How to Add Shadows to Icon in React Native
Shadows can add depth and dimension to flat icons, making them look more appealing and professional. In this blog post, let’s learn how to add a shadow to an icon in react native.
We will be using react native vector icons library for this tutorial. I suggest you to read how to use react native vector icons blog post to install and set up the library.
As the vector icons are basically fonts you can apply text style properties to the icon. See the following code.
{
shadowOpacity: 2,
textShadowRadius: 2,
textShadowOffset: {width: 2, height: 2},
}
The style includes properties that control the opacity of the shadow, the size of the shadow, and the position of the shadow relative to the text.
The shadow opacity is set to 2, which means it will be slightly opaque. The shadow radius is set to 2, making it relatively small. The shadow offset is defined by the width and height properties, which are both set to 2, causing the shadow to be slightly offset from the text in both directions.
The resulting effect is a slightly opaque, small shadow that adds depth and dimension to the icon.
Following is the complete code.
import {View} from 'react-native';
import React from 'react';
import Icon from 'react-native-vector-icons/MaterialCommunityIcons';
function App() {
return (
<View style={{flex: 1, justifyContent: 'center', alignItems: 'center'}}>
<Icon
name="account-circle"
size={75}
color="red"
style={{
shadowOpacity: 2,
textShadowRadius: 2,
textShadowOffset: {width: 2, height: 2},
}}
/>
</View>
);
}
export default App;
You will get the following output on Android.
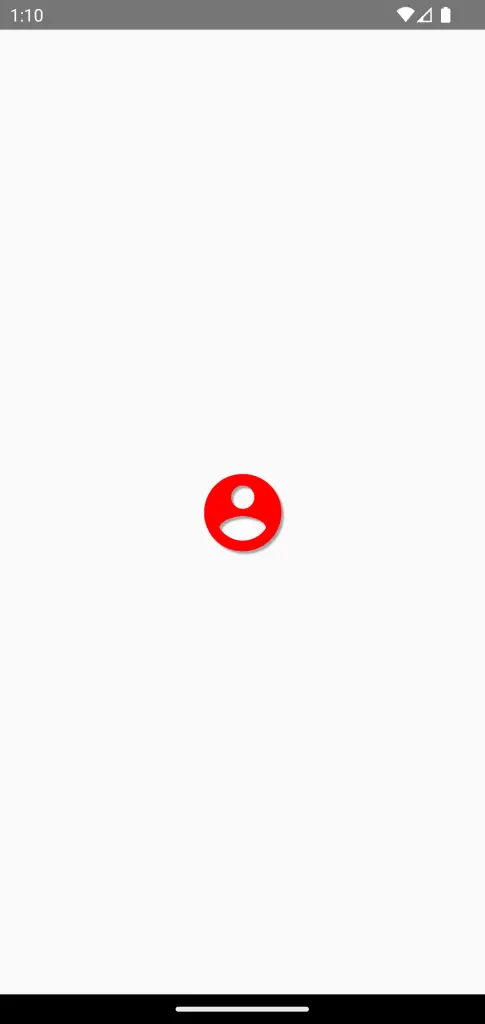
And following output on iOS.
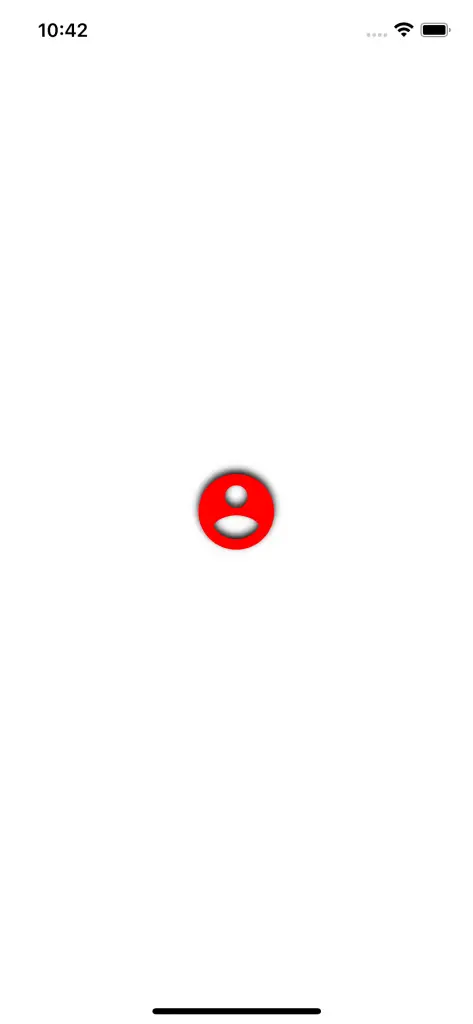
As you see, there are some differences in the appearance of shadows in Android and iOS. If you want a consistent look, it’s always a good idea to write platform specific style for both platforms.