How to Add Scroll to Bottom Functionality to FlatList in React Native
Everyone knows the importance of the FlatList component in react native apps. FlatList helps us to huge amounts of data in the most user-friendly way. In this blog post, let’s check how to add a scroll to bottom functionality to FlatList.
If you are looking for how to add the scroll to top option in FlatList then you may click here.
The FlatList has so many useful props and methods. We should make use of scrollToEnd method. It accepts parameters such as animated.
We have a FlatList and Pressable component In the following react native scroll to bottom example. When the button is pressed we invoke the scrolltoEnd method of FlatList using the useRef hook. Go through the code given below.
Following is the typescript code for FlatList scroll to the bottom.
import React, {useRef} from 'react';
import {StyleSheet, Pressable, FlatList, Text, View} from 'react-native';
interface Data {
id: string;
title: string;
}
const App = () => {
const flatlistRef = useRef<FlatList<Data>>(null);
const data: Data[] = [
{
id: '1',
title: 'First Item',
},
{
id: '2',
title: 'Second Item',
},
{
id: '3',
title: 'Third Item',
},
{
id: '4',
title: 'Fourth Item',
},
{
id: '5',
title: 'Fifth Item',
},
{
id: '6',
title: 'Sixth Item',
},
{
id: '7',
title: 'Seventh Item',
},
{
id: '8',
title: 'Eighth Item',
},
{
id: '9',
title: 'Ninth Item',
},
{
id: '10',
title: 'Tenth Item',
},
];
const onPressFunction = () => {
flatlistRef.current?.scrollToEnd();
};
return (
<View style={styles.container}>
<FlatList
ref={flatlistRef}
data={data}
keyExtractor={item => item.id}
renderItem={({item}) => <Text style={styles.title}>{item.title}</Text>}
/>
<Pressable style={styles.button} onPress={onPressFunction}>
<Text style={styles.arrow}>v</Text>
</Pressable>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
title: {
fontSize: 72,
},
button: {
position: 'absolute',
width: 50,
height: 50,
borderRadius: 50 / 2,
backgroundColor: 'pink',
alignItems: 'center',
justifyContent: 'center',
right: 30,
bottom: 30,
},
arrow: {
fontSize: 36,
},
});
export default App;
Following is the code for non typescript users.
import React, {useRef} from 'react';
import {StyleSheet, Pressable, FlatList, Text, View} from 'react-native';
const App = () => {
const flatlistRef = useRef();
const data = [
{
id: '1',
title: 'First Item',
},
{
id: '2',
title: 'Second Item',
},
{
id: '3',
title: 'Third Item',
},
{
id: '4',
title: 'Fourth Item',
},
{
id: '5',
title: 'Fifth Item',
},
{
id: '6',
title: 'Sixth Item',
},
{
id: '7',
title: 'Seventh Item',
},
{
id: '8',
title: 'Eighth Item',
},
{
id: '9',
title: 'Ninth Item',
},
{
id: '10',
title: 'Tenth Item',
},
];
const onPressFunction = () => {
flatlistRef.current.scrollToEnd();
};
return (
<View style={styles.container}>
<FlatList
ref={flatlistRef}
data={data}
keyExtractor={(item) => item.id}
renderItem={({item}) => <Text style={styles.title}>{item.title}</Text>}
/>
<Pressable style={styles.button} onPress={onPressFunction}>
<Text style={styles.arrow}>v</Text>
</Pressable>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
title: {
fontSize: 72,
},
button: {
position: 'absolute',
width: 50,
height: 50,
borderRadius: 50 / 2,
backgroundColor: 'pink',
alignItems: 'center',
justifyContent: 'center',
right: 30,
bottom: 30,
},
arrow: {
fontSize: 36,
},
});
export default App;
Following is the output of this react native tutorial.
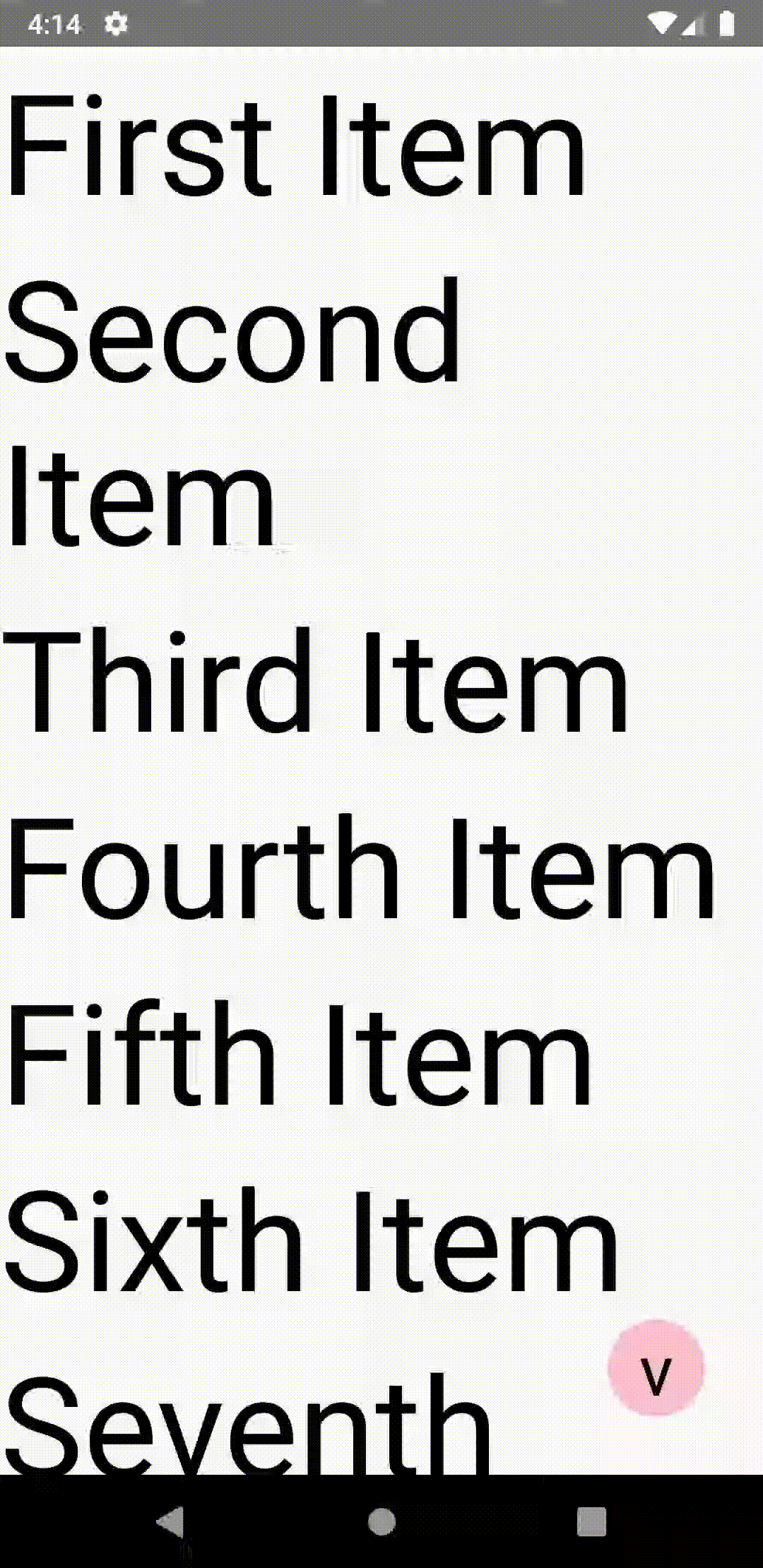
That’s it. Thank you for reading!