How to Set LazyHGrid Background Color in iOS SwiftUI
In the realm of SwiftUI, LazyHGrid is a powerful tool for creating horizontal grid layouts. In this blog post will focus on a specific aspect of working with LazyHGrid: how to set a background color. This seemingly simple task can enhance your app’s visual appeal significantly.
LazyHGrid Overview
Before we delve into setting the background color, let’s briefly discuss what LazyHGrid is. LazyHGrid is used to present data in a horizontally scrolling grid layout. This lazy-loading capability ensures that the app only renders views currently visible on screen, optimizing performance.
Set the Background Color for LazyHGrid
A common requirement while designing an app is to set a background color for a grid. This helps to visually separate the grid from the rest of the content on the screen and can enhance user experience. Let’s look at how we can achieve this with SwiftUI’s LazyHGrid.
Here is an example of a LazyHGrid displaying numbers in two rows with a blue background:
struct ContentView: View {
let gridItems = [GridItem(.flexible()), GridItem(.flexible())]
var body: some View {
ScrollView(.horizontal) {
LazyHGrid(rows: gridItems, spacing: 20) {
ForEach(1...50, id: \.self) { index in
Text("\(index)")
.frame(width: 100, height: 100)
.background(Color.yellow)
.cornerRadius(10)
}
}
.padding()
.background(Color.blue)
}
}
}
In this example, the numbers 1 through 50 are displayed in yellow boxes on a blue background. The LazyHGrid scrolls horizontally.
This code block creates a LazyHGrid with a blue background color. Let’s break it down:
ScrollView(.horizontal)
: This creates a scroll view that scrolls horizontally.LazyHGrid(rows: gridItems, spacing: 20)
: This creates a LazyHGrid with two rows and 20 points of spacing.ForEach(1...50, id: \.self)
: This creates 50 child views for the grid.Text("\(index)")
: This displays the number for each child view..frame(width: 100, height: 100)
: This sets each child view’s size to 100×100 points..background(Color.yellow)
: This sets the background color of each child view to yellow..cornerRadius(10)
: This rounds the corners of each child view..padding()
: This adds padding around the LazyHGrid..background(Color.blue)
: This sets the background color of the LazyHGrid to blue.
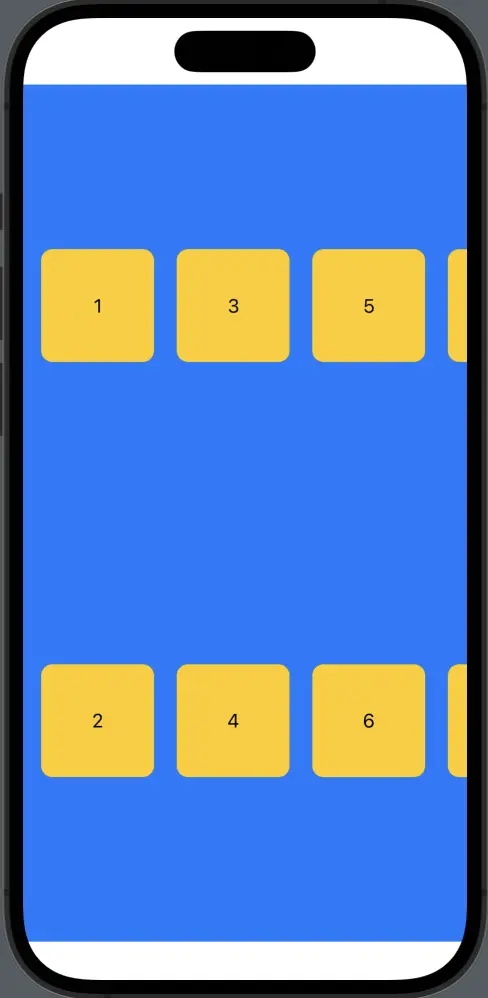
Setting the background color for a LazyHGrid in SwiftUI is simple yet effective for improving your app’s aesthetics. Using this method, you can create a visually engaging user interface that separates your grid content from other elements on the screen.