How to Add Picker Wheel in iOS SwiftUI
The Picker Wheel is a popular UI element in SwiftUI that allows users to scroll through a list of options and make a selection. It’s especially useful in mobile apps where screen real estate is limited. In this blog post, we’ll explore how to implement and customize a Picker Wheel in SwiftUI.
What is a Picker Wheel?
A Picker Wheel is a rotating selection tool that lets users scroll through a list of options. It’s often used in date pickers, but it can be customized for any list of selectable items. In SwiftUI, creating a Picker Wheel is straightforward and highly customizable.
Basic Implementation of Picker Wheel
Here’s the complete code for implementing a Picker Wheel with dynamic data:
import SwiftUI
struct ContentView: View {
@State private var selectedIndex = 0
let options = ["Option 1", "Option 2", "Option 3"]
var body: some View {
Picker("Choose an option", selection: $selectedIndex) {
ForEach(0..<options.count, id: \.self) {
Text(options[$0]).tag($0)
}
}
.pickerStyle(.wheel)
}
}
As you see, the picker style is set to wheel.
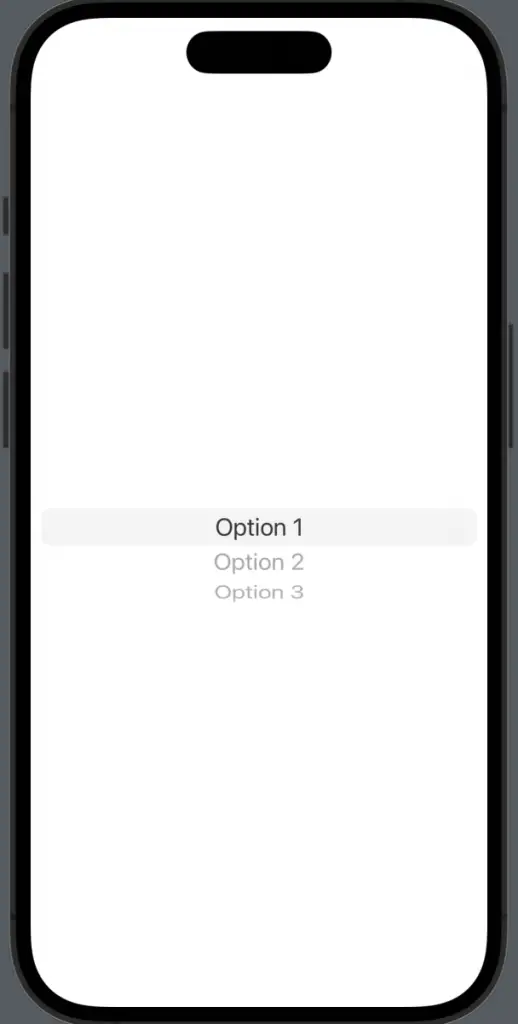
Implementing a Picker Wheel with dynamic data in SwiftUI is straightforward. By using a ForEach
loop, you can easily populate the Picker with an array of options. This makes your app more flexible and easier to manage.