How to Change Text Color in iOS SwiftUI
In this blog post, we’re going to dig into an interesting topic for anyone developing with SwiftUI: adjusting text color. This might seem like a simple task, but with the flexibility of SwiftUI, we can do so much more than simply choosing a color from the palette.
Basics: Change Text Color in SwiftUI
First, let’s start with the basics. How do you change the color of text in SwiftUI? The foregroundColor()
modifier is your best friend here. This function changes the color of the text, and it’s as straightforward as it sounds. Here’s a simple example:
Text("Hello, SwiftUI!")
.foregroundColor(.red)
In the example above, we have a text view displaying “Hello, SwiftUI!” in red color.
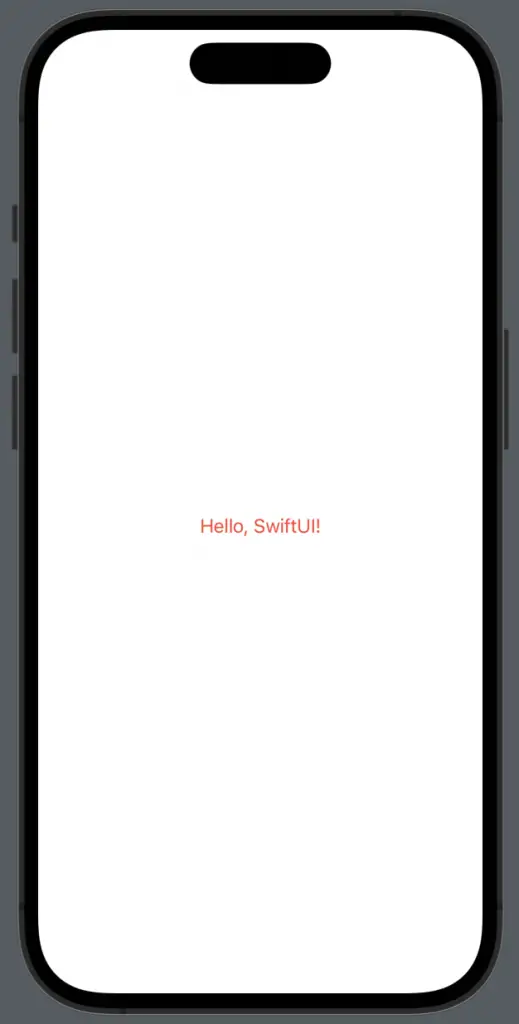
You can use any of the pre-defined colors in SwiftUI such as .blue
, .green
, .yellow
, etc. You can also define your own custom color. Here’s an example:
Text("Hello, SwiftUI!")
.foregroundColor(Color(red: 0.2, green: 0.6, blue: 0.4))
In this example, the text color is a custom color defined by red, green, and blue components.
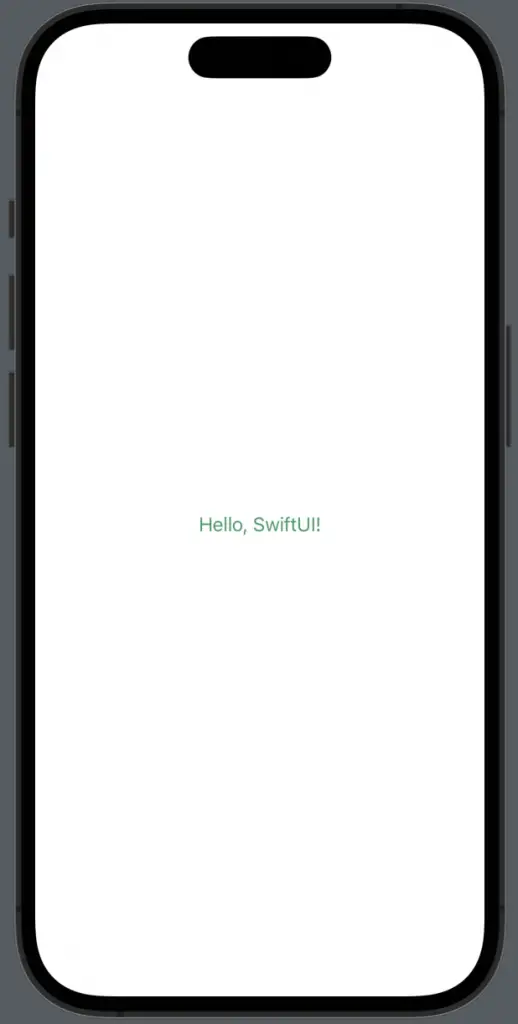
The Power of Environment
One of the key features of SwiftUI is its data-driven approach. You can adjust the text color using the Environment, which allows you to create a common theme across your application.
For instance, you can set a default text color that will be applied across multiple views. Here’s how you can do this:
struct ContentView: View {
var body: some View {
VStack {
Text("Hello, SwiftUI!")
Text("Mastering SwiftUI")
}
.foregroundColor(.purple)
}
}
In the code snippet above, both text views will have purple color, thanks to the applied .foregroundColor()
modifier on their common parent view VStack
.
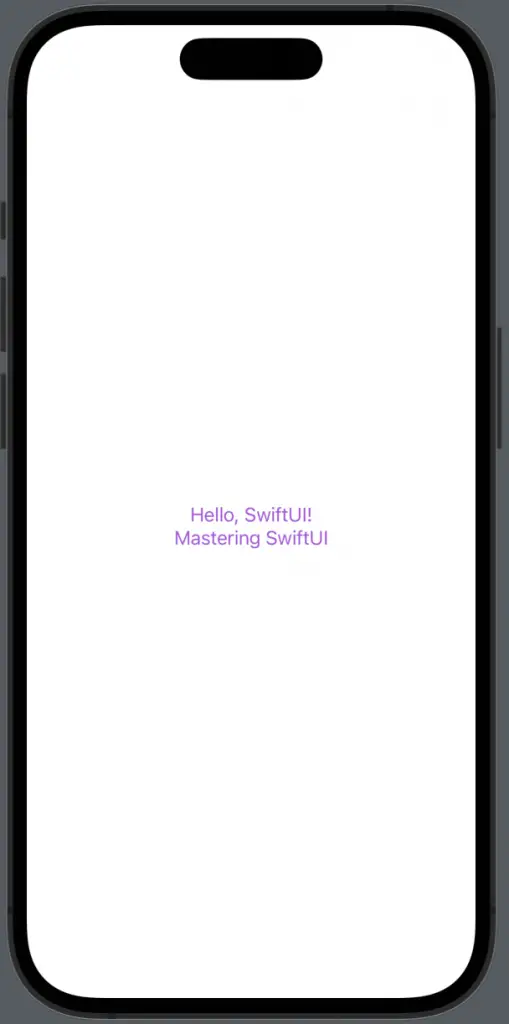
Dynamic Text Color
SwiftUI also supports Dark Mode, a feature that users love. It’s important to ensure that your text color changes appropriately when a user switches between light and dark modes. Thankfully, SwiftUI provides a dynamic color scheme that adapts to the current mode. Here’s how to use it:
Text("Hello, SwiftUI!")
.foregroundColor(Color.primary)
The .primary
color is a dynamic color that changes based on the current color scheme. In light mode, .primary
is black, and in dark mode, it becomes white.
In SwiftUI, adjusting the text color is a simple task, but the range of possibilities it opens up is quite impressive. You can create static color changes, define app-wide color themes, and accommodate user preferences with dynamic color changes.
If you want to change the text background then see this SwiftUI Text background color tutorial.