How to Add Scroll to Top Functionality to FlatList in React Native
FlatList is one of the most popular components used in react native mobile apps. It helps you to show your data to users in a convenient and scrollable way. A scroll to the top option will be a nice feature for FlatList with so much data. It helps users to return to the top of the list with a single click.
Let’s check how to add this feature to the FlatList. FlatList has so many props and methods. We can make use of scrollToIndex method to scroll to the top. This method accepts parameters such as animated, index, viewOffset, and viewPosition.
Make the value of the index 0 scrolls to the top as the index of the first item in the FlatList is zero.
Let’s create a react native example where I have a FlatList with predefined data. It also has a button at the bottom right. The FlatList scrolls to the top item when the button is pressed. The following example also gives you an idea about how to use scrollToIndex in FlatList.
The following code is in typescript.
import React, {useRef} from 'react';
import {StyleSheet, Pressable, FlatList, Text, View} from 'react-native';
interface Data {
id: number;
title: string;
}
const App = () => {
const flatlistRef = useRef<FlatList<Data>>(null);
const data: Data[] = [
{
id: 1,
title: 'First Item',
},
{
id: 2,
title: 'Second Item',
},
{
id: 3,
title: 'Third Item',
},
{
id: 4,
title: 'Fourth Item',
},
{
id: 5,
title: 'Fifth Item',
},
{
id: 6,
title: 'Sixth Item',
},
{
id: 7,
title: 'Seventh Item',
},
{
id: 8,
title: 'Eighth Item',
},
{
id: 9,
title: 'Ninth Item',
},
{
id: 10,
title: 'Tenth Item',
},
];
const onPressFunction = () => {
flatlistRef.current?.scrollToIndex({index: 0});
};
return (
<View style={styles.container}>
<FlatList
ref={flatlistRef}
data={data}
keyExtractor={item => item.id.toString()}
renderItem={({item}) => <Text style={styles.title}>{item.title}</Text>}
/>
<Pressable style={styles.button} onPress={onPressFunction}>
<Text style={styles.arrow}>^</Text>
</Pressable>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
title: {
fontSize: 72,
},
button: {
position: 'absolute',
width: 50,
height: 50,
borderRadius: 50 / 2,
backgroundColor: 'pink',
alignItems: 'center',
justifyContent: 'center',
right: 30,
bottom: 30,
},
arrow: {
fontSize: 48,
},
});
export default App;
Following is the code for non typescript users.
import React, {useRef} from 'react';
import {StyleSheet, Pressable, FlatList, Text, View} from 'react-native';
const App = () => {
const flatlistRef = useRef();
const data = [
{
id: 1,
title: 'First Item',
},
{
id: 2,
title: 'Second Item',
},
{
id: 3,
title: 'Third Item',
},
{
id: 4,
title: 'Fourth Item',
},
{
id: 5,
title: 'Fifth Item',
},
{
id: 6,
title: 'Sixth Item',
},
{
id: 7,
title: 'Seventh Item',
},
{
id: 8,
title: 'Eighth Item',
},
{
id: 9,
title: 'Ninth Item',
},
{
id: 10,
title: 'Tenth Item',
},
];
const onPressFunction = () => {
flatlistRef.current.scrollToIndex({index: 0});
};
return (
<View style={styles.container}>
<FlatList
ref={flatlistRef}
data={data}
keyExtractor={(item) => item.id}
renderItem={({item}) => <Text style={styles.title}>{item.title}</Text>}
/>
<Pressable style={styles.button} onPress={onPressFunction}>
<Text style={styles.arrow}>^</Text>
</Pressable>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
title: {
fontSize: 72,
},
button: {
position: 'absolute',
width: 50,
height: 50,
borderRadius: 50 / 2,
backgroundColor: 'pink',
alignItems: 'center',
justifyContent: 'center',
right: 30,
bottom: 30,
},
arrow: {
fontSize: 48,
},
});
export default App;
As you see in the code, when the Pressable component is pressed we invoke the scrollToIndex method with the help of the useRef hook.
Following is the output of the above example.
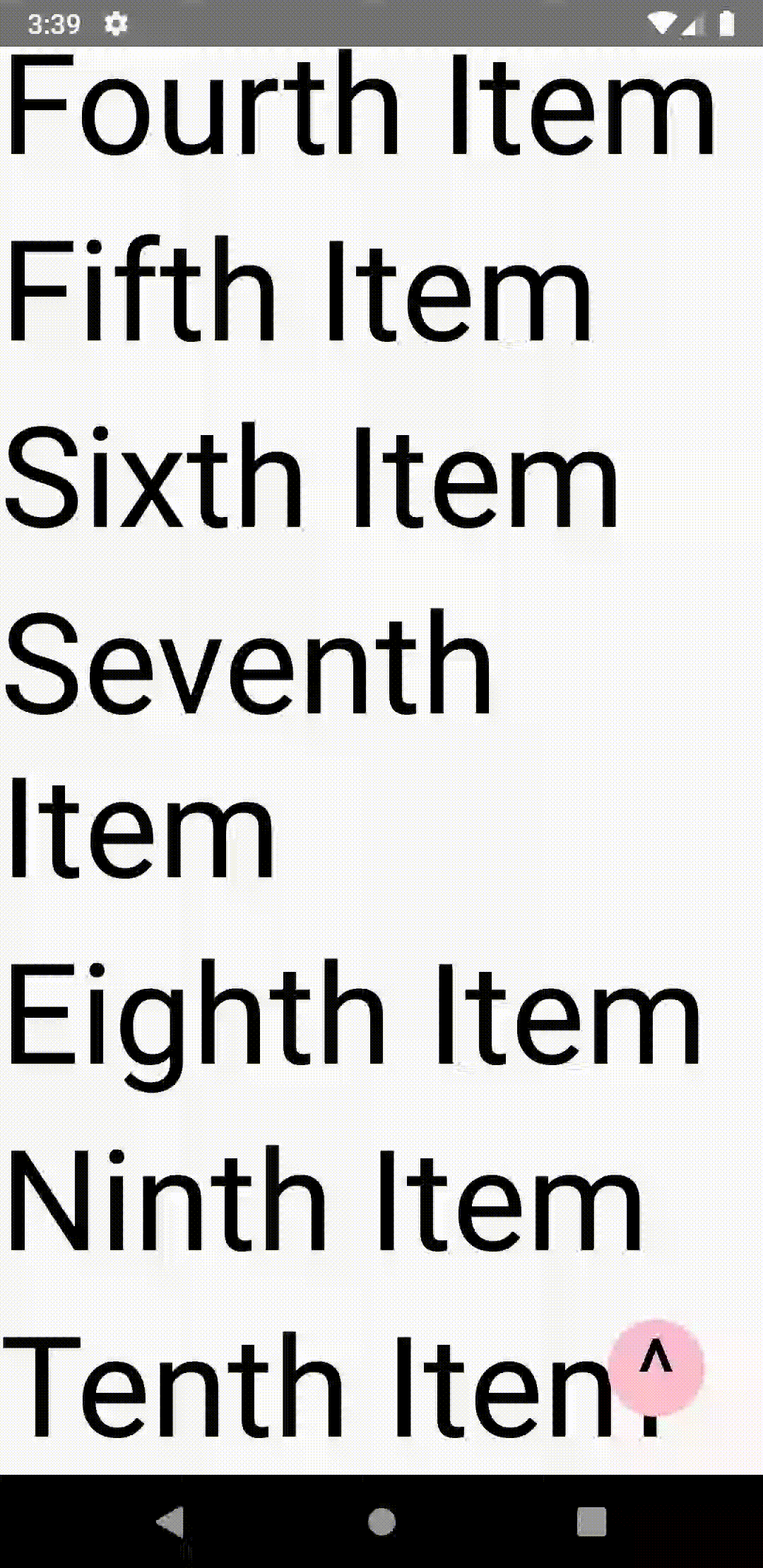
That’s how you add the scroll to the top feature to your react native FlatList component.
One Comment