How to Share PDF File in React Native
Sharing files can be a complex task, especially if you want to ensure that your users can share the files with different other apps. In this tutorial, let’s how to share pdf file in react native.
We use react native share library to share pdf files from our react native app. You can install the library using the following command.
npm i react-native-share --save
In this example, we are also using react native blob util library. It will help us to download a pdf file from a remote URL. You can install it using the following command.
npm install --save react-native-blob-util
After that browse to the ios folder and run the pod install command. That’s the installation part.
We download a pdf file from a remote URL to the given path and share the pdf using the path. See the following code.
import {StyleSheet, Button, View, Platform} from 'react-native';
import React, {useEffect} from 'react';
import ReactNativeBlobUtil from 'react-native-blob-util';
import Share from 'react-native-share';
function App() {
let downloadPath =
Platform.OS === 'ios'
? ReactNativeBlobUtil.fs.dirs.DocumentDir + '/awesome.pdf'
: ReactNativeBlobUtil.fs.dirs.DownloadDir + '/awesome.pdf';
const shareOptions = {
title: 'Share file',
failOnCancel: false,
url: `file://${downloadPath}`,
};
useEffect(() => {
openPdf();
}, []);
const openPdf = () => {
ReactNativeBlobUtil.config({
fileCache: true,
appendExt: 'pdf',
addAndroidDownloads: {
title: 'awesome pdf',
description: 'An awesome PDF',
mime: 'application/pdf',
useDownloadManager: true,
mediaScannable: true,
notification: true,
path: downloadPath,
},
})
.fetch(
'GET',
'https://unec.edu.az/application/uploads/2014/12/pdf-sample.pdf',
)
.then(res => {
const path = res.path();
console.log(path);
});
};
const sharePdf = async () => {
try {
const ShareResponse = await Share.open(shareOptions);
console.log('Result =>', ShareResponse);
} catch (error) {
console.log('Error =>', error);
}
};
return (
<View style={styles.container}>
<Button title="Click to Share PDF" onPress={() => sharePdf()} />
</View>
);
}
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
The code is a simple React Native application that demonstrates how to share a PDF file. It starts by importing the required modules and components from the ‘react-native’ library, as well as the ‘react-native-share’ and ‘react-native-blob-util’ libraries.
The downloadPath variable is used to store the file path for the PDF file. The shareOptions object is passed as an argument to the Share.open() function and contains information about the file being shared, such as the title and the file URL.
The useEffect hook is used to call the openPdf function when the component is mounted, which downloads the PDF file from a remote URL using the ReactNativeBlobUtil library.
The sharePdf function is called when the user clicks the button, which opens the device’s sharing dialog and passes the shareOptions object to it.
Following is the output in Android.
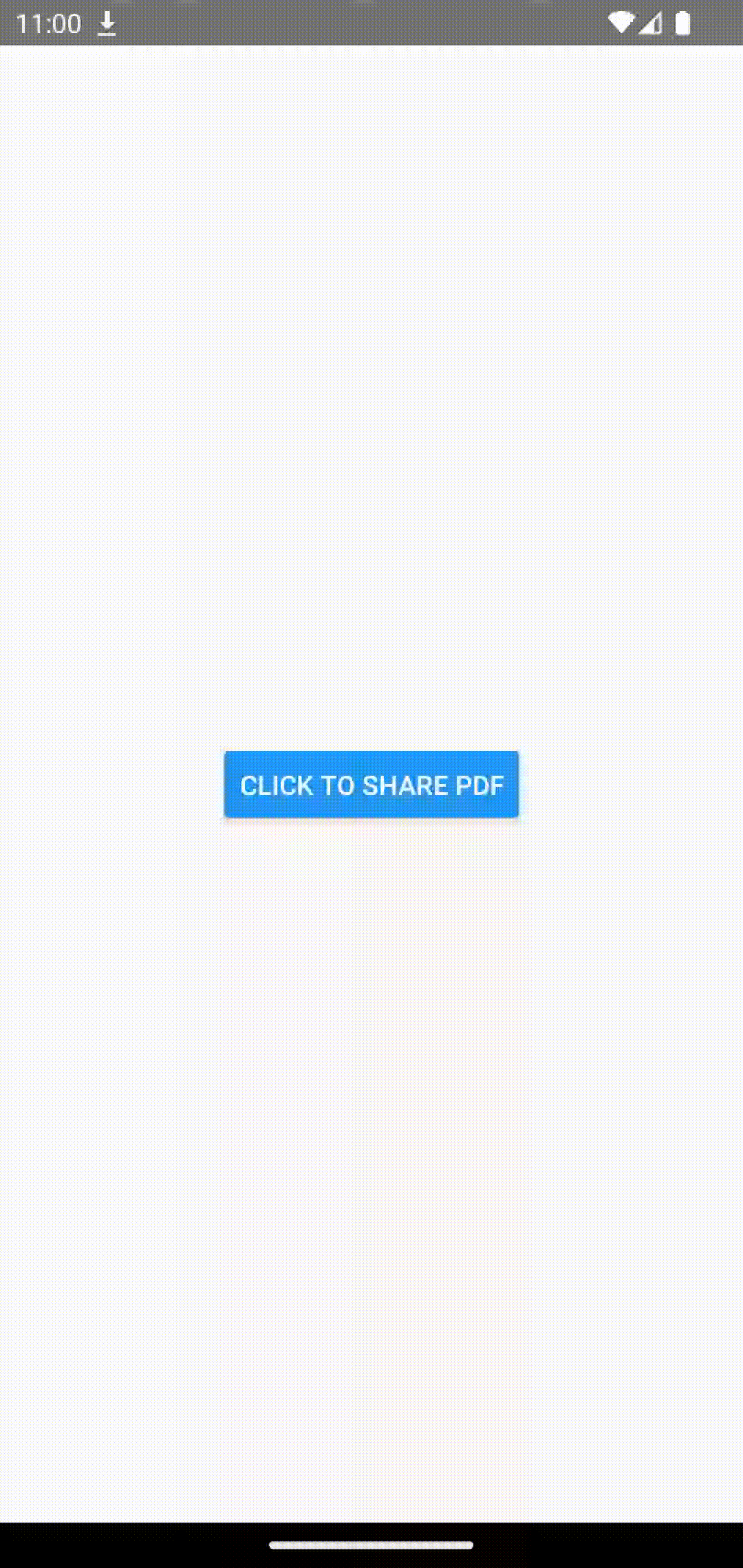
Following is the output in iOS.
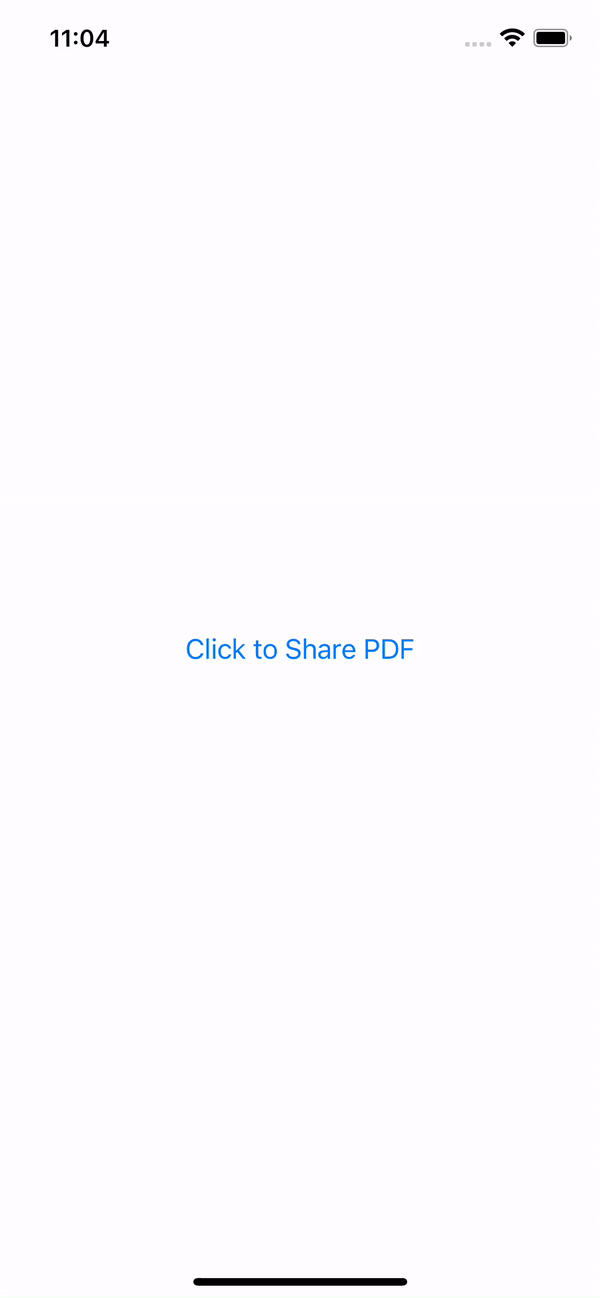
That’s how you share pdf files in react native.