How to Display PDF from Base64 in React Native
It’s common for applications to need to display PDF files to their users. This can be especially important for applications that rely on displaying invoices, contracts, or other important documents.
In this tutorial, let’s learn how to display PDF from base64 in react native using react native pdf library.
React Native Pdf also need another library react native blob util. Install both libraries using the command given below.
npm install react-native-pdf react-native-blob-util --save
Also, don’t forget to run the pod install command inside the ios folder.
On Android, open android/app/build.gradle and add the following inside the android {} tag.
packagingOptions {
pickFirst 'lib/x86/libc++_shared.so'
pickFirst 'lib/x86_64/libjsc.so'
pickFirst 'lib/arm64-v8a/libjsc.so'
pickFirst 'lib/arm64-v8a/libc++_shared.so'
pickFirst 'lib/x86_64/libc++_shared.so'
pickFirst 'lib/armeabi-v7a/libc++_shared.so'
}
That’s the installation part.
You can pass the base64 as the source URI and the pdf will be shown. See the following code.
import React from 'react';
import {StyleSheet, Dimensions, View, Platform} from 'react-native';
import Pdf from 'react-native-pdf';
const source = {
uri: 'data:application/pdf;base64,JVBERi0xLjQ........Cg==',
};
const App = () => {
return (
<View style={styles.container}>
<Pdf
source={source}
trustAllCerts={Platform.OS === 'ios'}
onLoadComplete={(numberOfPages, filePath) => {
console.log(`number of pages: ${numberOfPages}`);
}}
onPageChanged={(page, numberOfPages) => {
console.log(`current page: ${page}`);
}}
onError={error => {
console.log(error);
}}
onPressLink={uri => {
console.log(`Link presse: ${uri}`);
}}
style={styles.pdf}
/>
</View>
);
};
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'flex-start',
alignItems: 'center',
marginTop: 25,
},
pdf: {
flex: 1,
width: Dimensions.get('window').width,
height: Dimensions.get('window').height,
},
});
For testing, you can use the base64 data given here.
Following is the output in Android.
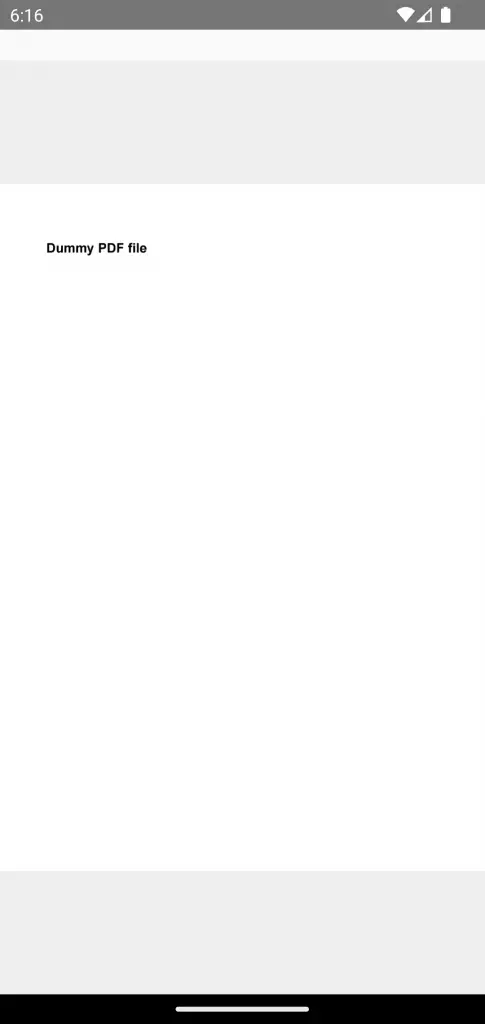
And following is the output in iOS.
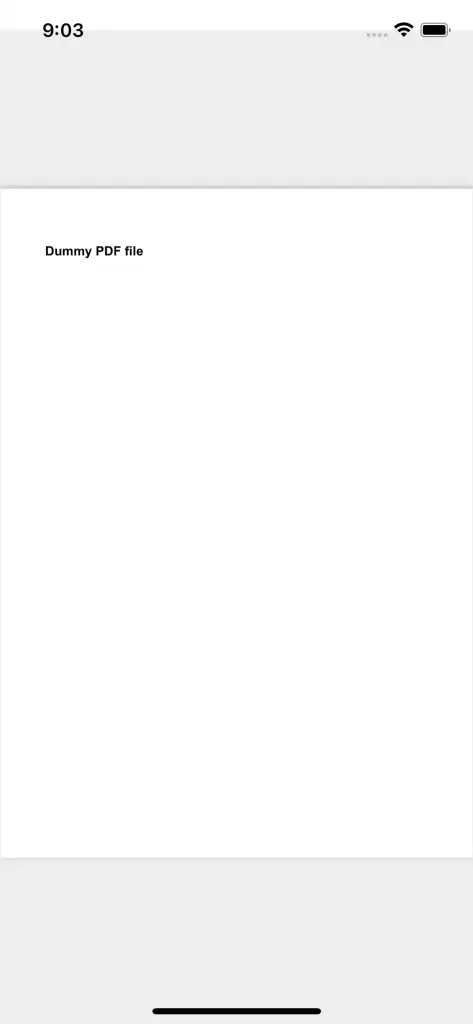
That’s how you show PDF files from base64 in react native.