!”.
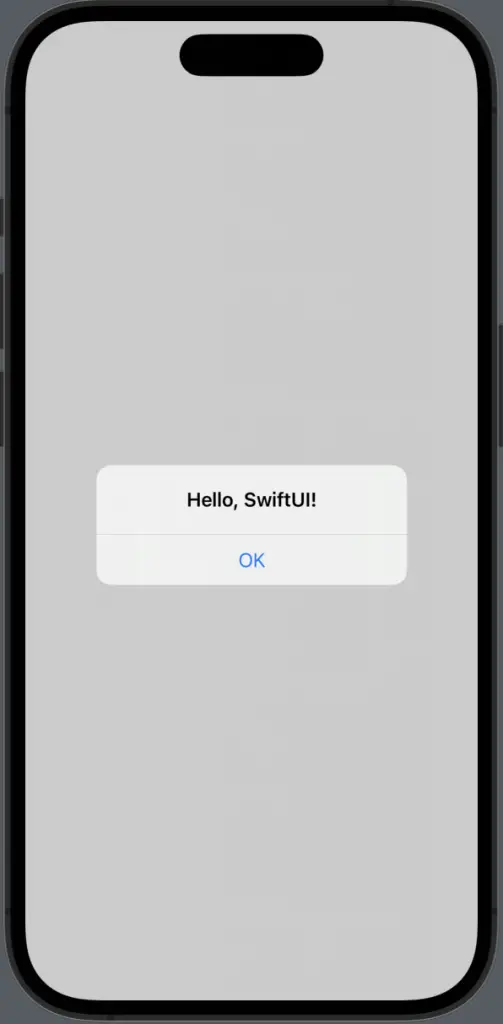
What is a ConfirmationDialog?
A ConfirmationDialog
is similar to an alert but is generally used to confirm an action. It also contains a title, a message, and buttons.
Example: Basic ConfirmationDialog
Here’s a simple example of a ConfirmationDialog
:
import SwiftUI
struct ContentView: View {
@State private var showConfirmation = false
var body: some View {
Button("Delete File") {
showConfirmation = true
}
.confirmationDialog("Are you sure?",
isPresented: $showConfirmation,
titleVisibility:Visibility.visible ) {
Button("Yes, Delete it") {
// Perform delete action
}
Button("Cancel") {
// Cancel action
}
}
}
}
The showConfirmation
state variable controls the visibility of the dialog. When the button is clicked, the dialog appears with the title “Are you sure?” and two buttons for user interaction.
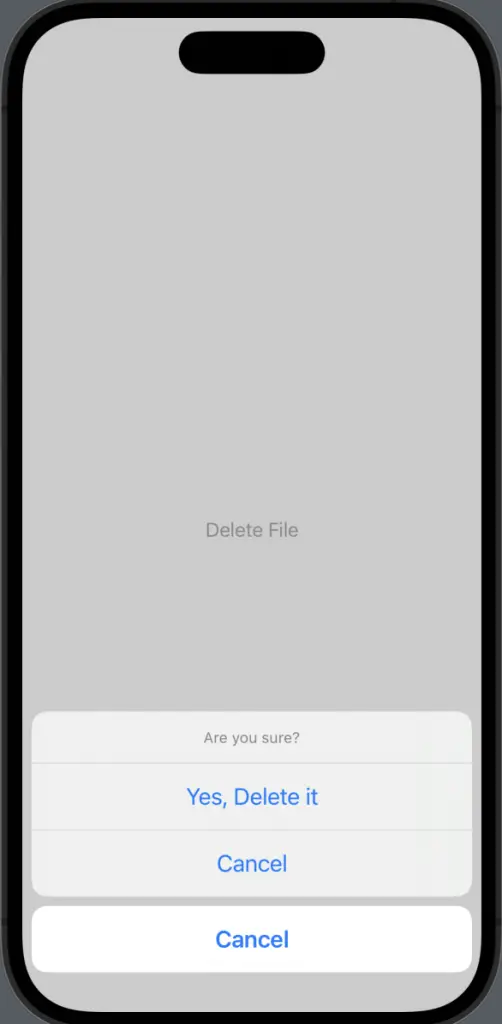
Key Differences
Context
- Alert: Used for notifications and simple decisions.
- ConfirmationDialog: Specifically designed for confirming actions.
Customization
- Alert: Limited customization options.
- ConfirmationDialog: More flexible, allowing for additional text and multiple buttons.
Platform Availability
- Alert: Available on all Apple platforms.
- ConfirmationDialog: Primarily designed for iOS and may not be fully supported on other platforms.
User Experience
- Alert: More intrusive, capturing immediate attention.
- ConfirmationDialog: Less intrusive, allowing for a smoother user experience.
Both Alert
and ConfirmationDialog
have their own use-cases and advantages. Alert
is more suitable for simple notifications and decisions, while ConfirmationDialog
is better for confirming actions and offers more customization options.
Understanding these differences will help you make an informed choice for your SwiftUI app.