How to Share Links in React Native
Sharing links is an important part of many mobile apps, allowing users to easily share content with friends and family. In this blog post, let’s learn how to share links from a react native app to other apps.
The React Native Share package is a popular library for adding sharing functionality to your react native app. This library provides a simple, cross-platform way to share text, URLs, or other data with other apps on a user’s device.
You can install react native share using any of the following commands.
npm i react-native-share --save
or
yarn add react-native-share
After that, run pod install command in the ios folder of your project.
Following is the code where you can share a URL in react native app.
import {StyleSheet, Button, View} from 'react-native';
import React from 'react';
import Share from 'react-native-share';
function App() {
const shareOptions = {
title: 'Awesome Tutorial',
url: 'https://codingwithrashid.com/how-to-share-pdf-file-in-react-native/',
message: 'Check this out!',
};
const shareURL = async () => {
try {
const ShareResponse = await Share.open(shareOptions);
console.log('Result =>', ShareResponse);
} catch (error) {
console.log('Error =>', error);
}
};
return (
<View style={styles.container}>
<Button title="Click to Share Link" onPress={() => shareURL()} />
</View>
);
}
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
You will get the following output in Android.
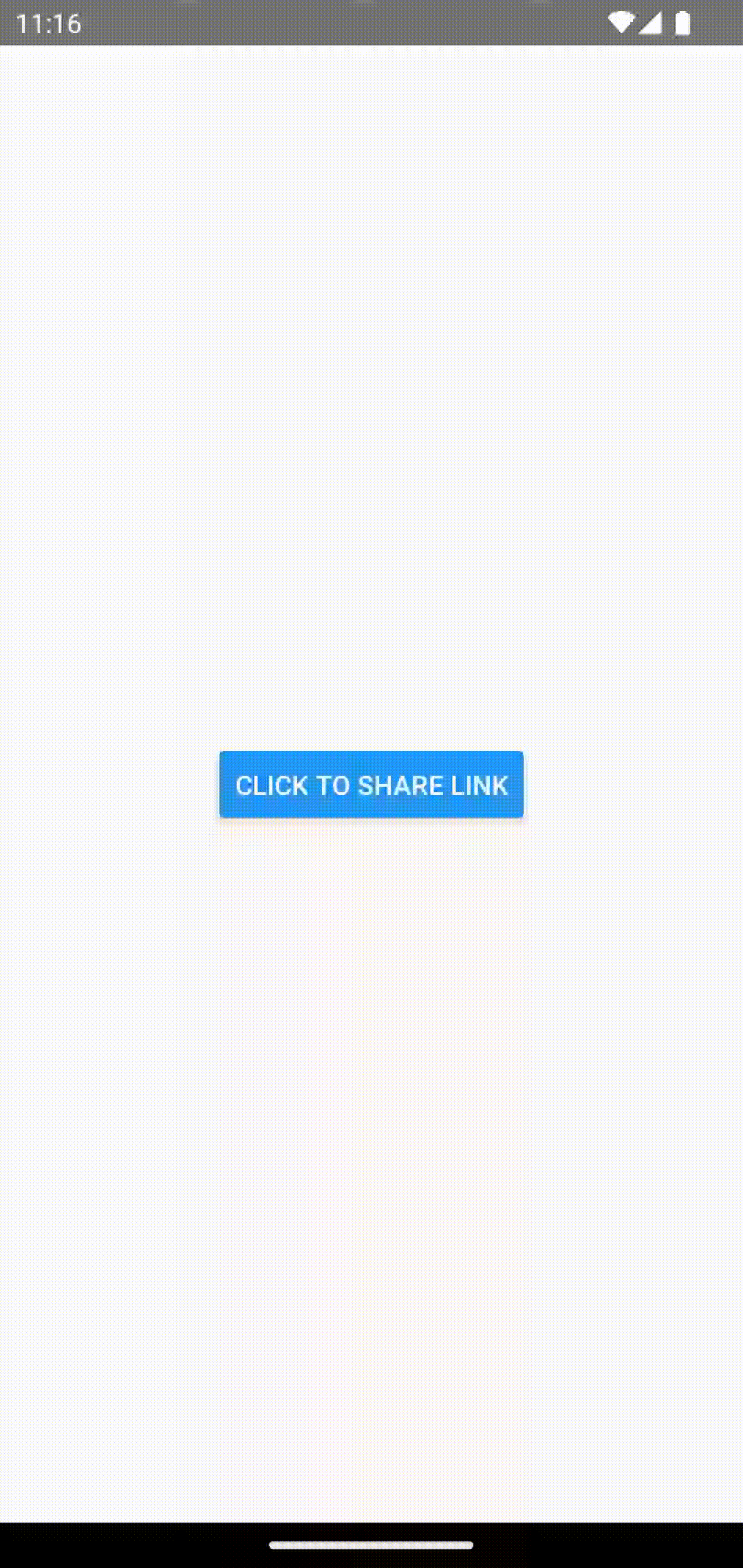
Following is the output in iOS.
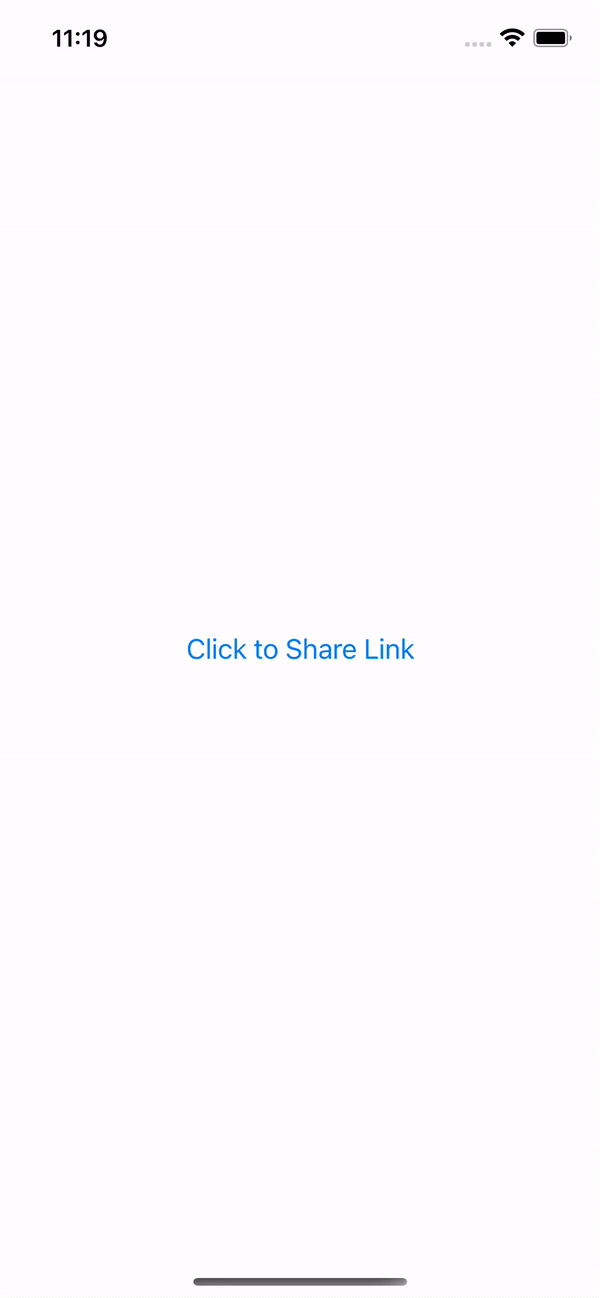
That’s how you share URL in react native