How to Show Static HTML in React Native Webview
You can show static HTML in your React Native app using the WebView component. The WebView component is not available in react native core but you can get it from react native community.
Firstly, install react native webview using any of the following commands.
npm install --save react-native-webview
or
yarn add react-native-webview
The iOS users don’t forget to run pod install inside the ios folder.
You can show the static HTML by passing it to the source prop of the WebView component.
<WebView
source={{
html: '<h1>Hello world</h1>'
}}
Following is the complete example.
import React from 'react';
import {View} from 'react-native';
import WebView from 'react-native-webview';
const App = () => {
return (
<View style={{flex: 1}}>
<WebView
originWhitelist={['*']}
scalesPageToFit={false}
source={{
html: `
<body>
<h1>My First Heading</h1>
<p>My first paragraph.</p>
</body>`,
}}
/>
</View>
);
};
export default App;
Following is the output of the above react native webview example.
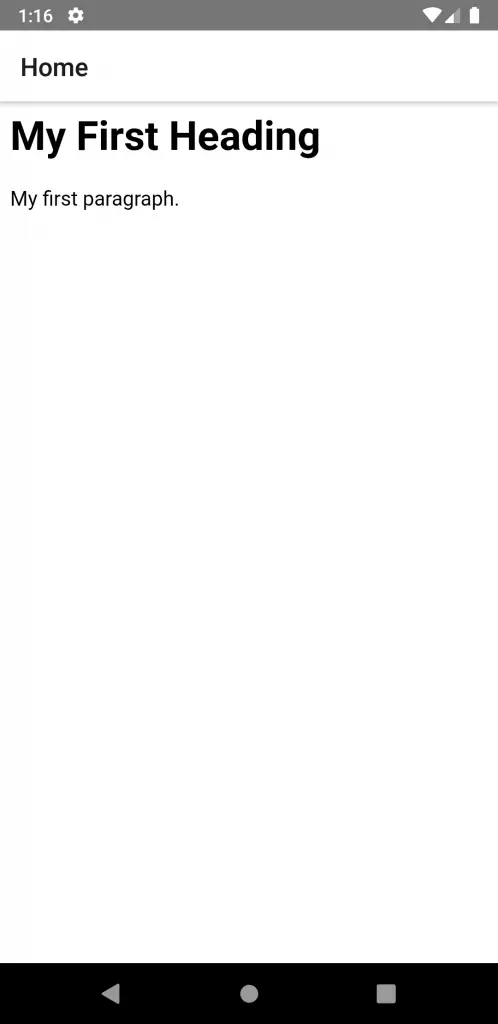
That’s how you show HTML in react native using WebView.