How to Open SMS App in React Native
An SMS app allows users to send and receive text messages on their mobile devices. Sometimes, we want to prompt users to open the SMS app. In this tutorial, let’s learn how to open the SMS app in react native.
The Linking API in React Native offers a solution for developers to manage both incoming and outgoing links within their app. With this tool, you can effortlessly open links from external sources, guide users to other apps or websites, and initiate emails or text messages straight from your app.
You can use the openURL method of Linking to open the SMS app with the phone number you want to send the message.
Linking.openURL('sms:+123456789')
If you just want to launch the SMS app then see the following snippet.
Linking.openURL('sms:')
Following is the complete example where I launch the SMS app when the button is pressed.
import {StyleSheet, Button, View, Linking} from 'react-native';
import React from 'react';
function App() {
return (
<View style={styles.container}>
<Button
title="Click to Open SMS App"
onPress={() => Linking.openURL('sms:+91234567')}
/>
</View>
);
}
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
You will get the following output in Android.
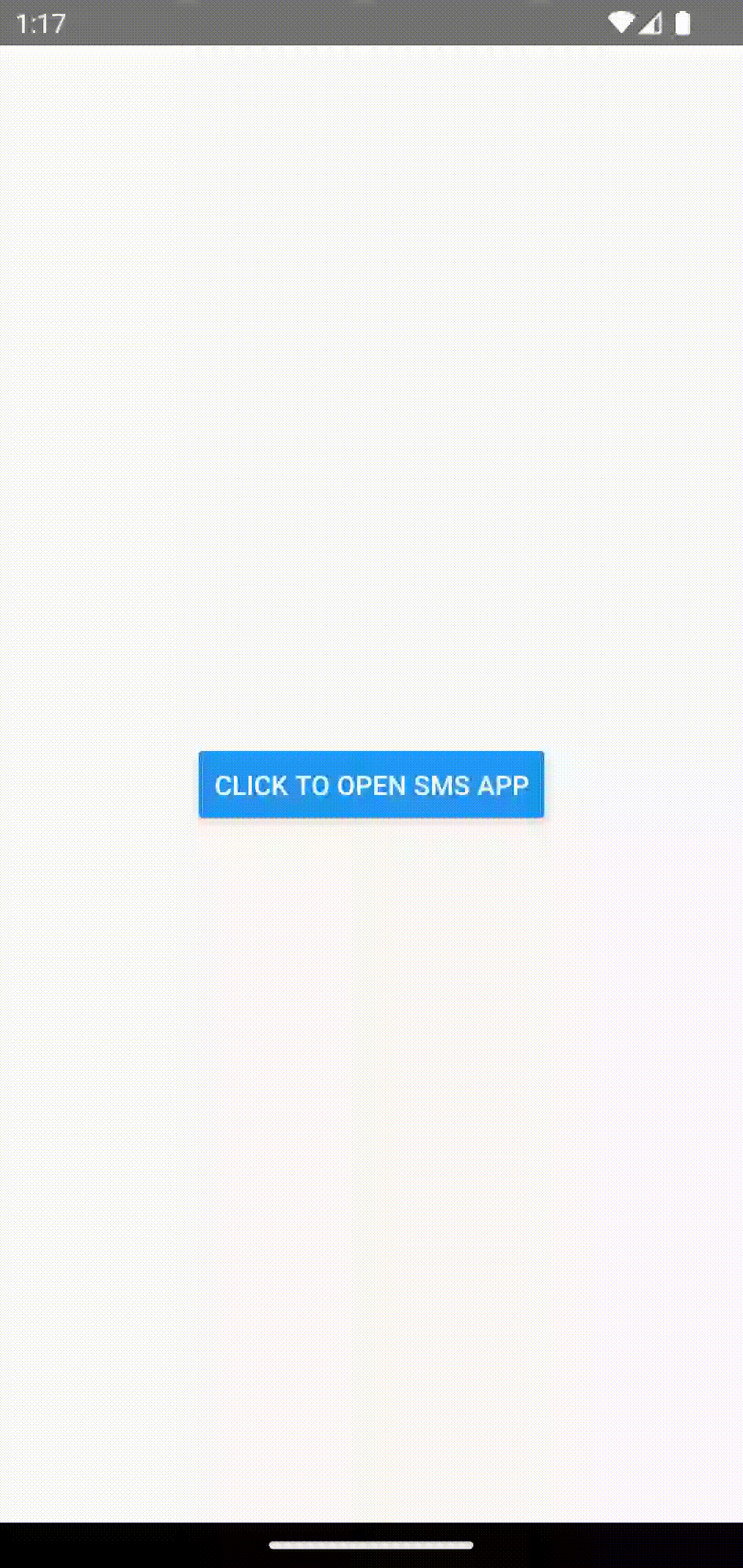
Following is the output in iOS.
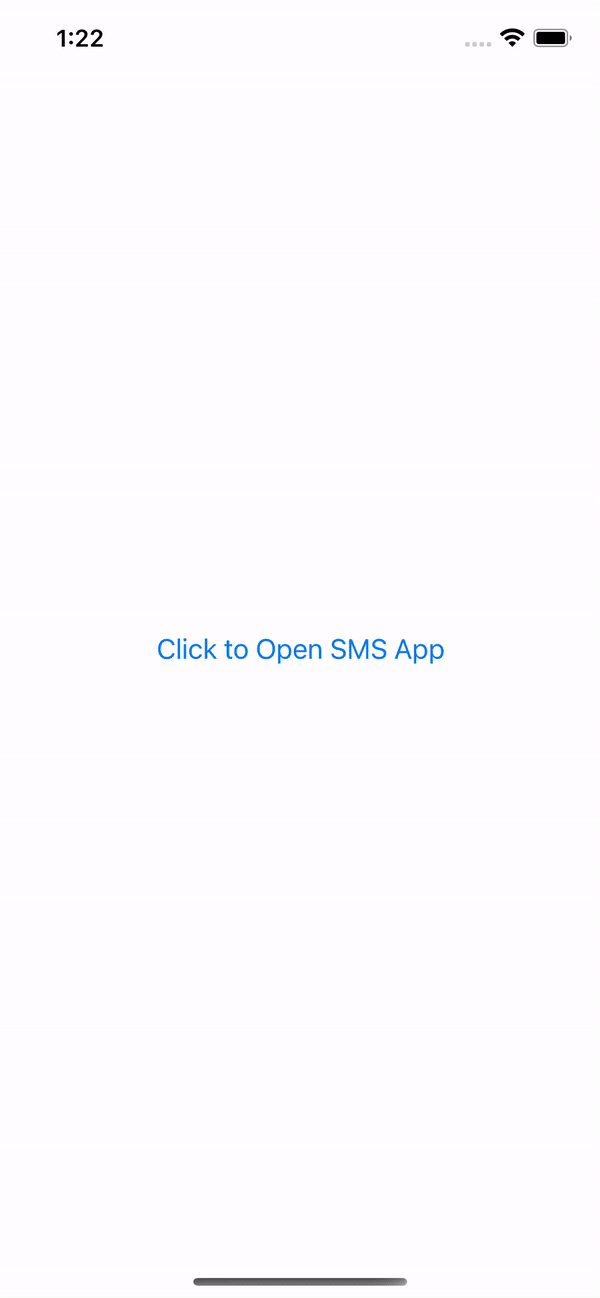
That’s how you open the SMS app in react native using Linking API.