A Comprehensive Guide to Text Decoration in React Native
When developing a React Native application, text styling is crucial for creating an appealing UI/UX. One often overlooked aspect of text styling is text decoration.
Whether you’re creating hyperlinks, emphasizing text, or adding strikethroughs, understanding text decoration can make a difference. In this blog post, we’ll explore various ways to decorate text in React Native.
Prerequisites
- A working React Native environment
- Basic understanding of React Native and JavaScript
Text Decoration Line
One of the simplest properties for decorating text is textDecorationLine
. It supports underline, line-through, and combinations of both. Here’s how to use it:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const App = () => (
<View style={styles.container}>
<Text style={styles.underlineText}>This is underlined text.</Text>
<Text style={styles.lineThroughText}>This is line-through text.</Text>
</View>
);
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
},
underlineText: {
textDecorationLine: 'underline',
},
lineThroughText: {
textDecorationLine: 'line-through',
},
});
export default App;
textDecorationLine: 'underline'
adds an underline to the text.textDecorationLine: 'line-through'
adds a line through the text.
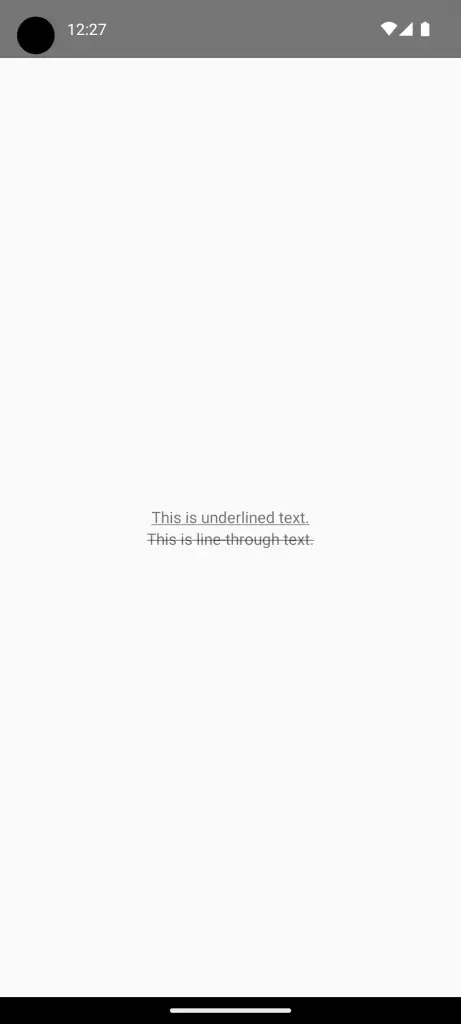
Text Decoration Color
You can also specify the color of the text decoration line using the textDecorationColor
property. This property works only in iOS and not in Android devices.
const styles = StyleSheet.create({
underlineText: {
textDecorationLine: 'underline',
textDecorationColor: 'red',
},
});
Text Decoration Style
For more variety, textDecorationStyle
can be used to set the style of the text decoration line. It accepts values like solid
, double
, dotted
, and dashed
. Like textDecorationColor
style property this works only on iOS.
const styles = StyleSheet.create({
dottedUnderlineText: {
textDecorationLine: 'underline',
textDecorationStyle: 'dotted',
},
});
Combine Multiple Text Decorations
You can combine multiple decorations to achieve complex styles.
const styles = StyleSheet.create({
combinedText: {
textDecorationLine: 'underline line-through',
textDecorationStyle: 'dotted',
textDecorationColor: 'green',
},
});
Text decoration in React Native provides a simple yet effective way to style your text elements. Using properties like textDecorationLine
, textDecorationColor
, and textDecorationStyle
, you can create various text styles to fit your application’s design needs.