How to Add Underline to Text in React Native
You’ve built a React Native app and now you want to style your text. One classic way to make important text stand out is by underlining it. In this blog post, we’ll go through the different ways you can underline text in React Native.
Using Inline Styles
The easiest way to add underline to text is using inline styles. Here’s a simple example:
import {Text, View} from 'react-native';
import React from 'react';
const App = () => {
return (
<View>
<Text style={{textDecorationLine: 'underline'}}>
This is an underlined text
</Text>
</View>
);
};
export default App;
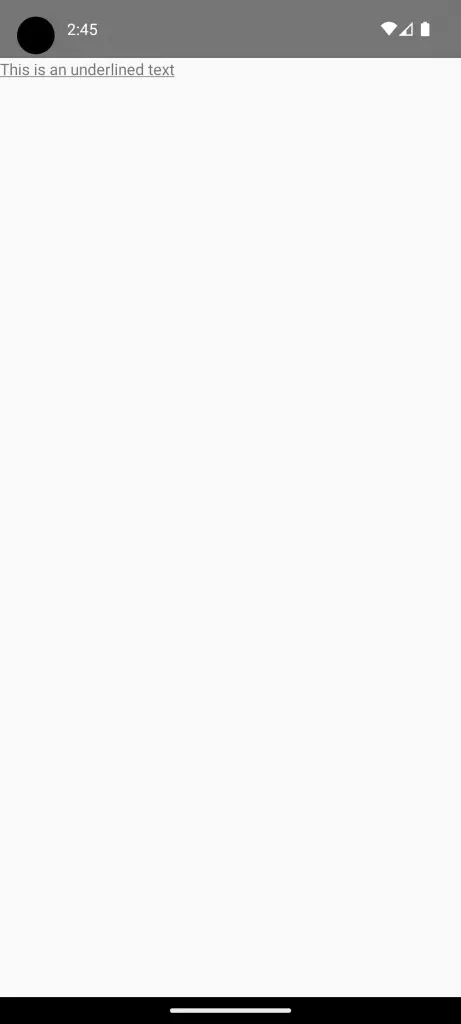
Using StyleSheet
Another option is to use StyleSheet
. This is helpful if you want to reuse the style.
import {StyleSheet, Text, View} from 'react-native';
import React from 'react';
const App = () => {
return (
<View>
<Text style={styles.underlinedText}>This is an underlined text</Text>
</View>
);
};
export default App;
const styles = StyleSheet.create({
underlinedText: {
textDecorationLine: 'underline',
},
});
Combine with Other Text Decorations
You can also combine underline with other text decorations like line-through
.
import {Text, View} from 'react-native';
import React from 'react';
const App = () => {
return (
<View>
<Text style={{textDecorationLine: 'underline line-through'}}>
Underlined and Strike Through
</Text>
</View>
);
};
export default App;
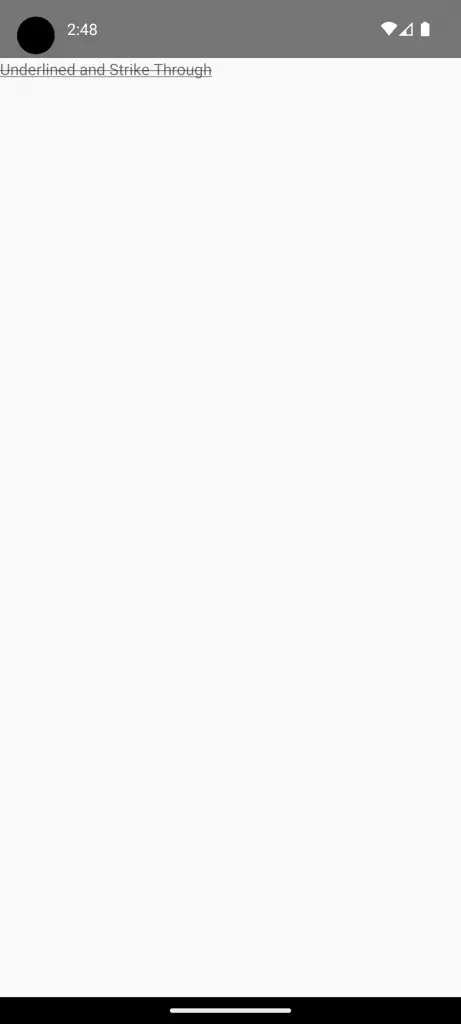
Underlining text in React Native can be done in several ways, from simple inline styles to more customized solutions using View
. Choose the method that best fits your project’s needs.