How to Align VStack Items to Top in iOS SwiftUI
Creating a visually stunning and functional UI is an important part of app development. SwiftUI, Apple’s UI toolkit, provides various layout structures to facilitate this task. VStack is one of these structures that arranges views vertically.
However, a common question arises – How to align VStack items to the top in SwiftUI?. In this post, we will explore how to achieve this.
VStack in SwiftUI
Before we delve into aligning VStack items, let’s recap what VStack is. VStack, short for Vertical Stack, is a layout in SwiftUI that stacks its child views vertically. It gives developers control over the distribution and alignment of these views along the Y-axis.
VStack Alignment: The Basics
By default, VStack places its child views in the center of the available space. It also aligns them along a horizontal axis, which can be leading, center, or trailing. However, what if you want the child views to align towards the top?
Align VStack Items to the Top in SwiftUI
Aligning VStack items to the top is not as straightforward as setting an alignment parameter. It involves wrapping the VStack in a frame that has a maximum height and setting its alignment to .topLeading
. Here’s an example:
struct ContentView: View {
var body: some View {
VStack() {
Text("Hello")
Text("World")
}.frame(maxWidth: .infinity, maxHeight: .infinity, alignment: .topLeading)
}
}
In this code snippet, the VStack containing two text views – “Hello” and “World” – is wrapped in a frame with maxWidth: .infinity
and maxHeight: .infinity
. The alignment is set to .topLeading
. As a result, the VStack’s child views align to the top of the available space.
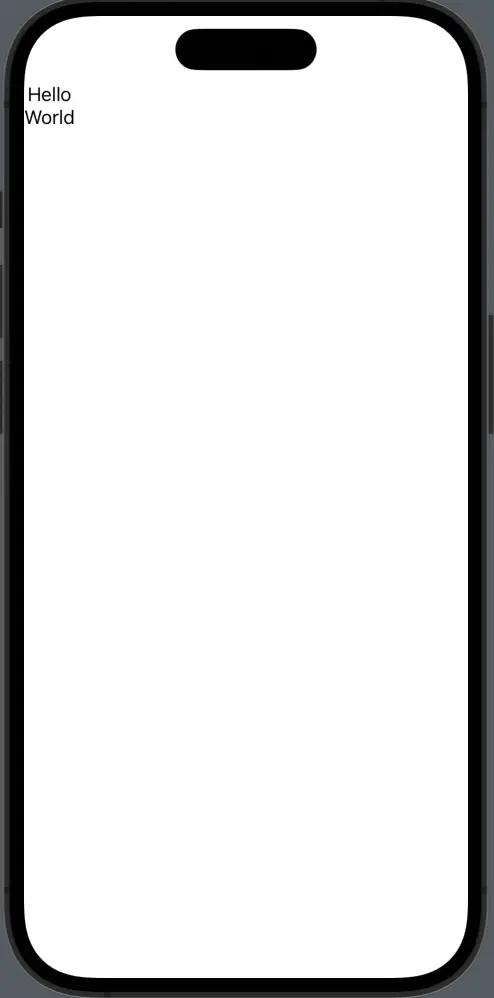
Here’s what happens in the above code:
maxWidth: .infinity
andmaxHeight: .infinity
make the frame occupy all the available space.- The
alignment: .topLeading
parameter aligns the VStack’s content at the top left corner (for left-to-right languages). - Thus, the VStack views – “Hello” and “World” – are placed at the top, rather than the center.
Understanding how to align VStack items to the top in SwiftUI can be an excellent tool in your SwiftUI toolkit. It allows you to manipulate the layout further and create visually engaging and functionally effective user interfaces.