SwiftUI: LazyVGrid vs LazyVStack
In SwiftUI, understanding the differences between LazyVGrid and LazyVStack is important for creating an optimized, responsive layout. In this blog post, we’re diving into these two SwiftUI view types to provide a clear understanding of when and how to use each, along with illustrative examples.
SwiftUI LazyVGrid
LazyVGrid is a SwiftUI view that organizes its child views into a grid that scrolls vertically. As the name implies, it creates items “lazily,” which means it only generates views as they appear on screen. This leads to more efficient performance, particularly when dealing with large amounts of data.
LazyVGrid Example
Here is a basic example of LazyVGrid which displays a set of numbers in a vertical grid layout:
struct ContentView: View {
let gridItems = Array(repeating: GridItem(.flexible()), count: 2)
var body: some View {
ScrollView {
LazyVGrid(columns: gridItems, spacing: 20) {
ForEach(1...50, id: \.self) { index in
Text("\(index)")
.frame(width: 100, height: 100)
.background(Color.orange)
.cornerRadius(10)
}
}
}
}
}
In this example, the numbers 1 through 50 are displayed in orange boxes. The grid will scroll vertically.
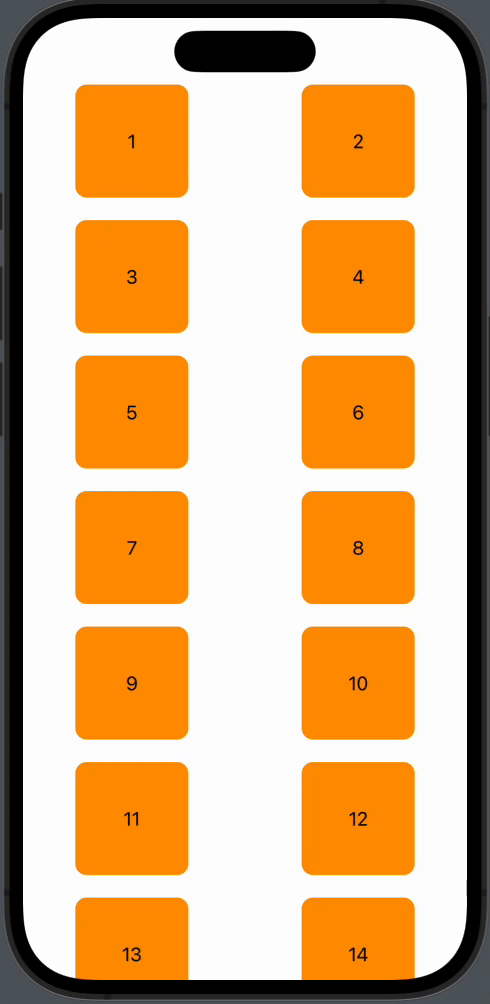
SwiftUI LazyVStack
On the other hand, LazyVStack is a SwiftUI view that arranges its child views in a vertical line. Like the LazyVGrid, it also creates items “lazily.” However, unlike LazyVGrid, LazyVStack does not organize items in multiple columns. Instead, it stacks views in a single column.
LazyVStack Example
Here is a simple example of LazyVStack which displays a list of numbers stacked vertically:
struct ContentView: View {
var body: some View {
ScrollView {
LazyVStack(spacing: 20) {
ForEach(1...50, id: \.self) { index in
Text("\(index)")
.frame(width: 100, height: 100)
.background(Color.blue)
.cornerRadius(10)
}
}
}
}
}
In this example, the numbers 1 through 50 are displayed in blue boxes. The items are arranged in a single column and the stack will scroll vertically.
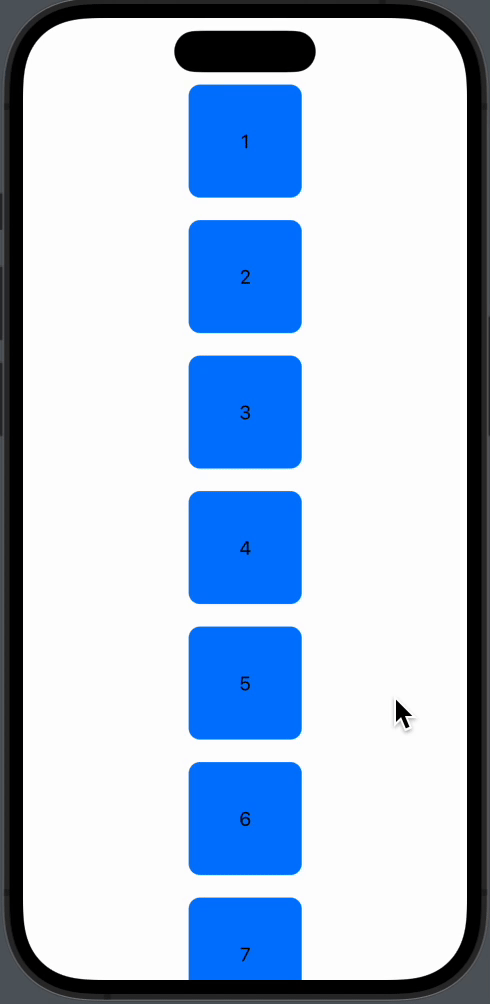
Comparison of LazyVGrid and LazyVStack
The main difference between LazyVGrid and LazyVStack lies in their layout. While LazyVGrid arranges its children in multiple columns that scroll vertically, LazyVStack places its children in a single column that also scrolls vertically.
You should use LazyVGrid when you need a flexible grid interface with multiple columns. Conversely, you should use LazyVStack when you need a single column vertical list of items.
Both LazyVGrid and LazyVStack have unique strengths that can be leveraged based on the layout requirements of your SwiftUI app. With a good understanding of their differences and use cases, you can select the one that best fits your needs.