How to Create Toast in React Native (Android)
If you are an Android app developer then you might already know what a toast is and how to use it in Android apps. You can create Toasts in React Native also.
You need to use ToastAndroid API to create toast in React Native. You can check out the official document of ToastAndroid here. We use the show method of ToastAndroid to show a predefined message and duration.
The following example shows the basic usage of toast in react native. The toast is shown when the Button is pressed.
import React from 'react';
import {ToastAndroid, Button, View} from 'react-native';
const App = () => {
return (
<View>
<Button
title="click to show toast"
onPress={() => {
ToastAndroid.show(
'Toast message with long duration',
ToastAndroid.LONG,
);
}}
/>
</View>
);
};
export default App;
Following is the output of the example.
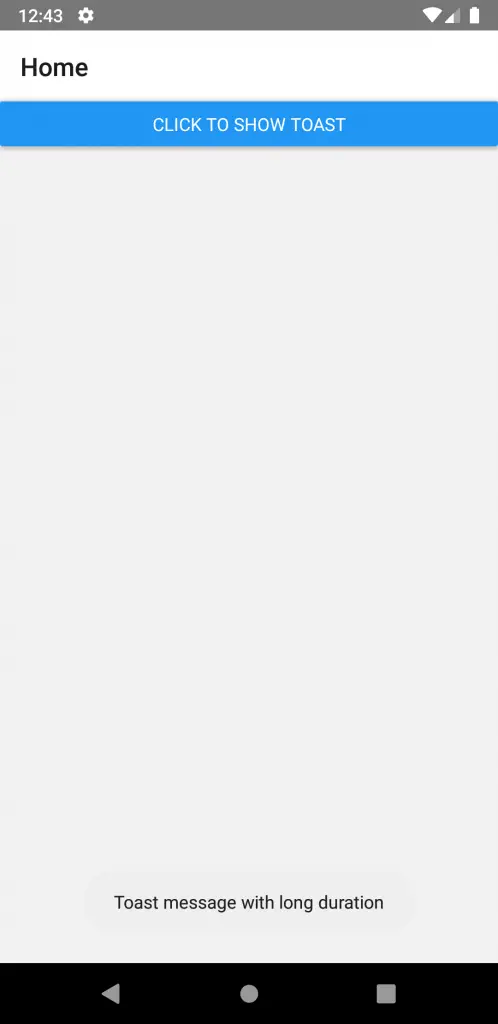
That’s how you show Toasts in react native.