How to Add LazyHStack in iOS SwiftUI
In SwiftUI, LazyHStack is a powerful view for creating horizontal, scrollable layouts efficiently. It’s a great tool for developers looking to showcase content in a horizontal line, optimizing performance by loading only the views currently in sight.
This blog post will guide you through the fundamental aspects of LazyHStack, including a practical example.
What is LazyHStack?
LazyHStack is a container view in SwiftUI that arranges its children along a horizontal axis and only renders views that are currently within the visible frame. This can lead to significant improvements in performance, especially when handling large collections of views.
How LazyHStack Works
The “Lazy” part of LazyHStack means that views are created as they become visible. This contrasts with standard HStack, which creates all of its child views up front. This lazy loading can provide a more responsive user experience, especially when scrolling through large amounts of content.
A Simple Example of LazyHStack
Let’s take a look at a practical example to better understand how LazyHStack can be implemented:
struct ContentView: View {
var body: some View {
ScrollView(.horizontal, showsIndicators: false) {
LazyHStack {
ForEach(1...1000, id: \.self) { number in
Text("\(number)")
.frame(width: 80, height: 80)
.background(Color.orange)
.cornerRadius(10)
}
}
}
}
}
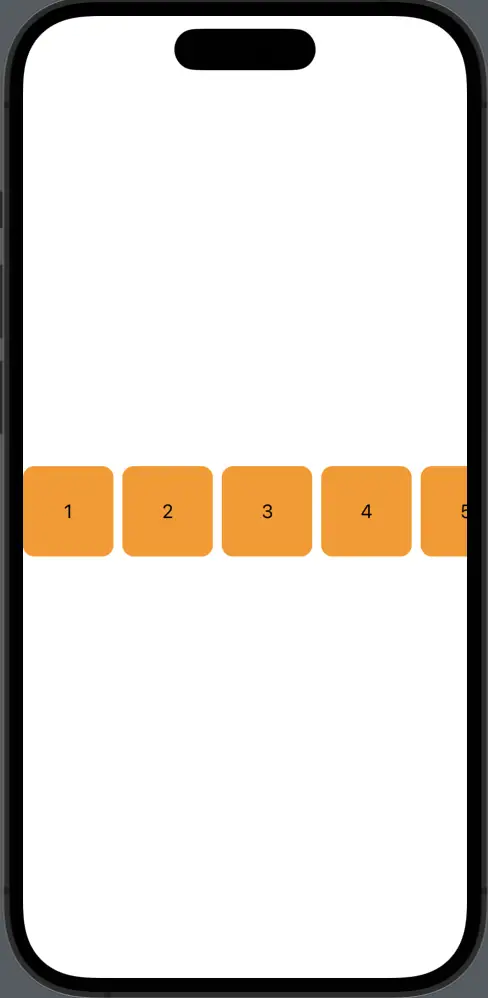
Here, we’ve wrapped the LazyHStack inside a ScrollView to allow horizontal scrolling. The ForEach loop creates a thousand numbered orange boxes. If you were to use a regular HStack here, it would attempt to render all one thousand views at once, which could lead to performance issues.
When to Use LazyHStack?
You should consider using LazyHStack when:
- You want to create a horizontally scrolling view.
- You’re working with a large number of child views that don’t all need to be displayed simultaneously.
- You want to optimize the performance of your app by loading only the visible views.
How to Customize LazyHStack
You can further customize LazyHStack with various modifiers and properties, such as alignment and spacing. For example:
LazyHStack(alignment: .center, spacing: 10) {
// Your views here
}
This will center align the children and add a spacing of 10 points between them.
LazyHStack is a valuable addition to the SwiftUI framework, providing an efficient way to handle horizontal scrolling of large collections of views. It offers the same familiar layout design as HStack but with the added advantage of lazy loading. Utilizing LazyHStack in appropriate scenarios will help in creating more responsive and efficient applications.