How to Add Slider Step in iOS SwiftUI
Sliders are essential UI components that empower users to input or select values within a defined range. In SwiftUI, creating sliders is straightforward, but controlling the increments at which values change requires more understanding.
This blog post explores the usage of step increments to enhance the precision and user experience of sliders in SwiftUI.
Create a Slider with Step Increments
Here’s an example demonstrating how to create a slider with defined step increments in SwiftUI:
import SwiftUI
struct ContentView: View {
@State private var sliderValue = 0.5
var body: some View {
Slider(value: $sliderValue, in: 0...1, step: 0.1)
.padding()
}
}
Code Breakdown
State Variable:
The sliderValue
state variable holds the current value of the slider.
Slider Initialization:
We initialize a Slider with a value range of 0 to 1 and bind it to the sliderValue
state variable.
Step Increment:
The step
parameter is set to 0.1, indicating that the slider’s value changes in increments of 0.1.
Padding:
Padding is added to ensure proper spacing and a visually pleasing layout.
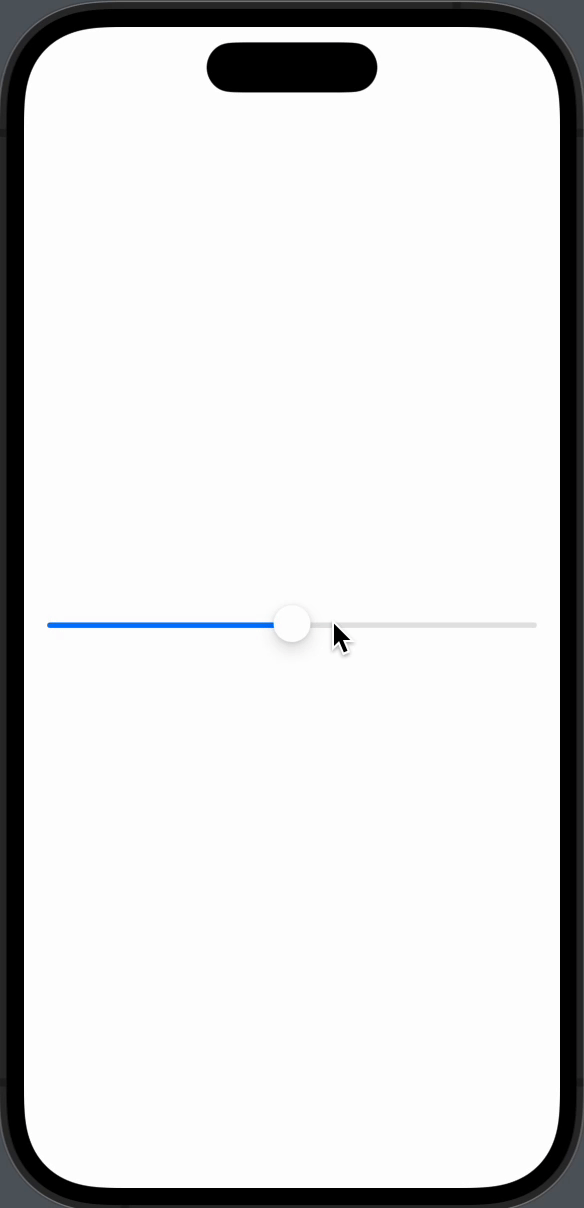
Advantages of Using Step Increments
Precision
By setting step increments, you ensure that users can select values with precision, especially when dealing with numerical inputs or settings that require specific levels.
Guided Input
Step increments guide users’ selections, making it easier for them to navigate through a range of values without worrying about fine-tuning.
Consistency
When users move the slider, they’ll notice consistent jumps, creating a predictable interaction pattern that enhances usability.
Incorporating step increments into your SwiftUI sliders can elevate the precision and user experience of your app’s UI. The provided example showcases how to apply step increments effectively.