How to Enable Autofocus in React Native TextInput
In mobile apps, automatically focusing on a particular input field can significantly enhance the user experience. With React Native, enabling autofocus in the TextInput
component is relatively straightforward.
In this blog post, we’ll delve into using the autoFocus
prop and its practical applications.
Prerequisites
- Basic knowledge of React Native and JavaScript
- A working React Native development environment
A Simple TextInput Example
Before we jump into autofocus, let’s look at a simple example of using the TextInput
component.
import React from 'react';
import { View, TextInput } from 'react-native';
const App = () => {
return (
<View>
<TextInput placeholder="Enter text here" />
</View>
);
};
Enable Autofocus
To automatically focus on a TextInput
when a screen renders, you can use the autoFocus
prop.
<TextInput placeholder="I will be focused" autoFocus={true} />
The autoFocus
prop, when set to true
, automatically focuses on the TextInput
as soon as it appears on the screen.
Use Cases for Autofocus
1. Login Screens
Automatically focusing on the username or email field can speed up the login process.
2. Search Bars
When a user navigates to a search screen, automatically focusing on the search bar is often expected behavior.
Handle Multiple TextInputs
In forms with multiple fields, the next input field can be auto-focused when the user finishes with the current one.
import React from 'react';
import {View, TextInput} from 'react-native';
const App = () => {
const nextInput = React.createRef();
return (
<View>
<TextInput
placeholder="First Field"
returnKeyType="next"
autoFocus
onSubmitEditing={() => nextInput.current.focus()}
/>
<TextInput ref={nextInput} placeholder="Second Field" />
</View>
);
};
export default App;
We use React.createRef()
to create a reference for the next TextInput
. The onSubmitEditing
prop allows us to trigger a function that sets focus to the next input.
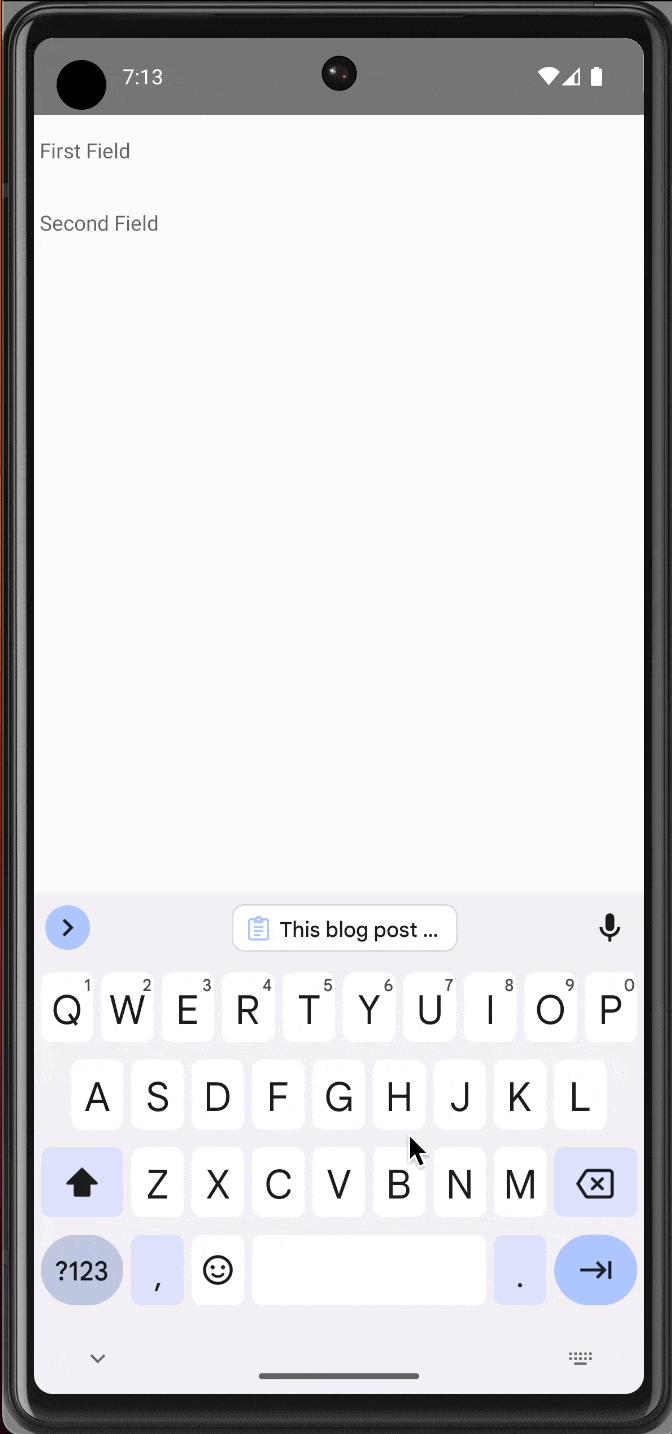
The autoFocus
feature in React Native’s TextInput
component is a simple yet effective tool for enhancing user experience. Whether it’s a login screen, a search bar, or a multi-field form, setting the focus automatically can make the interaction smoother and quicker.