How to use useRef Hook in React Native Functional Component
Hooks are an integral part of a functional component. The useRef hook is used to invoke methods on react native components. In this blog post, let’s check how to use useRef in react native.
We have a TextInput component and a Pressable component. When we press the Pressable component, the text inside TextInput should be cleared by invoking clear method. If you are new to functional components in react native, then please go through this blog post before proceeding.
We use the useRef hook to invoke a clear method as given in the following react native example.
import React, {useState, useRef} from 'react';
import {TextInput, View, Pressable, Text} from 'react-native';
const App = () => {
const inputRef = useRef();
const [input, setInput] = useState('');
const pressed = () => {
inputRef.current.clear();
};
return (
<View style={{flex: 1}}>
<TextInput
ref={inputRef}
style={{height: 40, borderColor: 'gray', borderWidth: 1}}
onChangeText={(text) => setInput(text)}
value={input}
/>
<Pressable onPress={pressed}>
<Text>Clear it!</Text>
</Pressable>
</View>
);
};
export default App;
The output of the above example is given below.
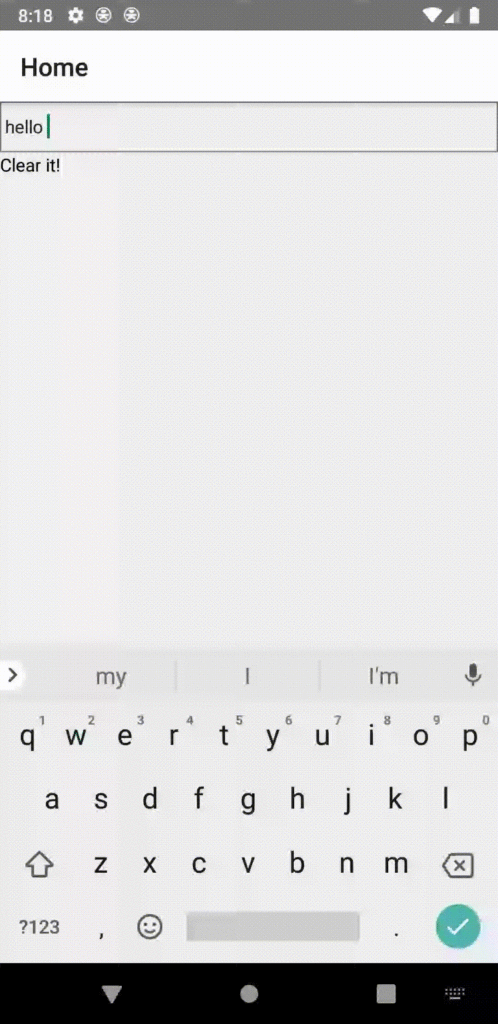
I hope this tutorial has helped you!
Thanks Rashid for all your tutorials. Kindly come up with a tutorial on authentication useReducer with an API call inclusive. Regards
Thanks Rashid for all your tutorials. Kindly come up with a tutorial on authentication useReducer with an API call inclusive. Regards