How to Hide Sheet Drag Indicator in iOS SwiftUI
In SwiftUI, sheets are a common way to present secondary views or modals. When you have multiple detents for your sheet, a drag indicator appears automatically to signify that the sheet can be dragged to different heights.
However, there might be scenarios where you want to hide this drag indicator. In this blog post, we’ll explore how to hide the sheet drag indicator in SwiftUI using the .presentationDragIndicator
modifier.
Show Drag Indicator
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Modal") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm a modal!")
Button("Dismiss") {
showModal = false
}
}.presentationDetents([.medium, .large])
}
}
}
Code Explanation
.presentationDetents([.medium, .large])
: We specify two detents,.medium
and.large
, which means the drag indicator will automatically appear.
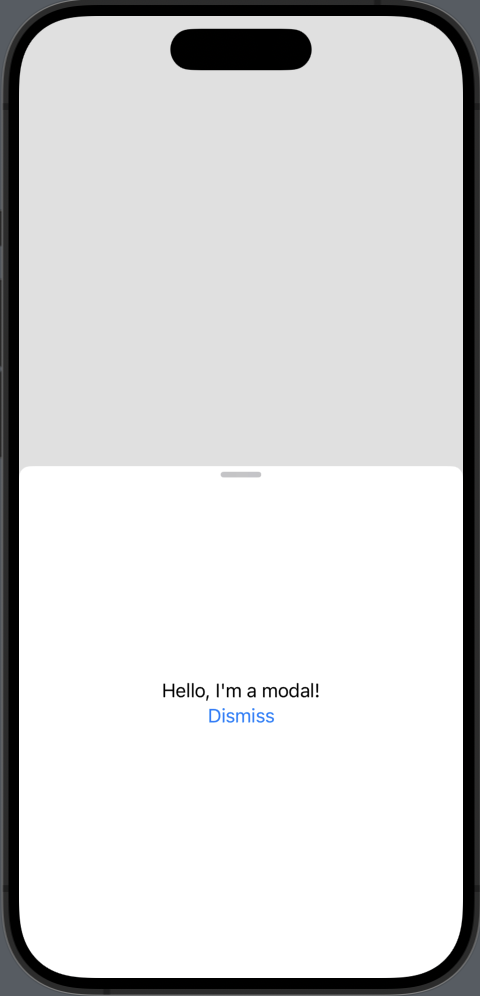
Hide Drag Indicator
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Modal") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm a modal!")
Button("Dismiss") {
showModal = false
}
}.presentationDetents([.medium, .large])
.presentationDragIndicator(.hidden)
}
}
}
Code Explanation
.presentationDragIndicator(.hidden)
: We use this modifier to hide the drag indicator, even when multiple detents are specified.
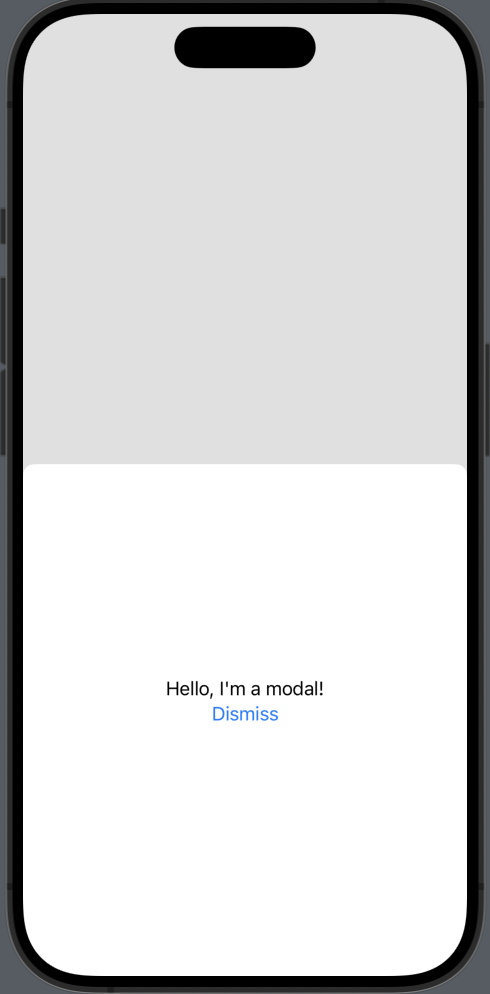
Why Hide the Drag Indicator?
Here are some reasons you might want to hide the drag indicator:
- Design Aesthetics: You may want a cleaner look for your sheet without the drag indicator.
- User Experience: If the sheet’s height doesn’t need to be adjusted, hiding the indicator can reduce user confusion.
- Custom Behavior: You might have implemented custom drag behavior and don’t need the default indicator.
Conclusion
Hiding the sheet drag indicator in SwiftUI is a straightforward task, thanks to the .presentationDragIndicator
modifier. Whether you want to improve the aesthetics, enhance user experience, or implement custom behavior, this modifier provides a simple solution.