How to Request Permissions for iOS in React Native
In order to access the user’s location, camera, contacts, etc., you need to request and handle the user’s response to these permissions in Android and iOS. In this blog post, let’s learn how to request iOS permissions in react native.
There’s no inbuilt API to request iOS permissions in react native. Hence, I am using react native permissions library for this tutorial where I request permission to access contact.
First of all, install the react native permission using any of the following commands.
npm install --save react-native-permissions
or
yarn add react-native-permissions
Then open Podfile and add permission handler. As here I want contact permission I am just adding that.
target 'example' do
permissions_path = '../node_modules/react-native-permissions/ios'
pod 'Permission-Contacts', :path => "#{permissions_path}/Contacts"
Then run the pod install command from the ios folder. After that add permission details in Info.plist file.
key>NSContactsUsageDescription</key>
<string>This app needs contact details for proper functioning!</string>
Now, you can request permission as given below.
import {StyleSheet, Button, View} from 'react-native';
import React from 'react';
import {request, PERMISSIONS} from 'react-native-permissions';
const App = () => {
const requestPermission = () => {
request(PERMISSIONS.IOS.CONTACTS).then(result => {
console.log(result);
});
};
return (
<View style={styles.container}>
<Button onPress={requestPermission} title="Download" />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
export default App;
Here the request appears when the button is pressed. The following will be the output.
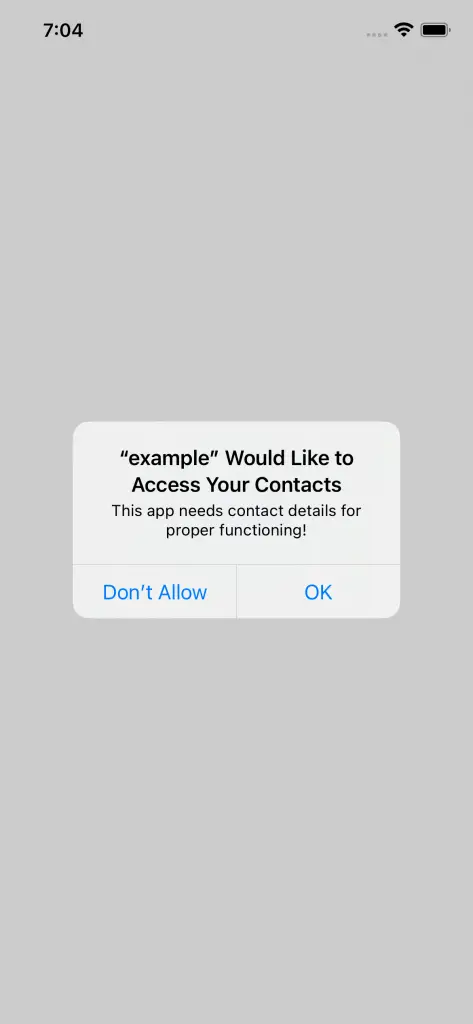
That’s how you ask iOS permissions in react native.