How to Open PDF File in React Native with Default PDF Viewer
Opening and viewing PDF files is not a built-in feature in React Native. In this blog post, let’s learn how to open a PDF file in React Native using the device’s default PDF viewer.
In this tutorial, we want to show the PDF file which is downloaded from a URL. In order to download and obtain the downloaded path we use react native blob util library. Then we use the same library to open the PDF.
Install the library using the following command.
npm install --save react-native-blob-util
Then navigate to the ios folder and run the following command.
pod install
Then you can import the library as given below.
import ReactNativeBlobUtil from 'react-native-blob-util'
Following is the complete code where a PDF file is downloaded and then opened when the Button is pressed.
import {StyleSheet, Button, View, Platform} from 'react-native';
import React from 'react';
import ReactNativeBlobUtil from 'react-native-blob-util';
function App() {
let downloadPath =
Platform.OS === 'ios'
? ReactNativeBlobUtil.fs.dirs.DocumentDir + '/awesome.pdf'
: ReactNativeBlobUtil.fs.dirs.DownloadDir + '/awesome.pdf';
const android = ReactNativeBlobUtil.android;
const openPdf = () => {
ReactNativeBlobUtil.config({
fileCache: true,
appendExt: 'pdf',
addAndroidDownloads: {
title: 'awesome pdf',
description: 'An awesome PDF',
mime: 'application/pdf',
useDownloadManager: true,
mediaScannable: true,
notification: true,
path: downloadPath,
},
})
.fetch(
'GET',
'https://unec.edu.az/application/uploads/2014/12/pdf-sample.pdf',
)
.then(res => {
console.log('here');
const path = res.path();
if (Platform.OS === 'ios') {
ReactNativeBlobUtil.ios.previewDocument(path);
} else {
android.actionViewIntent(path, 'application/pdf');
}
});
};
return (
<View style={styles.container}>
<Button title="Click to Open PDF" onPress={() => openPdf()} />
</View>
);
}
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
The openPdf function fetches the contents of a remote PDF file using the fetch method from the ReactNativeBlobUtil library.
The config method is called first to configure the download options for the file, including its title, description, MIME type, and the directory where it should be saved.
If the app is running on iOS, the previewDocument method of the ios property of ReactNativeBlobUtil is called to open the PDF file.
If the app is running on Android, the actionViewIntent method of the android object is called to open the PDF file.
Following is the output.
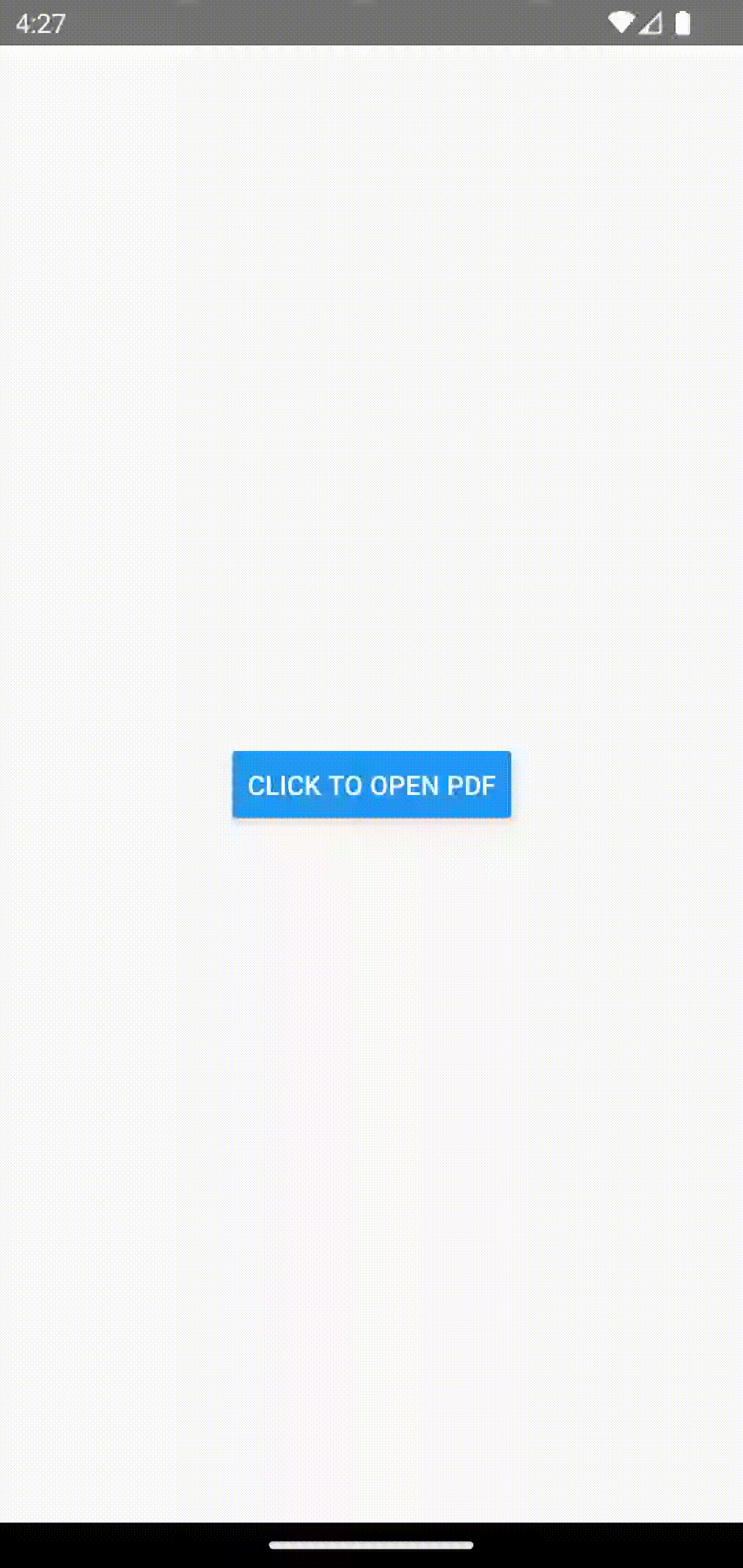
That’s how you open a pdf file with third-party app in react native.