How to Display PDF File from Project Assets in React Native
Sometimes, you may want to show the user a document that is loaded from the project assets. In this blog post, let’s check how to display a pdf file from your project assets in react native.
We are using react native pdf library to display pdf files. It also has a dependency with another library react native blob util. Hence, install the libraries using the following command.
npm install react-native-pdf react-native-blob-util --save
Then run pod install inside the ios folder. In Android, open android/app/build.gradle and add the following code inside the android tag.
packagingOptions {
pickFirst 'lib/x86/libc++_shared.so'
pickFirst 'lib/x86_64/libjsc.so'
pickFirst 'lib/arm64-v8a/libjsc.so'
pickFirst 'lib/arm64-v8a/libc++_shared.so'
pickFirst 'lib/x86_64/libc++_shared.so'
pickFirst 'lib/armeabi-v7a/libc++_shared.so'
}
That’s the installation part.
Add your pdf file to your project folder and import it into the app. Then you can display it using react native pdf.
See the following code.
import React from 'react';
import {StyleSheet, Dimensions, View, Platform} from 'react-native';
import Pdf from 'react-native-pdf';
import dummy from './dummy.pdf';
const App = () => {
return (
<View style={styles.container}>
<Pdf
source={dummy}
trustAllCerts={Platform.OS === 'ios'}
onLoadComplete={(numberOfPages, filePath) => {
console.log(`number of pages: ${numberOfPages}`);
}}
onPageChanged={(page, numberOfPages) => {
console.log(`current page: ${page}`);
}}
onError={error => {
console.log(error);
}}
onPressLink={uri => {
console.log(`Link presse: ${uri}`);
}}
style={styles.pdf}
/>
</View>
);
};
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'flex-start',
alignItems: 'center',
marginTop: 25,
},
pdf: {
flex: 1,
width: Dimensions.get('window').width,
height: Dimensions.get('window').height,
},
});
Following is the output in Android.
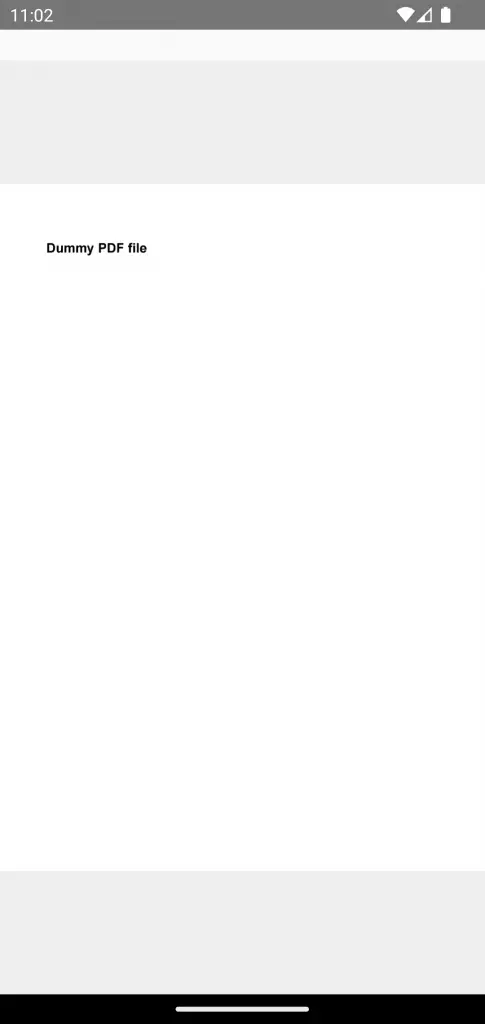
And the following is the output in iOS.
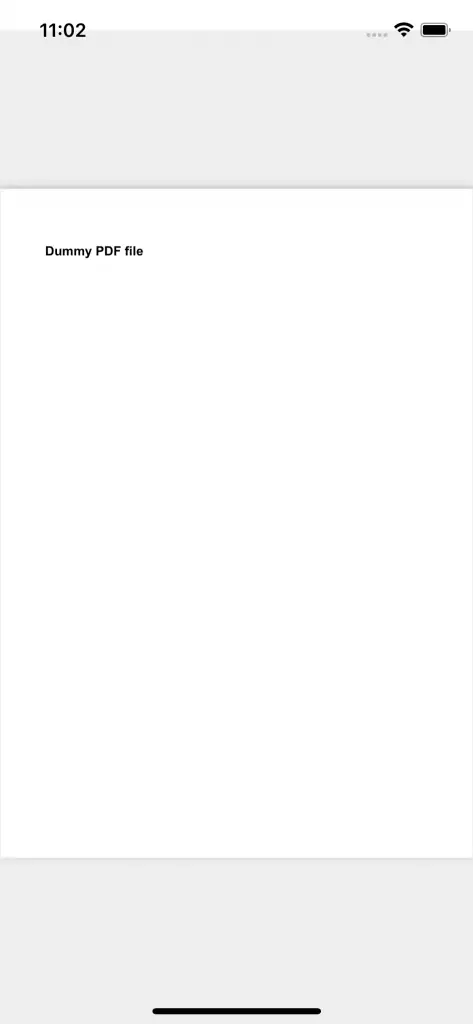
That’s how you open a pdf file from the project folder in react native.