How to Change TextInput Font in React Native
A great user interface is essential for engaging app experiences, and fonts play a big role in that. In React Native, the TextInput
component allows easy text input but defaults to the system font. This blog post will teach you how to change the font in TextInput
with examples and explanations.
Prerequisites
- A working React Native development environment
- Basic understanding of React Native and JavaScript
Set up a Basic TextInput
Firstly, let’s start by creating a simple TextInput
.
import React from 'react';
import { View, TextInput } from 'react-native';
const App = () => {
return (
<View>
<TextInput placeholder="Default Font" />
</View>
);
};
export default App;
Change Font Using Inline Style
You can directly specify a font family for your TextInput
using inline style.
<TextInput
placeholder="Custom Font"
style={{ fontFamily: 'monospace' }}
/>
The style
prop allows you to define custom styles. The fontFamily
sets the desired font.
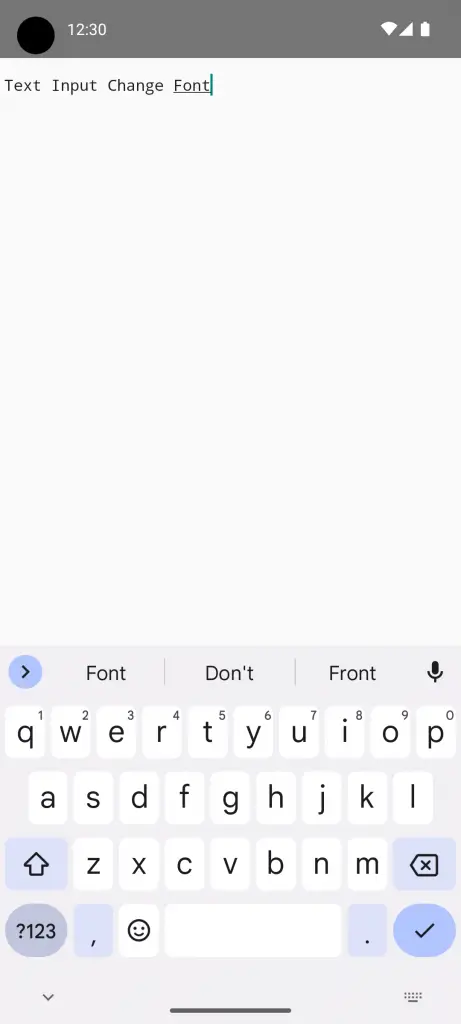
Use StyleSheet for Scalability
Using inline styles might not be practical for larger apps. StyleSheet
can make your code cleaner and more manageable.
import { StyleSheet } from 'react-native';
const styles = StyleSheet.create({
customFontInput: {
fontFamily: 'Courier New',
},
});
// In the component
<TextInput
placeholder="Custom Font"
style={styles.customFontInput}
/>
The StyleSheet.create
function organizes your styles. The customFontInput
style includes the fontFamily
property.
Conclusion
Changing the font in React Native’s TextInput
is a straightforward process but adds a polished look and feel to your app. Whether you use built-in fonts or custom ones, setting them up is relatively simple and goes a long way in enhancing the user interface.