What are Different Picker Styles in iOS SwiftUI
Pickers are essential UI elements that allow users to select from a list of options. SwiftUI offers various styles to customize the appearance and behavior of pickers.
In this blog post, we’ll dive into the different picker styles available in SwiftUI and how to implement them.
DefaultPickerStyle
The DefaultPickerStyle
is the standard style that adapts to the platform it’s running on. Here’s a simple example:
import SwiftUI
struct ContentView: View {
@State private var selectedNumber = 0
var body: some View {
Picker("Choose a number", selection: $selectedNumber) {
ForEach(0..<10) {
Text("\($0)")
}
}
}
}
This will display the picker in the default style for the device you’re using.
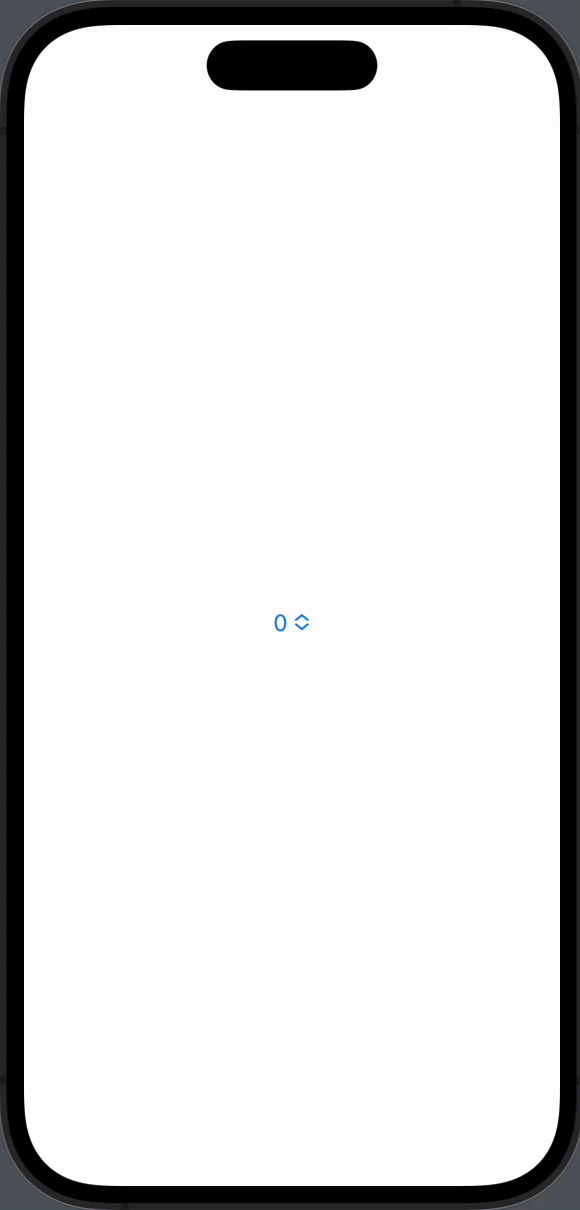
WheelPickerStyle
The WheelPickerStyle
gives a spinning wheel appearance, commonly seen in iOS. To use it, add the .pickerStyle()
modifier:
Picker("Choose a number", selection: $selectedNumber) {
ForEach(0..<10) {
Text("\($0)")
}
}
.pickerStyle(WheelPickerStyle())
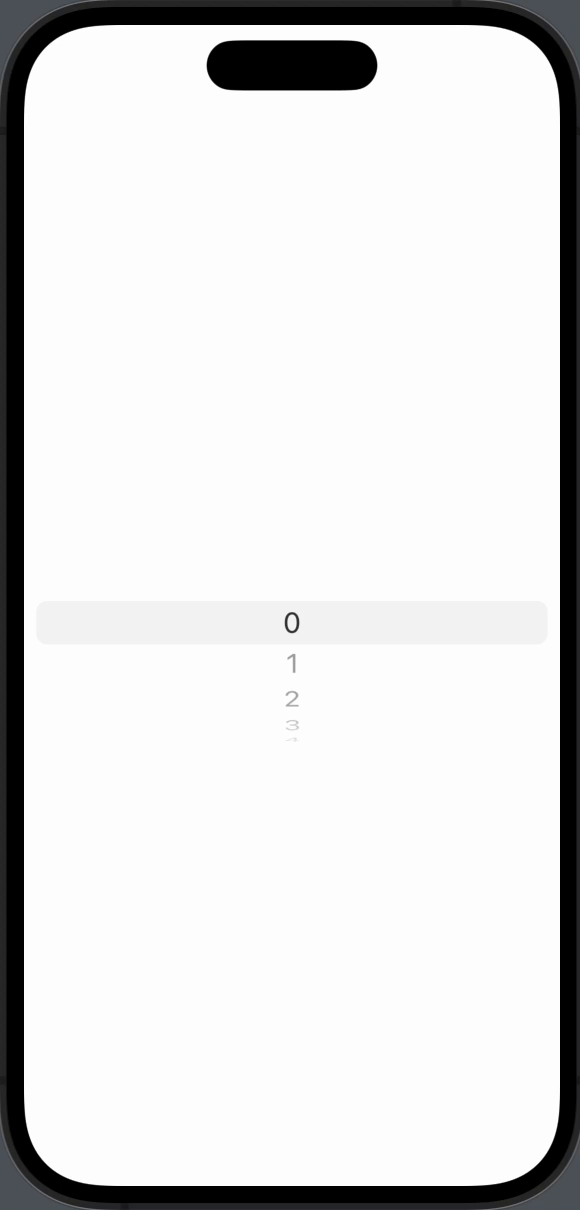
SegmentedPickerStyle
The SegmentedPickerStyle
displays the options as a segmented control. This style is best for a small number of options:
Picker("Choose a number", selection: $selectedNumber) {
ForEach(0..<3) {
Text("\($0)")
}
}
.pickerStyle(SegmentedPickerStyle())
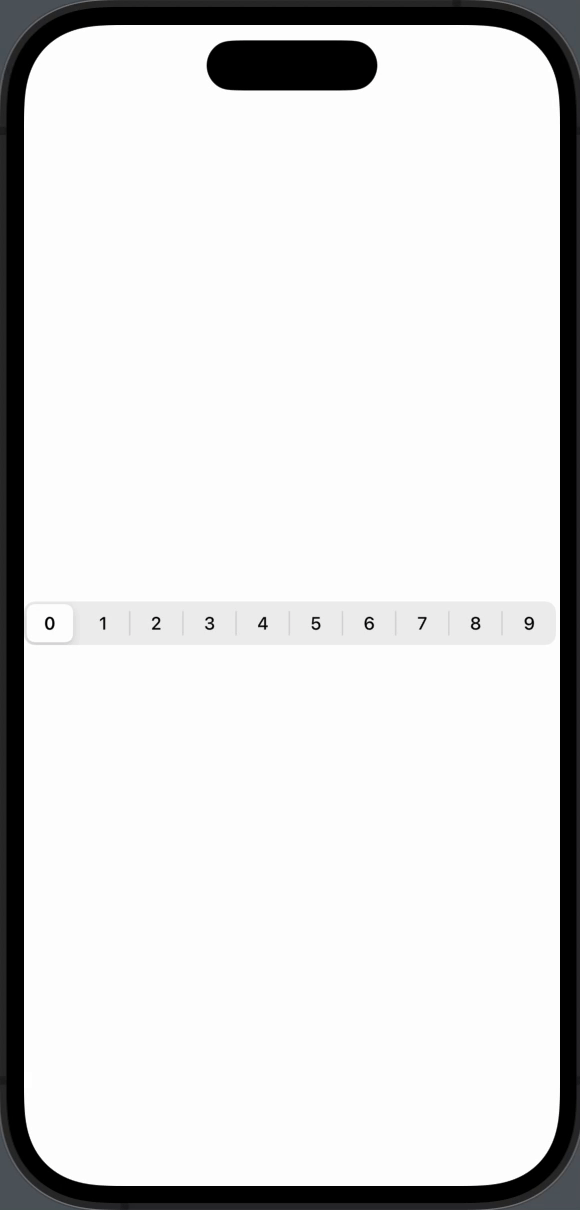
InlinePickerStyle
The InlinePickerStyle
shows the picker inline within the view hierarchy. This is useful when you want to integrate the picker seamlessly:
Picker("Choose a number", selection: $selectedNumber) {
ForEach(0..<10) {
Text("\($0)")
}
}
.pickerStyle(InlinePickerStyle())
When to Use Which Style
- DefaultPickerStyle: When you want the OS to decide.
- WheelPickerStyle: For a classic iOS look.
- SegmentedPickerStyle: For a small set of options.
- InlinePickerStyle: For seamless integration.
SwiftUI provides a variety of picker styles to suit different needs. Whether you want a classic wheel, a segmented control, or an inline picker, SwiftUI has got you covered. Understanding these styles can help you create more intuitive and user-friendly apps.