SwiftUI: LazyVStack vs VStack
In SwiftUI, both VStack and LazyVStack are essential components for arranging views vertically in a stack. But how do they differ? This blog post will explore these differences and help you understand when to use VStack and when to opt for LazyVStack.
SwiftUI VStack
VStack is a SwiftUI view that arranges its children in a vertical line. It’s an incredibly straightforward and easy-to-use way to organize your views. VStack is helpful when you have a small, finite number of child views to display, and it’s certain that these views won’t consume substantial memory.
VStack Example
Here’s an example of VStack in SwiftUI:
struct ContentView: View {
var body: some View {
VStack {
Text("Hello")
.font(.largeTitle)
.padding()
Text("World!")
.font(.title)
.padding()
}
}
}
This VStack contains two Text views stacked vertically, the first one saying “Hello” and the second “World!”.
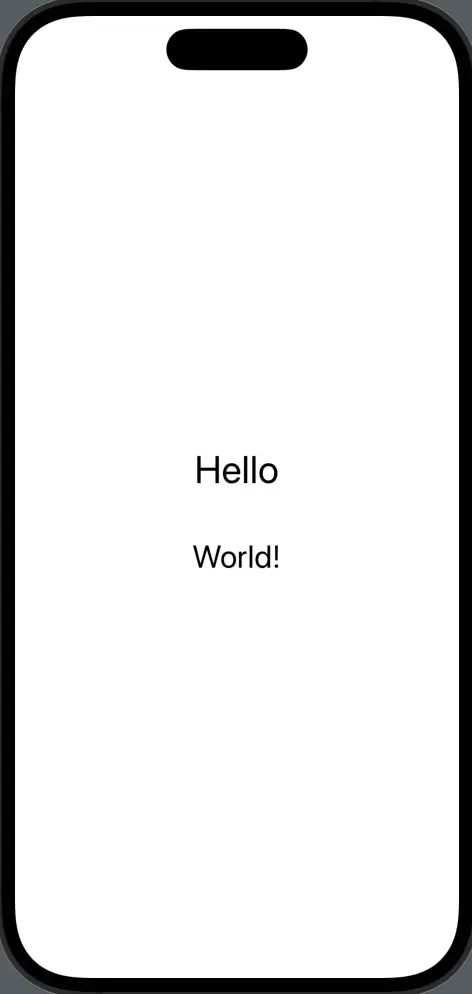
SwiftUI LazyVStack
LazyVStack, as the name suggests, is a SwiftUI view that “lazily” arranges its children in a vertical line. It only creates the items that are currently visible on screen, which can significantly improve performance when working with large sets of data.
LazyVStack Example
Here’s an example of a LazyVStack in SwiftUI:
struct ContentView: View {
var body: some View {
ScrollView {
LazyVStack {
ForEach(1...1000, id: \.self) { number in
Text("Row \(number)")
}
}
}
}
}
This LazyVStack creates a large number of Text views, each displaying a different row number. However, SwiftUI only initializes the views that are currently visible, greatly optimizing performance.
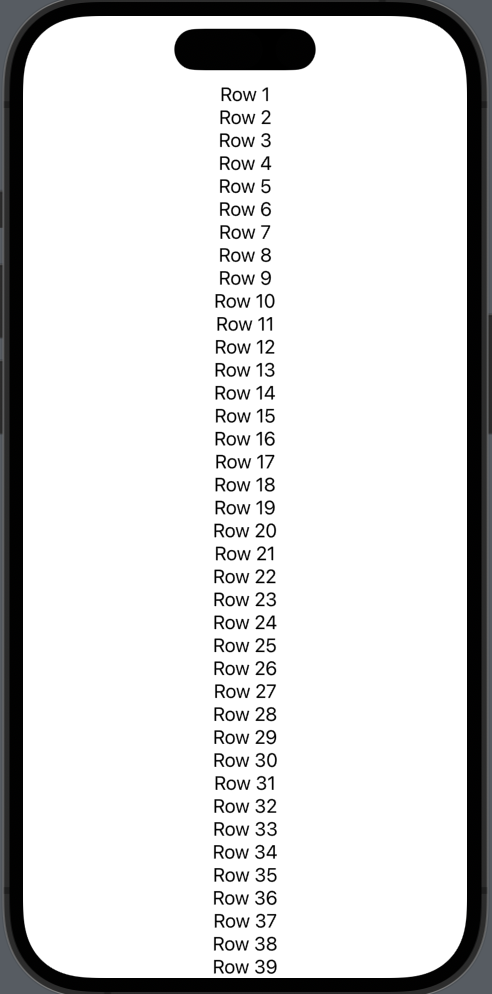
Differences Between VStack and LazyVStack
The primary difference between VStack and LazyVStack lies in how they handle memory. VStack initializes all of its children up front, making it ideal for a small number of child views. LazyVStack, on the other hand, initializes its children as they become visible, making it much more memory-efficient for larger collections.
When to Use VStack or LazyVStack?
The choice between VStack and LazyVStack depends on the specific needs of your application. If you’re dealing with a small, known set of child views, VStack is the way to go. On the other hand, if you have a large number of child views or a dynamic set of views, LazyVStack would be the better option due to its memory efficiency.
Both VStack and LazyVStack play vital roles in SwiftUI, and knowing when to use each can help improve the performance and efficiency of your app. Always remember to consider the size and complexity of your child views when deciding between VStack and LazyVStack.