How to Display PDF files in React Native
The Portable Document Format (PDF) is one of the most popular formats used for documents. In this blog post, let’s check how to show pdf files in react native.
React Native Pdf is a third party that helps us to open and read pdf files from a URL or local assets in react native. It has important features such as drag and zoom, password-protected pdf support, etc. In short, you can create a pdf viewer with this library.
To make React Native Pdf work you also need another library react native blob util. Install both libraries using the command given below.
npm install react-native-pdf react-native-blob-util --save
Also, don’t forget to run the pod install command inside the ios folder.
On Android, open android/app/build.gradle and add the following inside the android {} tag.
packagingOptions {
pickFirst 'lib/x86/libc++_shared.so'
pickFirst 'lib/x86_64/libjsc.so'
pickFirst 'lib/arm64-v8a/libjsc.so'
pickFirst 'lib/arm64-v8a/libc++_shared.so'
pickFirst 'lib/x86_64/libc++_shared.so'
pickFirst 'lib/armeabi-v7a/libc++_shared.so'
}
Now, you can create a simple pdf viewer in react native as given below.
import React from 'react';
import {StyleSheet, Dimensions, View, Platform} from 'react-native';
import Pdf from 'react-native-pdf';
const source = {
uri: 'https://www.africau.edu/images/default/sample.pdf',
cache: true,
};
const App = () => {
return (
<View style={styles.container}>
<Pdf
source={source}
trustAllCerts={Platform.OS === 'ios'}
onLoadComplete={(numberOfPages, filePath) => {
console.log(`number of pages: ${numberOfPages}`);
}}
onPageChanged={(page, numberOfPages) => {
console.log(`current page: ${page}`);
}}
onError={error => {
console.log(error);
}}
onPressLink={uri => {
console.log(`Link presse: ${uri}`);
}}
style={styles.pdf}
/>
</View>
);
};
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'flex-start',
alignItems: 'center',
marginTop: 25,
},
pdf: {
flex: 1,
width: Dimensions.get('window').width,
height: Dimensions.get('window').height,
},
});
This ‘Pdf’ component displays the PDF file and provides callbacks for various events, such as when the PDF file is completely loaded, when a page is changed, when an error occurs, and when a link is pressed.
The output of this react native pdf viewer example on Android is as given below.
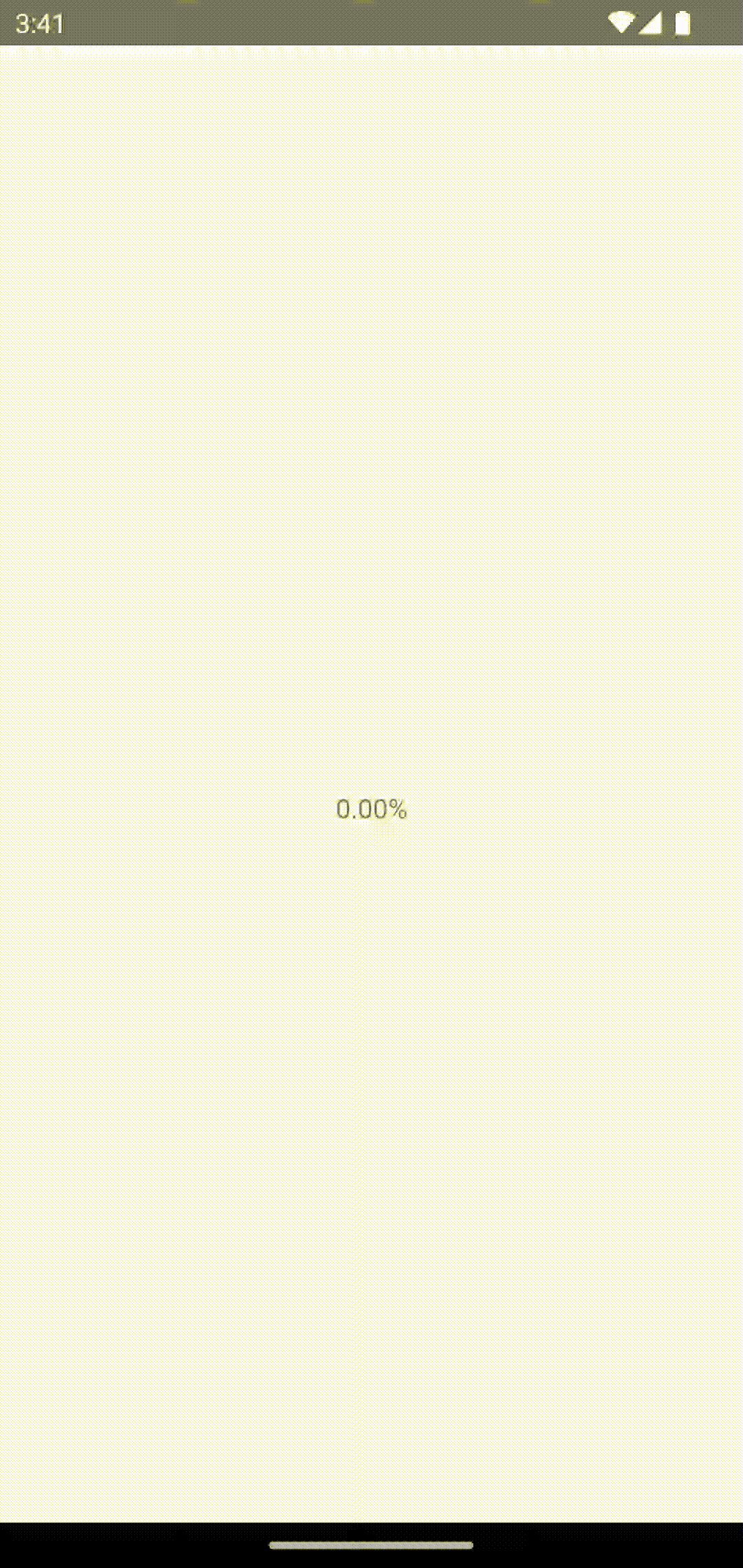
Following is the output in iOS.
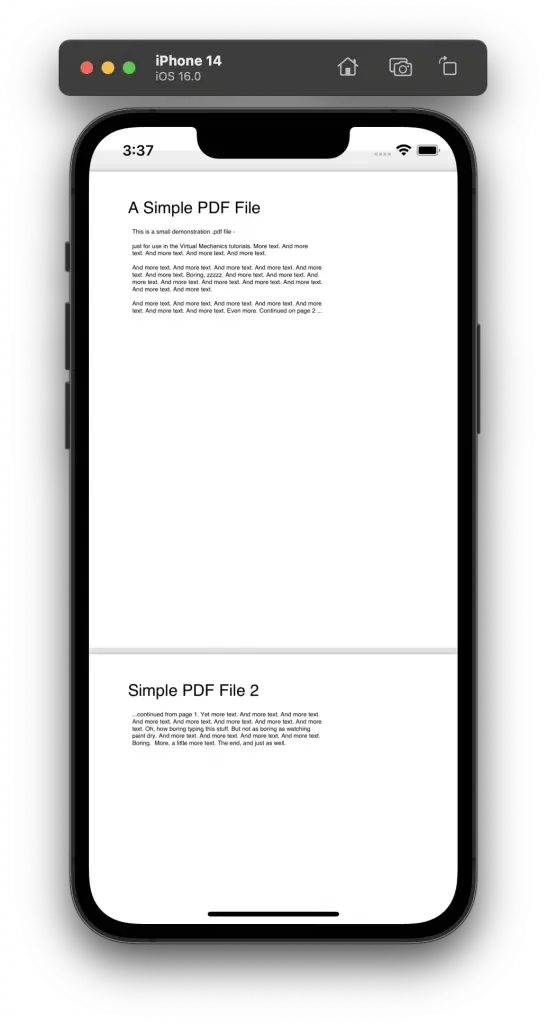
This is just a basic example and you can go through the official documentation of react native pdf library to do advanced things.
2 Comments