How to add Image with Rounded Corners in React Native
In this blog post, let’s learn how to add an image with rounded corners in react native. We use the Image component and the borderRadius property to create rounded corners for the image.
See the following code snippet.
<Image source={cat} style={{width: 250,
height: 250,
borderRadius: 50}} />
You will get the following output.
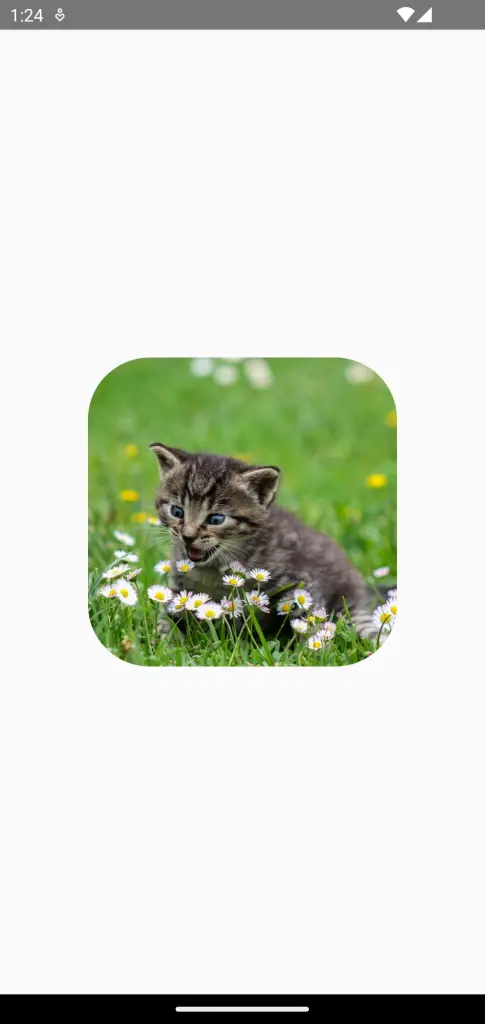
Following is the complete code for reference.
import React from 'react';
import {Image, View, StyleSheet} from 'react-native';
import cat from './cat.jpg';
const App = () => {
return (
<View style={styles.container}>
<Image source={cat} style={styles.image} />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
image: {
width: 250,
height: 250,
borderRadius: 50,
},
});
export default App;
Using the borderRadius style property you can even create round, circular images.
That’s how you add an image with rounded corners in react native.