How to Highlight Specific Words in React Native Text
React Native is a versatile framework that enables you to build mobile apps using JavaScript. Often, you’ll want to highlight certain words in a text string to grab the user’s attention.
In this blog post, we will learn how to highlight specific words within a text string in React Native.
What You Will Need
- Basic understanding of React Native
- A new or existing React Native project
The Example Code
Let’s dive into the example code and understand how it works.
import React from 'react';
import {StyleSheet, View, Text} from 'react-native';
const highlightWords = (string, wordsToHighlight) => {
return string
.split(' ')
.map((word, i) => (
<Text key={i}>
{wordsToHighlight.includes(word.toLowerCase()) ? (
<Text style={styles.highlighted}>{word} </Text>
) : (
`${word} `
)}
</Text>
));
};
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>
{highlightWords(
'Here is a text with some very important words that need to be highlighted',
['important', 'highlighted'],
)}
</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
padding: 24,
justifyContent: 'center',
},
text: {
fontSize: 20,
},
highlighted: {
backgroundColor: 'yellow',
},
});
export default App;
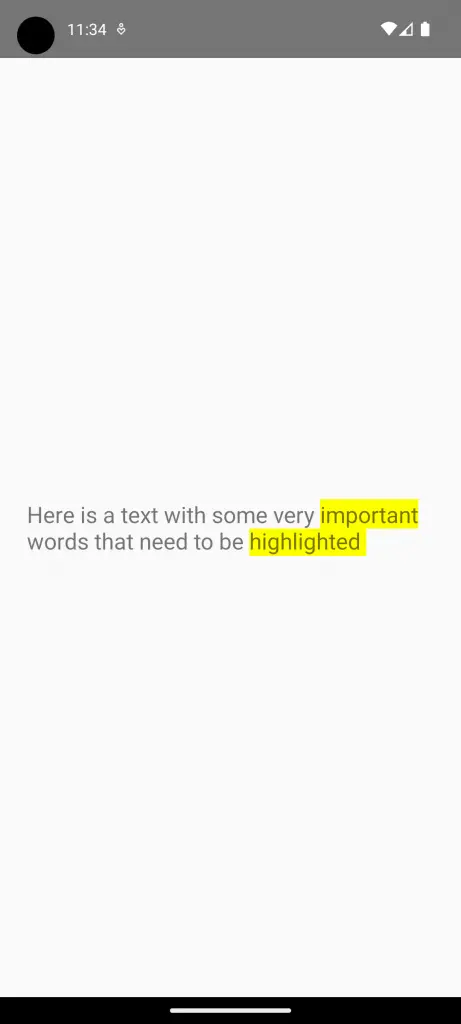
The highlightWords
Function
This function takes in two parameters:
string
: the sentence you want to process.wordsToHighlight
: an array containing the words you want to highlight.
The function splits the string
into an array of words and then maps over these words.
For each word, the function checks whether it exists in the wordsToHighlight
array. If it does, it wraps the word with a Text
component with a highlighted
style. Otherwise, it returns the word as is.
The App
Component
This is the main React Native component. It uses the highlightWords
function to process the text and highlight the words “important” and “highlighted”.
Styling
The styles
object contains three styles:
container
: The main view style.text
: The style for the text in theText
component.highlighted
: The style for the highlighted words, setting the background color to yellow.
The example code offers a straightforward way to highlight specific words in a text string in React Native. It uses the Text
component to selectively apply styles based on the words you want to emphasize. This is particularly useful for drawing attention to key information within an app.
One Comment