How to Create Bullet Points in React Native Text
Bullet points are an excellent way to organize and display information in a clean format. While React Native doesn’t offer a built-in feature for bullet points, you can still create them in creative ways. This blog post explores multiple techniques to add bullet points to your React Native app.
Prerequisites
- A functional React Native environment
- Basic knowledge of React Native and JavaScript
Method 1: Using Unicode Characters
One easy way to add bullet points is by using Unicode characters. You can manually copy paste a bullet symbol (•
) before each item in your list.
import React from 'react';
import {View, Text} from 'react-native';
const App = () => {
return (
<View>
<Text> • Item 1</Text>
<Text> • Item 2</Text>
<Text> • Item 3</Text>
</View>
);
};
export default App;
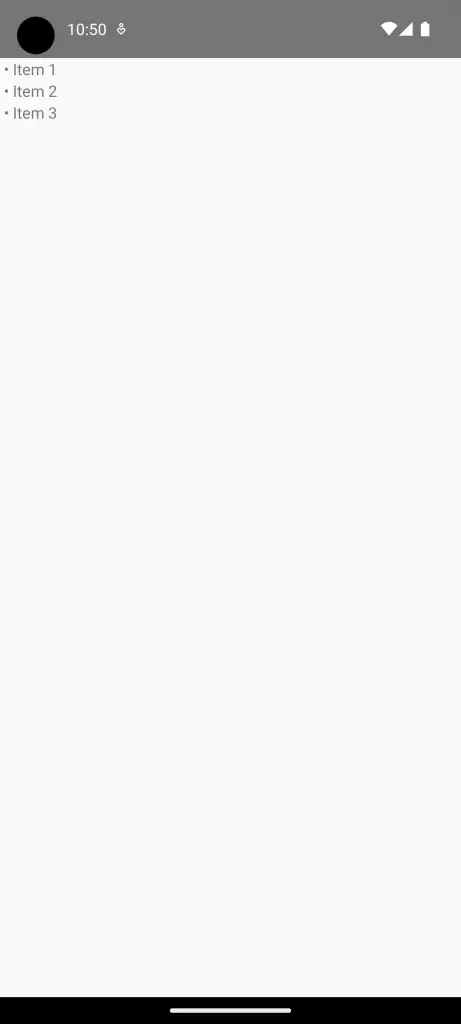
Method 2: Using Exact Unicode Characters
You can also use the exact Unicode representations like \u2022
or \u25CF
to create bullet points.
import React from 'react';
import {View, Text} from 'react-native';
const App = () => {
return (
<View>
<Text> {' \u25CF Item 1'}</Text>
<Text> {' \u25CF Item 2'}</Text>
<Text> {' \u25CF Item 3'}</Text>
</View>
);
};
export default App;
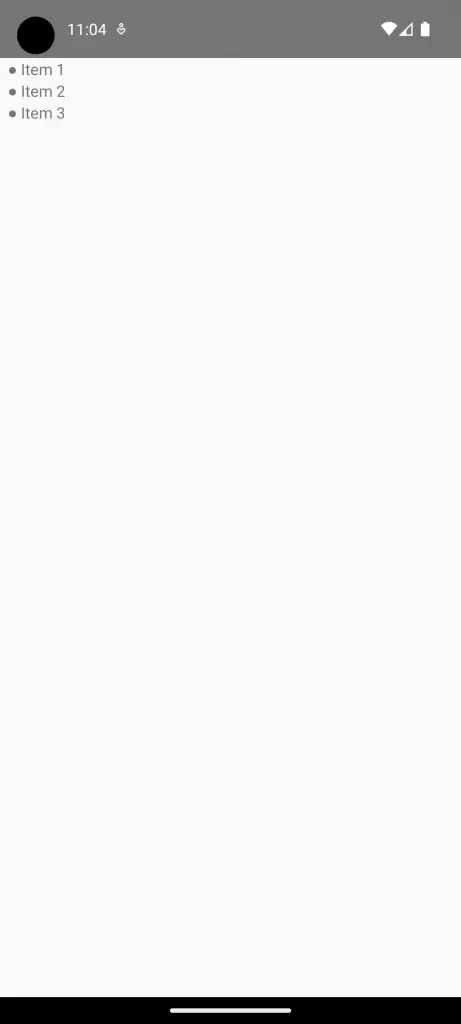
Method 3: Using Custom Components
For reusable lists, you might prefer a custom BulletPoint
component.
const BulletPoint = ({ item }) => {
return (
<Text style={styles.listItem}>
<Text style={styles.bulletPoint}>• </Text>
<Text>{item}</Text>
</Text>
);
};
const App = () => {
const items = ['Item 1', 'Item 2', 'Item 3'];
return (
<View style={styles.container}>
{items.map((item, index) => (
<BulletPoint key={index} item={item} />
))}
</View>
);
};
BulletPoint
is a custom component that takes an item
prop. The .map()
function creates a list of bullet points based on an array of items.
Creating bullet points in React Native can be a bit tricky due to the lack of built-in support. However, with these methods, you can easily add bullet points to your lists. You can use Unicode characters, their exact Unicode representations, or even create custom components for more complex lists.