React Navigation: How to Hide Header in React Native
In this blog post, let’s learn how to customize the header in React Navigation to hide it from screens. This is a common requirement for many React Native apps.
Here, I am not covering how to install and use react navigation. For that, you can check out my blog post here.
We can hide the header by setting the headerShown prop as false. The following example hides the header for a specific screen only.
import * as React from 'react';
import {NavigationContainer} from '@react-navigation/native';
import {createStackNavigator} from '@react-navigation/stack';
import FirstScreen from './FirstScreen';
import SecondScreen from './SecondScreen';
const Stack = createStackNavigator();
const App = () => {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen
name="FirstScreen"
component={FirstScreen}
options={{headerShown: false}}
/>
<Stack.Screen name="SecondScreen" component={SecondScreen} />
</Stack.Navigator>
</NavigationContainer>
);
};
export default App;
Following is the output where the header of the first screen is hidden.
Do you want to hide the header of a whole Stack Navigator? Then you should use both screenOptions and headerShown as given below.
import * as React from 'react';
import {NavigationContainer} from '@react-navigation/native';
import {createStackNavigator} from '@react-navigation/stack';
import FirstScreen from './FirstScreen';
import SecondScreen from './SecondScreen';
const Stack = createStackNavigator();
const App = () => {
return (
<NavigationContainer headerMode="none">
<Stack.Navigator
screenOptions={{
headerShown: false,
}}>
<Stack.Screen name="FirstScreen" component={FirstScreen} />
<Stack.Screen name="SecondScreen" component={SecondScreen} />
</Stack.Navigator>
</NavigationContainer>
);
};
export default App;
Now, see the output where the headers of all the screens are hidden.
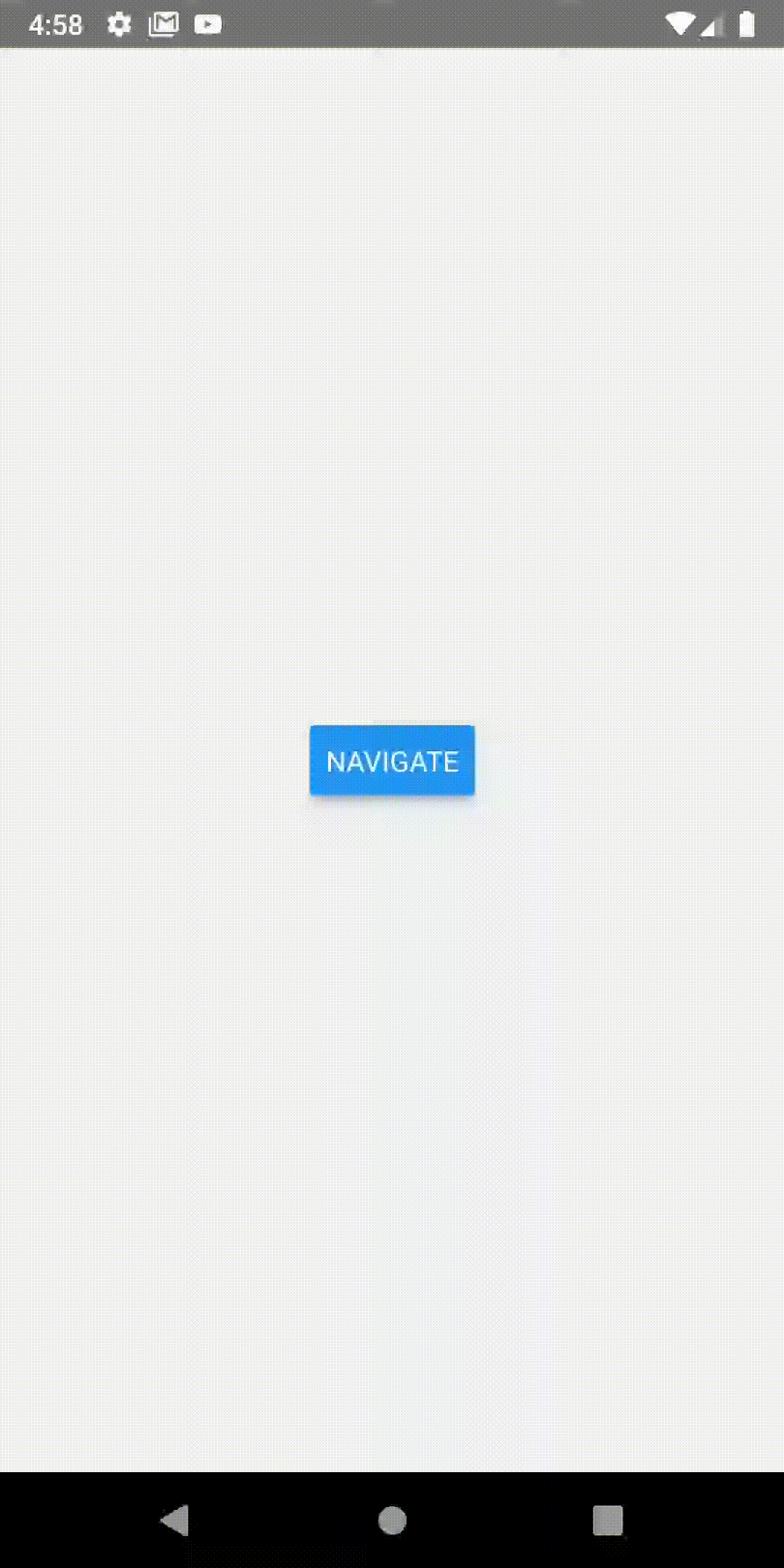
That’s it. I hope you find this react native navigation tutorial to hide the header helpful.
thank you
thank you