Text Alignment in React Native: A Detailed Guide
Text alignment is a crucial aspect of any mobile app’s user interface. It determines how text will be displayed within a layout, ensuring readability and visual appeal.
In this blog post, we’ll explore how to align text in React Native, using various methods and styles. We’ll include multiple examples to make sure you fully grasp the concept.
What is Text Alignment?
Text alignment refers to how text is positioned within a container. It can be aligned to the left, right, center, or justified. React Native provides easy-to-use properties for this purpose.
Basic Text Alignment Using textAlign
Left Alignment
By default, text in React Native is left-aligned. However, you can explicitly specify this using the textAlign
property.
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const App = () => {
return (
<View>
<Text style={styles.leftAlign}>This is left-aligned text.</Text>
</View>
);
};
const styles = StyleSheet.create({
leftAlign: {
textAlign: 'left',
},
});
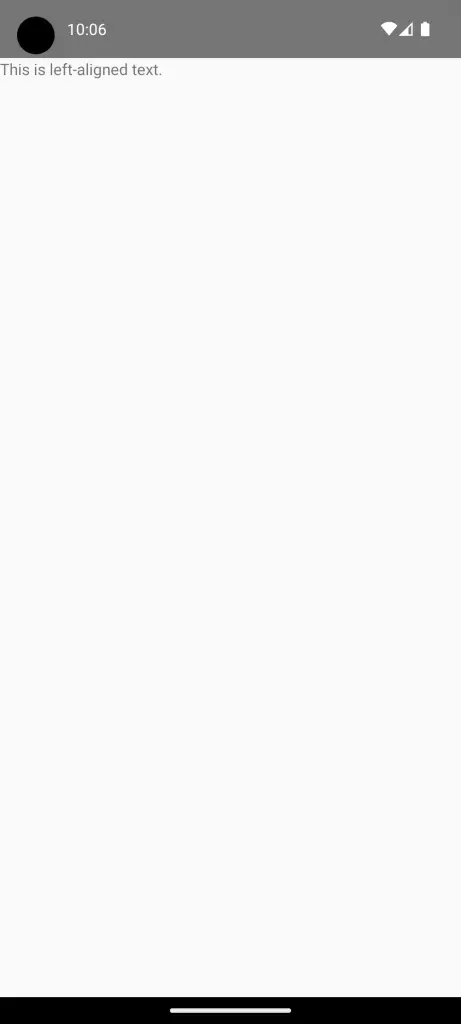
Center Alignment
Centering text is straightforward. Just set textAlign
to 'center'
.
<Text style={{ textAlign: 'center' }}>This is center-aligned text.</Text>
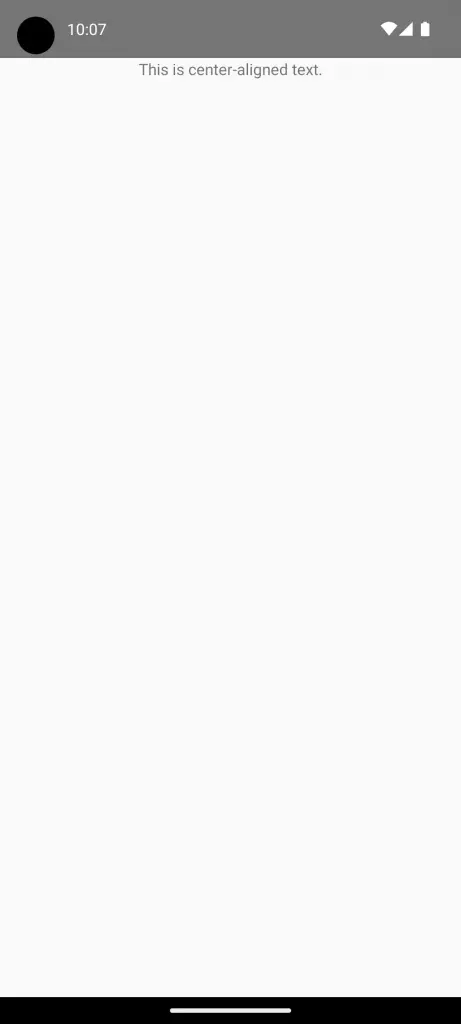
Right Alignment
To align text to the right, set textAlign
to 'right'
.
<Text style={{ textAlign: 'right' }}>This is right-aligned text.</Text>
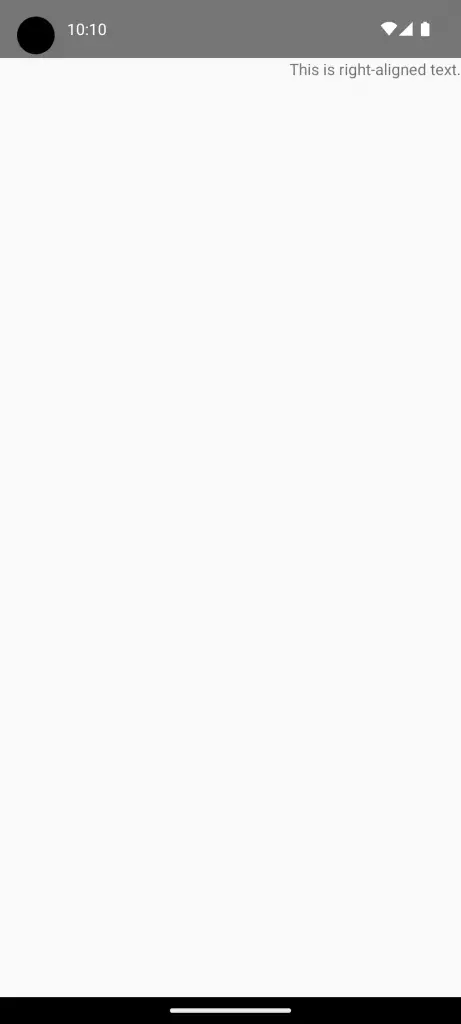
Advanced Text Alignment Techniques
Use Flexbox
You can use Flexbox to align a block of text within a container.
<View style={{ alignItems: 'center' }}>
<Text>This text is centered using Flexbox.</Text>
</View>
Use Padding and Margins
You can also align text by manipulating padding and margins.
<Text style={{ paddingLeft: 50 }}>This text is shifted to the right.</Text>
Mastering text alignment in React Native can significantly improve your app’s user experience. With the various methods and properties available, aligning text becomes a simple task. Keep practicing with different examples to perfect your skills.