How to Add Text Button in React Native
Text buttons are an essential part of any mobile application, offering a simple and effective way for users to interact with the app. React Native provides multiple ways to create buttons, and in this tutorial, we’ll explore two popular methods: using TouchableOpacity
and Pressable
.
Prerequisites
- Working React Native environment
- Basic knowledge of React Native and JavaScript
Use TouchableOpacity
for Text Button
The most straightforward way to create a text button is using TouchableOpacity
as a wrapper.
import React from 'react';
import {TouchableOpacity, Text, View, Alert} from 'react-native';
const App = () => {
return (
<View>
<TouchableOpacity onPress={() => Alert.alert('Button Pressed!')}>
<Text>Click Me to show the Alert!</Text>
</TouchableOpacity>
</View>
);
};
export default App;
TouchableOpacity
makes any view responsive to touch. The onPress
prop specifies what happens when the button is tapped.
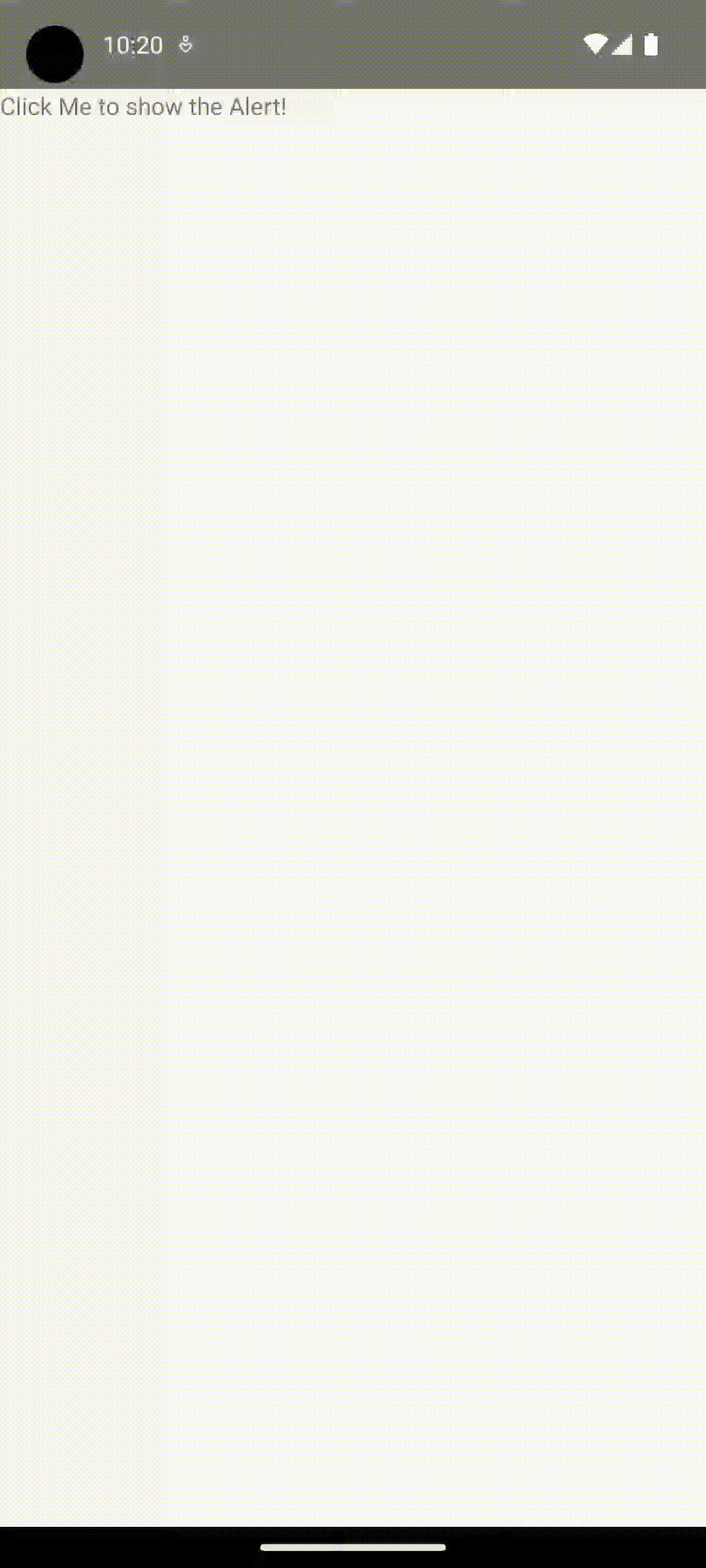
Use Pressable
for Text Button
Pressable
is another touch-responsive wrapper that provides more customization options. Here’s an example:
import React from 'react';
import {TouchableOpacity, Text, View, Alert} from 'react-native';
const App = () => {
return (
<View>
<TouchableOpacity onPress={() => Alert.alert('Button Pressed!')}>
<Text>Click Me to show the Alert!</Text>
</TouchableOpacity>
</View>
);
};
export default App;
Whether you choose TouchableOpacity
or Pressable
, creating text buttons in React Native is a breeze. Both methods offer easy customization and the ability to attach functions via the onPress
prop.
Depending on your specific needs, you can opt for the simplicity of TouchableOpacity
or the additional features provided by Pressable
.