How to Capitalize TextInput Component in React Native
TextInput is the component where the end user gives input. Sometimes, we may need to control how the values are inserted into the TextInput. Let’s check how to capitalize the text inserted through TextInput in react native.
If you are looking for how to capitalize a Text component in react native then you can check this blog post.
The textInput component has a prop named autoCapitalize. This prop can be used to capitalize the input.
In the following react native example, I am capitalizing the whole input using autoCapitalize prop.
import React, {useState} from 'react';
import {TextInput} from 'react-native';
const App = () => {
const [input, setInput] = useState('');
return (
<TextInput
style={{height: 40, borderColor: 'gray', borderWidth: 1}}
autoCapitalize="characters"
onChangeText={(text) => setInput(text)}
value={input}
/>
);
};
export default App;
See the output below.
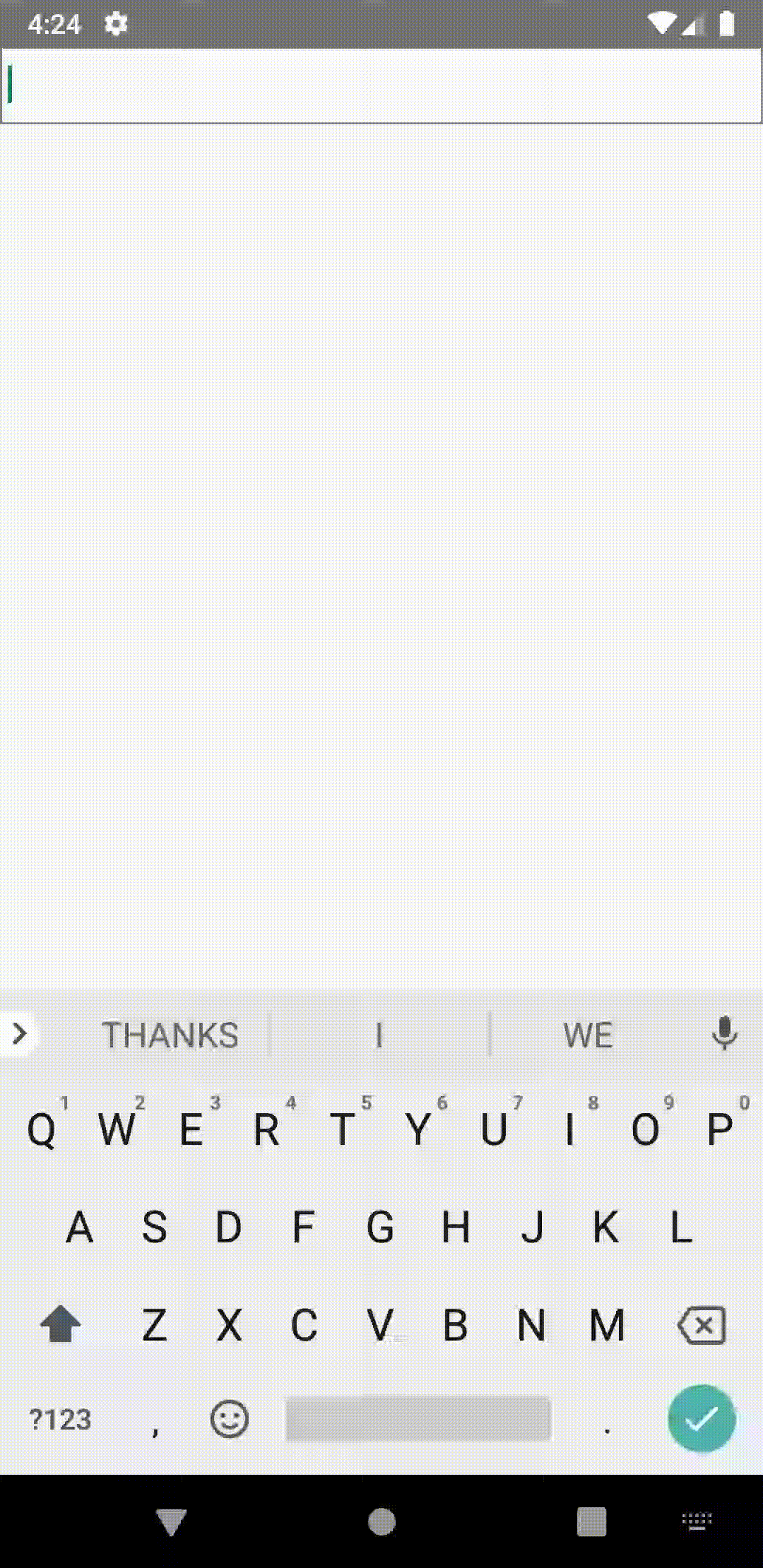
As you noticed, the value of autoCapitalize prop is “characters” in the above example. This made all the text capitalize. If you want to capitalize the first letter of each word then use the value “words”. The value “sentences” can be used when you want to capitalize the first letter of each sentence. For more information on autoCapitalize prop, you can go here.
You should note that autoCapitalize prop is not supported by some keyboard types such as name-phone-pad. I hope this blog post will help you to capitalize easily with the TextInput component in react native.