How to Change Background Color of SwiftUI Segmented Picker
SwiftUI has revolutionized the way we design user interfaces for iOS and macOS. Among its many features, the Segmented Picker stands out for its ease of use and customization.
In this blog post, we’ll focus on how to change the background color of a SwiftUI Segmented Picker.
What is a Segmented Picker?
A Segmented Picker in SwiftUI is a horizontal control made of multiple segments, each functioning as a discrete button. It’s a great way to present a set of mutually exclusive choices side by side.
Why Customize the Background Color?
Customizing the background color of your Segmented Picker can make it blend seamlessly with your app’s design. It can also improve user experience by making the options more readable and interactive.
Steps to Change the Background Color
Create a State Variable
First, you’ll need a state variable to keep track of the selected segment.
@State private var selectedSegment = 0
Add the Segmented Picker
Next, add the Segmented Picker to your SwiftUI view.
Picker("", selection: $selectedSegment) {
Text("First").tag(0)
Text("Second").tag(1)
Text("Third").tag(2)
}
.pickerStyle(SegmentedPickerStyle())
Add the Background Color
To change the background color, you can use the background
modifier.
.background(Color.yellow)
Complete Example
Here’s how everything comes together:
import SwiftUI
struct ContentView: View {
@State private var selectedSegment = 0
var body: some View {
Picker("", selection: $selectedSegment) {
Text("First").tag(0)
Text("Second").tag(1)
Text("Third").tag(2)
}
.pickerStyle(SegmentedPickerStyle())
.background(Color.yellow)
}
}
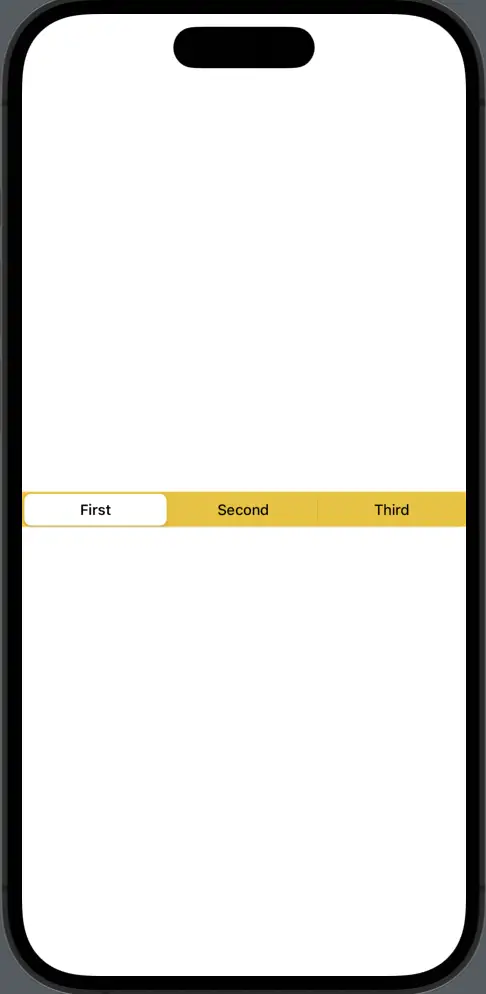
Advanced Customization
For more advanced customization, you can use the .background
modifier with gradients or even images.
import SwiftUI
struct ContentView: View {
@State private var selectedSegment = 0
var body: some View {
Picker("", selection: $selectedSegment) {
Text("First").tag(0)
Text("Second").tag(1)
Text("Third").tag(2)
}
.pickerStyle(SegmentedPickerStyle())
.background(LinearGradient(gradient: Gradient(colors: [Color.red, Color.blue]), startPoint: .leading, endPoint: .trailing))
}
}
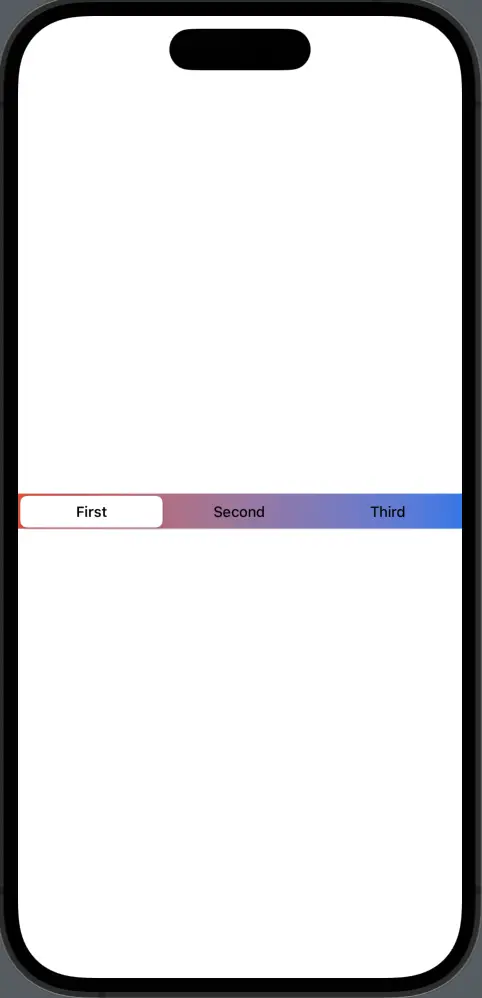
Changing the background color of a SwiftUI Segmented Picker is a simple yet effective way to enhance your app’s UI. With just a few lines of code, you can make your Segmented Picker more visually appealing and user-friendly.